Express and Firebase: Building Real-Time Applications
Real-time applications have become essential in modern web development, allowing users to interact with systems and receive updates instantly. Express.js, a minimal and flexible Node.js web application framework, paired with Firebase, a real-time NoSQL database and backend-as-a-service, creates a powerful combination for developing dynamic, real-time applications. This blog explores how to use Express and Firebase together and provides practical examples to get you started.
Understanding Real-Time Applications
Real-time applications (RTAs) allow users to get instant updates without needing to refresh the page. This is achieved through technologies like WebSockets, Firebase Realtime Database, and Firestore, which enable data synchronization across clients in real-time. Express.js serves as the backend framework that handles routing, middleware, and business logic, while Firebase manages the real-time data synchronization and user authentication.
Setting Up Express with Firebase
Before diving into real-time features, it’s important to set up your Express server and connect it to Firebase. The following steps outline how to integrate Firebase into an Express application.
1. Initializing an Express Application
Start by creating a basic Express application. If you haven’t installed Express yet, you can do so using npm:
```bash npm install express ```
Create an `index.js` file with the following code:
```javascript const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, World!'); }); const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on port ${port}`); }); ```
2. Integrating Firebase
To integrate Firebase, you’ll need to install the Firebase SDK:
```bash npm install firebase ```
Next, initialize Firebase in your Express application:
```javascript const firebase = require('firebase/app'); require('firebase/database'); require('firebase/auth'); const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", databaseURL: "YOUR_DATABASE_URL", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; firebase.initializeApp(firebaseConfig); ```
Real-Time Data Synchronization with Firebase
Firebase Realtime Database allows you to store and sync data between users in real-time. The following example demonstrates how to set up a basic real-time data listener using Firebase and Express.
Example: Real-Time Chat Application
Let’s create a simple chat application where users can send and receive messages in real-time.
1. Setting Up Firebase Realtime Database
In your Firebase console, create a new database and set the rules to allow read/write access (for testing purposes):
```json { "rules": { ".read": true, ".write": true } } ```
2. Creating an API Endpoint to Send Messages
In your Express app, create an endpoint to post messages to Firebase:
```javascript app.post('/send-message', (req, res) => { const message = req.body.message; const timestamp = Date.now(); firebase.database().ref('messages/').push({ message: message, timestamp: timestamp }); res.status(200).send('Message sent'); }); ```
3. Listening for Real-Time Updates
On the client-side, you can listen for new messages in real-time:
```javascript firebase.database().ref('messages/').on('child_added', (snapshot) => { const messageData = snapshot.val(); console.log('New message:', messageData.message); }); ```
Handling User Authentication with Firebase
Firebase Authentication provides a simple way to manage user accounts and authenticate users in your Express app. Here’s how you can set up user authentication with Firebase.
Example: User Registration and Login
1. Setting Up Firebase Authentication
In your Firebase console, enable the desired authentication methods (e.g., Email/Password).
2. User Registration Endpoint
Create an endpoint in Express to register new users:
```javascript app.post('/register', (req, res) => { const email = req.body.email; const password = req.body.password; firebase.auth().createUserWithEmailAndPassword(email, password) .then((userCredential) => { res.status(200).send('User registered'); }) .catch((error) => { res.status(400).send(error.message); }); }); ```
3. User Login Endpoint
Similarly, create an endpoint for user login:
```javascript app.post('/login', (req, res) => { const email = req.body.email; const password = req.body.password; firebase.auth().signInWithEmailAndPassword(email, password) .then((userCredential) => { res.status(200).send('User logged in'); }) .catch((error) => { res.status(400).send(error.message); }); }); ```
Conclusion
Combining Express and Firebase enables developers to build powerful real-time applications with dynamic features and seamless user experiences. Whether you’re working on a chat application, live updates, or collaborative tools, Express and Firebase provide the necessary tools to bring your ideas to life. By leveraging Firebase’s real-time database and authentication alongside Express’s robust backend capabilities, you can create scalable, real-time applications that meet modern web development needs.
Further Reading:
Table of Contents
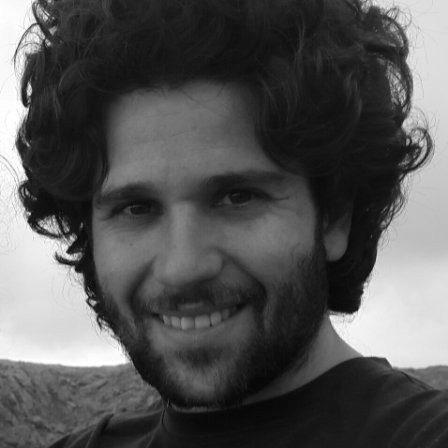
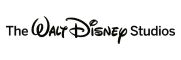