Express and Authentication: Implementing OAuth 2.0
Authentication is a critical aspect of web applications, ensuring that users are who they claim to be. OAuth 2.0 is a widely adopted authentication protocol that enables secure authorization in a simple and standardized manner. Combined with Express.js, a popular Node.js web application framework, implementing OAuth 2.0 becomes straightforward.
This article explores how to set up OAuth 2.0 in an Express.js application, guiding you through the process of integrating with popular OAuth 2.0 providers such as Google, GitHub, and Facebook.
Understanding OAuth 2.0
OAuth 2.0 is an authorization framework that allows third-party services to exchange tokens securely on behalf of a user. It’s commonly used for enabling Single Sign-On (SSO) and providing a secure way for applications to access user data without exposing user credentials.
Setting Up Express.js
Before diving into OAuth 2.0 implementation, let’s set up a basic Express.js application. Ensure you have Node.js and npm installed on your machine.
1. Initialize Your Node.js Project:
```bash mkdir express-oauth cd express-oauth npm init -y ```
2. Install Required Dependencies:
```bash npm install express express-session passport passport-oauth2 ```
3. Create a Basic Express Application:
```javascript const express = require('express'); const session = require('express-session'); const passport = require('passport'); const app = express(); // Express session configuration app.use(session({ secret: 'your_secret_key', resave: false, saveUninitialized: true, })); // Initialize Passport app.use(passport.initialize()); app.use(passport.session()); app.get('/', (req, res) => { res.send('Welcome to Express with OAuth 2.0'); }); app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); }); ```
Implementing OAuth 2.0 with Express.js
Now that we have a basic Express.js setup, let’s integrate OAuth 2.0.
1. Configuring Passport with OAuth 2.0 Strategy
Passport.js is a popular middleware for handling authentication in Node.js applications. We will use the OAuth 2.0 strategy to authenticate users.
```bash npm install passport-google-oauth20 ```
2. Setting Up the OAuth 2.0 Strategy
Let’s configure Passport with the Google OAuth 2.0 strategy.
```javascript const GoogleStrategy = require('passport-google-oauth20').Strategy; passport.use(new GoogleStrategy({ clientID: 'YOUR_GOOGLE_CLIENT_ID', clientSecret: 'YOUR_GOOGLE_CLIENT_SECRET', callbackURL: 'http://localhost:3000/auth/google/callback' }, (accessToken, refreshToken, profile, done) => { // Use profile information (mainly profile.id) to check if the user is registered in your DB // Simulating user lookup return done(null, profile); })); // Serialize user to save user data into session passport.serializeUser((user, done) => { done(null, user); }); // Deserialize user to retrieve data from session passport.deserializeUser((user, done) => { done(null, user); }); ```
3. Creating Routes for Authentication
Next, define routes for initiating and handling the authentication process.
```javascript // Route to start authentication with Google app.get('/auth/google', passport.authenticate('google', { scope: ['profile', 'email'] }) ); // Google will redirect the user to this URL after approval app.get('/auth/google/callback', passport.authenticate('google', { failureRedirect: '/' }), (req, res) => { // Successful authentication res.redirect('/profile'); } ); // Profile route app.get('/profile', (req, res) => { if (req.isAuthenticated()) { res.send(`<h1>Welcome ${req.user.displayName}</h1>`); } else { res.redirect('/'); } }); // Logout route app.get('/logout', (req, res) => { req.logout(err => { if (err) return next(err); res.redirect('/'); }); }); ```
4. Testing the OAuth 2.0 Authentication
Run your Express.js application and navigate to `http://localhost:3000/auth/google`. You should be redirected to Google for authentication. Upon successful login, you’ll be redirected back to your app, where you can view the user’s profile.
Conclusion
By following this guide, you’ve implemented OAuth 2.0 authentication in an Express.js application. This method not only secures your Node.js applications but also enhances user experience by providing easy access through trusted providers like Google.
Further Reading:
Table of Contents
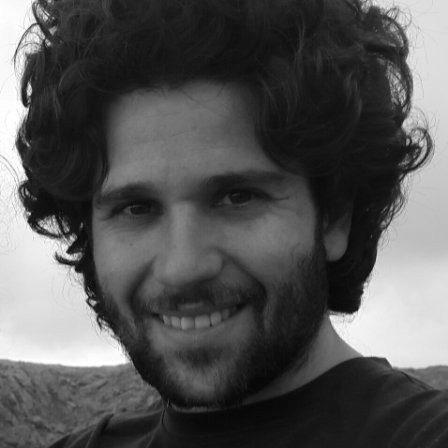
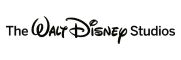