Express and Docker: Containerizing Your Web Application
Containerizing a web application is a powerful strategy for improving deployment and scalability. By combining Express, a minimal and flexible Node.js web application framework, with Docker, a containerization platform, developers can streamline their deployment processes and ensure consistent environments across different stages. This article explores how to use Express and Docker together, providing practical examples to guide you through containerizing your web application.
Understanding Express and Docker
Express is a popular framework for building web applications in Node.js, known for its simplicity and robust features. Docker is a containerization platform that enables developers to package applications and their dependencies into containers, which can be deployed across various environments seamlessly. Combining these tools allows for a more efficient development workflow and consistent deployment.
Setting Up an Express Application
Before containerizing your Express application, you need a basic Express setup. Here’s a quick example of a simple Express application.
Example: Basic Express Application
- Initialize a new Node.js project:
```bash mkdir my-express-app cd my-express-app npm init -y ```
- Install Express:
```bash npm install express ```
- Create a basic Express server in `index.js`:
```javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`App listening at http://localhost:${port}`); }); ```
Creating a Dockerfile for Your Express Application
Docker requires a `Dockerfile` to build an image of your application. This file contains instructions for Docker to create a containerized version of your app.
Example: Dockerfile
- Create a `Dockerfile` in your project root:
```dockerfile # Use the official Node.js image. # https://hub.docker.com/_/node FROM node:14 # Set the working directory in the container. WORKDIR /usr/src/app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies. RUN npm install # Copy the rest of the application code. COPY . . # Expose port 3000 to the outside world. EXPOSE 3000 # Define the command to run your app. CMD [ "node", "index.js" ] ```
Building and Running the Docker Container
With your `Dockerfile` ready, you can now build and run your Docker container.
- Build the Docker image:
```bash docker build -t my-express-app . ```
- Run the Docker container:
```bash docker run -p 3000:3000 my-express-app ```
Your Express application should now be accessible at `http://localhost:3000`.
Managing Environment Variables with Docker
Docker allows you to manage environment variables easily, which is useful for configuring different environments (development, testing, production).
Example: Docker Compose for Environment Variables
- Create a `docker-compose.yml` file:
```yaml version: '3' services: app: build: . ports: - "3000:3000" environment: NODE_ENV: production PORT: 3000 ```
- Run Docker Compose:
```bash docker-compose up ```
Debugging and Logging in Docker
Debugging and logging are critical aspects of managing containerized applications.
Example: Viewing Logs
To view the logs from your running container:
```bash docker logs <container_id> ```
You can also use Docker Compose to view logs from all services:
```bash docker-compose logs ```
Conclusion
Combining Express with Docker streamlines the deployment and scaling of web applications. By following the steps outlined above, you can efficiently containerize your Express application and ensure a consistent deployment environment. Leveraging Docker’s capabilities will help you maintain robust and scalable applications across various environments.
Further Reading
Table of Contents
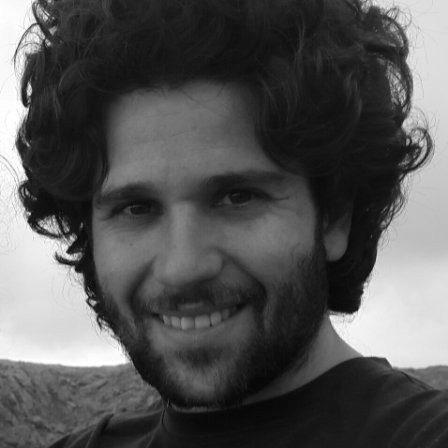
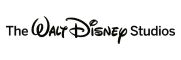