Express and OAuth: Implementing Third-Party Authentication
Authentication is a critical aspect of modern web applications, and leveraging third-party authentication mechanisms like OAuth can enhance both security and user experience. Express.js, a popular web framework for Node.js, provides a solid foundation for integrating OAuth-based authentication. This article explores how to implement OAuth in Express.js, including practical examples and best practices.
Understanding OAuth and Its Benefits
OAuth (Open Authorization) is an open standard for access delegation commonly used for token-based authentication and authorization. It allows users to grant third-party applications limited access to their resources without sharing their credentials. This approach enhances security and simplifies the login process for users.
Setting Up OAuth in an Express.js Application
Implementing OAuth in an Express.js application involves several steps, including configuring OAuth providers, setting up routes for authentication, and handling tokens. Below are key aspects and code examples demonstrating how to integrate OAuth with Express.js.
- Configuring OAuth Providers
Before integrating OAuth, you need to register your application with the OAuth provider (e.g., Google, Facebook) to obtain client credentials (Client ID and Client Secret). This information is used to authenticate your application with the provider.
- Installing Required Packages
To integrate OAuth with Express.js, you’ll need several npm packages, including `passport`, `passport-oauth2`, and the specific strategy for the OAuth provider you’re using. Install these packages using npm:
```bash npm install express passport passport-oauth2 ```
For example, to use Google OAuth, you also need:
```bash npm install passport-google-oauth20 ```
- Setting Up Passport for OAuth Authentication
Passport.js is a popular authentication middleware for Node.js that simplifies the integration of various authentication strategies, including OAuth. Here’s how you can set up Passport with Google OAuth:
```javascript const express = require('express'); const passport = require('passport'); const GoogleStrategy = require('passport-google-oauth20').Strategy; const session = require('express-session'); const app = express(); // Configure session middleware app.use(session({ secret: 'your-secret-key', resave: false, saveUninitialized: true })); // Initialize Passport and restore authentication state app.use(passport.initialize()); app.use(passport.session()); // Configure Passport to use Google OAuth passport.use(new GoogleStrategy({ clientID: 'YOUR_GOOGLE_CLIENT_ID', clientSecret: 'YOUR_GOOGLE_CLIENT_SECRET', callbackURL: 'http://localhost:3000/auth/google/callback' }, function(accessToken, refreshToken, profile, done) { // Save user profile information to session or database return done(null, profile); } )); // Serialize user information into session passport.serializeUser(function(user, done) { done(null, user); }); // Deserialize user information from session passport.deserializeUser(function(obj, done) { done(null, obj); }); ```
- Setting Up Authentication Routes
You need to create routes for handling the OAuth authentication flow. For Google OAuth, you would set up routes for initiating authentication and handling the callback:
```javascript // Route to start OAuth authentication app.get('/auth/google', passport.authenticate('google', { scope: ['profile', 'email'] }) ); // Callback route for Google OAuth app.get('/auth/google/callback', passport.authenticate('google', { failureRedirect: '/' }), function(req, res) { // Successful authentication res.redirect('/'); } ); // Route to log out app.get('/logout', function(req, res){ req.logout(); res.redirect('/'); }); // Route to display user profile app.get('/', function(req, res) { if (req.isAuthenticated()) { res.send(`<h1>Hello ${req.user.displayName}</h1><a href="/logout">Logout</a>`); } else { res.send('<h1>Home</h1><a href="/auth/google">Login with Google</a>'); } }); // Start the Express server app.listen(3000, function() { console.log('Server running on http://localhost:3000'); }); ```
- Handling Tokens and User Data
Once authenticated, you can handle user data and tokens as needed. Store tokens securely and use them to make API requests or manage user sessions.
Benefits of Using OAuth in Express.js Applications
– Enhanced Security: OAuth reduces the risk of exposing user credentials by delegating authentication to trusted third-party providers.
– Simplified User Experience: Users can log in using their existing accounts with providers like Google or Facebook, reducing friction and improving user experience.
– Access Control: OAuth allows granular control over the permissions granted to third-party applications.
Conclusion
Integrating OAuth into your Express.js application provides a secure and user-friendly authentication mechanism. By leveraging Passport.js and OAuth strategies, you can streamline the login process and enhance the security of your application. Follow best practices for managing tokens and user data to ensure a robust and reliable authentication system.
Further Reading:
Table of Contents
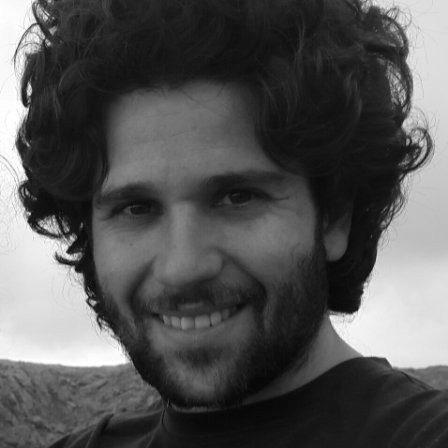
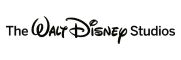