Express and MongoDB: Building Data-Driven Web Applications
In today’s digital landscape, data-driven web applications have become the backbone of numerous industries. Developers need robust tools to efficiently handle and process data. Express.js, a flexible and minimalistic web application framework, and MongoDB, a scalable and high-performance NoSQL database, together form a powerful stack for building data-driven applications. In this blog post, we will explore how to leverage the capabilities of Express and MongoDB to create efficient and scalable web applications. We will delve into code samples and best practices to help you get started on your journey to building data-driven applications.
Setting up the Development Environment
Before diving into the world of data-driven web applications, it is crucial to set up a robust development environment. Start by installing Node.js and npm. Then, create a new directory for your project and initialize it as an npm package. Install Express.js and other dependencies. Lastly, ensure you have MongoDB installed and running locally or configure a remote MongoDB instance for your application.
Code Sample:
javascript // Terminal commands $ mkdir data-driven-app $ cd data-driven-app $ npm init -y $ npm install express mongoose // index.js const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); });
Express.js Fundamentals
Express.js provides a minimalistic and flexible framework for building web applications. It offers a simple and intuitive API for handling HTTP requests and responses. With Express, you can create routes, middleware, and handle various HTTP methods. You can also render views, serve static files, and integrate with other middleware and template engines. Understanding the fundamentals of Express.js is vital for building data-driven applications.
Code Sample:
javascript const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); });
Working with MongoDB
MongoDB, a popular NoSQL database, provides a flexible and scalable solution for data storage. Start by installing MongoDB and configuring it for your application. Establish a connection to the MongoDB server using Mongoose, an object data modeling (ODM) library for MongoDB and Node.js. Create models and schemas to define the structure of your data. Perform CRUD operations such as creating, reading, updating, and deleting documents. Utilize advanced querying techniques like filtering, sorting, and pagination to retrieve data efficiently.
Code Sample:
javascript const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true, }); const schema = new mongoose.Schema({ name: String, age: Number, }); const Person = mongoose.model('Person', schema); // Creating a new document const person = new Person({ name: 'John Doe', age: 30 }); person.save(); // Querying documents Person.find({ age: { $gt: 25 } }).exec((err, people) => { if (err) { console.error(err); } else { console.log(people); } });
Building a Data-Driven Web Application with Express and MongoDB
Now that you have a solid foundation with Express and MongoDB, it’s time to build a data-driven web application. Start by creating routes and controllers to handle incoming requests. Use the models and schemas defined earlier to perform data operations. Implement functionality to create, read, update, and delete data. Render views using template engines like EJS or handlebars to display dynamic content.
Code Sample:
avascript // app.js const express = require('express'); const app = express(); const port = 3000; app.use(express.urlencoded({ extended: true })); // Routes const userRoutes = require('./routes/users'); app.use('/users', userRoutes); app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); }); // users.js (Controller) const express = require('express'); const router = express.Router(); const User = require('../models/user'); // Get all users router.get('/', async (req, res) => { try { const users = await User.find(); res.render('users/index', { users }); } catch (err) { console.error(err); res.status(500).send('Server Error'); } }); module.exports = router;
Best Practices for Performance and Security
To ensure your data-driven web application performs optimally and maintains security, follow best practices. Optimize database queries and indexes for faster retrieval. Implement caching mechanisms to reduce repetitive database calls. Sanitize user input to prevent common security vulnerabilities such as SQL injection and cross-site scripting (XSS). Implement authentication and authorization mechanisms to protect sensitive data. Utilize secure protocols (HTTPS) for transmitting data between the client and server. Regularly update and patch your dependencies to mitigate potential vulnerabilities.
Conclusion
Express and MongoDB offer a robust and efficient stack for building data-driven web applications. By leveraging the power of Express.js and MongoDB, you can create scalable and high-performance applications. In this blog post, we covered the basics of setting up the development environment, working with Express.js and MongoDB, building a data-driven web application, and best practices for performance and security. Armed with this knowledge, you are well-equipped to embark on your journey to building data-driven web applications. Remember to explore further, experiment, and always keep learning to stay ahead in the ever-evolving landscape of web development.
Table of Contents
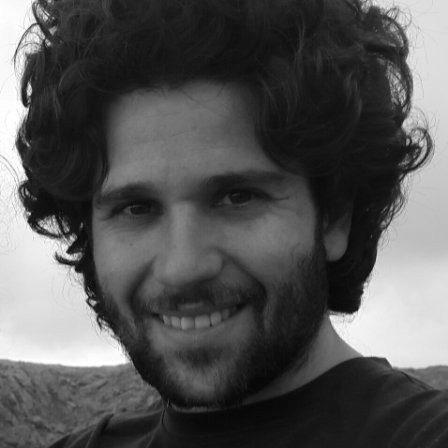
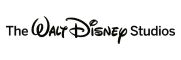