Express Middleware Demystified: A Comprehensive Guide
Express middleware is a powerful concept that lies at the heart of the Express framework in Node.js. It provides a flexible way to handle requests, responses, and route handling in a modular fashion. This article dives into the intricacies of Express middleware, offering a comprehensive guide to understanding, using, and creating middleware for your web applications.
Understanding Express Middleware
In the context of Express, middleware functions are functions that have access to the request (`req`), response (`res`), and the next middleware function in the application’s request-response cycle. Middleware can execute any code, make changes to the request and response objects, end the request-response cycle, or call the next middleware function.
Middleware is executed in the order it’s defined in the application, making it a powerful tool for handling various aspects of an HTTP request lifecycle, such as authentication, logging, error handling, and more.
Using Built-in Middleware
Express comes with several built-in middleware functions that simplify common tasks, such as serving static files, parsing JSON, and handling URL-encoded data.
Example: Using `express.json()` for JSON Parsing
The `express.json()` middleware parses incoming requests with JSON payloads and is based on the body-parser module.
```javascript const express = require('express'); const app = express(); app.use(express.json()); app.post('/data', (req, res) => { console.log(req.body); // The JSON payload will be parsed here res.send('Data received'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Creating Custom Middleware
Creating custom middleware in Express is straightforward. A custom middleware function can be as simple or as complex as needed, and it typically takes three parameters: `req`, `res`, and `next`.
Example: Logging Middleware
Here’s how you might create a simple logging middleware that logs the HTTP method and the URL of incoming requests.
```javascript const express = require('express'); const app = express(); // Custom logging middleware function logger(req, res, next) { console.log(`${req.method} ${req.url}`); next(); // Pass control to the next middleware } app.use(logger); app.get('/', (req, res) => { res.send('Home Page'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Middleware for Error Handling
Error-handling middleware is another essential aspect of Express. It allows you to handle errors in a centralized manner, providing a consistent approach to error management across your application.
Example: Error-Handling Middleware
To define an error-handling middleware, you need to include four parameters: `err`, `req`, `res`, and `next`.
```javascript const express = require('express'); const app = express(); // Custom error-handling middleware function errorHandler(err, req, res, next) { console.error(err.stack); res.status(500).send('Something broke!'); } app.get('/', (req, res) => { throw new Error('This is an error'); }); // Use the error-handling middleware app.use(errorHandler); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Third-Party Middleware
Express also supports third-party middleware, which can be installed via npm. These middleware modules can add extensive functionality to your application, such as session management, authentication, and security.
Example: Using `cookie-parser` for Cookie Management
The `cookie-parser` middleware parses cookies attached to the client request object.
```javascript const express = require('express'); const cookieParser = require('cookie-parser'); const app = express(); app.use(cookieParser()); app.get('/', (req, res) => { console.log('Cookies:', req.cookies); res.send('Check your console!'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Composing Middleware for Complex Logic
One of the powerful features of Express is the ability to compose multiple middleware functions to create more complex behaviors. Middleware can be stacked and executed in sequence, allowing you to build a robust request-handling pipeline.
Example: Middleware Stack
Here’s how you might stack multiple middleware functions to handle authentication, logging, and route handling.
```javascript const express = require('express'); const app = express(); // Authentication middleware function authenticate(req, res, next) { if (req.headers.authorization) { next(); } else { res.status(401).send('Unauthorized'); } } // Logging middleware function logger(req, res, next) { console.log(`${req.method} ${req.url}`); next(); } // Application routes app.get('/', authenticate, logger, (req, res) => { res.send('Welcome to the Home Page'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Conclusion
Express middleware is a fundamental part of developing flexible, modular, and scalable web applications in Node.js. Whether you’re using built-in middleware, creating your own, or leveraging third-party modules, mastering middleware will empower you to build more powerful and maintainable Express applications.
Further Reading:
Table of Contents
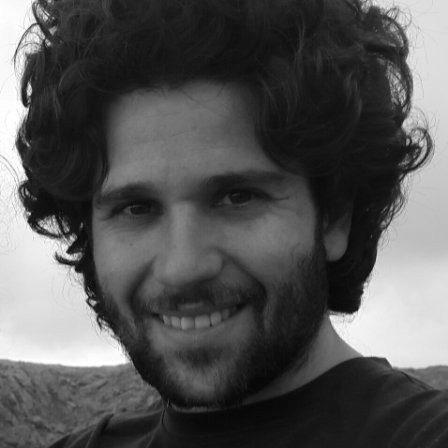
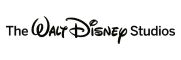