Express and Redis: Caching and Session Management
Express.js, a minimalist web framework for Node.js, is widely used for building web applications and APIs. When combined with Redis, an in-memory data store, you can significantly enhance the performance and reliability of your applications through efficient caching and session management. This blog explores how to leverage Redis in an Express.js environment to improve performance and manage sessions effectively.
Why Use Redis with Express?
Redis is a powerful, open-source, in-memory data store that supports various data structures like strings, hashes, lists, and sets. It is renowned for its high performance and is often used for caching, real-time analytics, and session management in web applications. Integrating Redis with Express allows you to:
- Cache frequently accessed data to reduce database load and improve response times.
- Manage sessions more efficiently by storing session data in memory, ensuring quick access and scalability.
Setting Up Redis with Express
To get started, you need to install the necessary packages. You’ll need Express, Redis, and `connect-redis`, a Redis session store for Express.
```bash npm install express redis connect-redis express-session ```
1. Configuring Session Management with Redis
Session management is crucial in web applications, especially for user authentication and state maintenance. By using Redis to store session data, you can ensure that session information is persistent, fast, and scalable.
Example: Setting Up Express with Redis for Session Management
```javascript const express = require('express'); const session = require('express-session'); const RedisStore = require('connect-redis')(session); const redis = require('redis'); const app = express(); const redisClient = redis.createClient(); app.use(session({ store: new RedisStore({ client: redisClient }), secret: 'your-secret-key', resave: false, saveUninitialized: false, cookie: { secure: false, maxAge: 60000 } })); app.get('/', (req, res) => { if (!req.session.views) { req.session.views = 1; } else { req.session.views++; } res.send(`Number of views: ${req.session.views}`); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); ```
In this example, we set up session management in an Express app using Redis as the session store. The session data is stored in Redis, and each time a user accesses the site, their session view count is incremented.
2. Implementing Caching with Redis
Caching is another powerful use case for Redis. By caching frequently accessed data, you can dramatically reduce the load on your primary database and improve response times.
Example: Implementing Data Caching with Redis
```javascript const express = require('express'); const redis = require('redis'); const fetch = require('node-fetch'); const app = express(); const redisClient = redis.createClient(); const fetchData = async (key) => { const response = await fetch(`https://api.example.com/data/${key}`); const data = await response.json(); return data; }; app.get('/data/:key', async (req, res) => { const { key } = req.params; redisClient.get(key, async (err, cachedData) => { if (err) throw err; if (cachedData) { res.send(JSON.parse(cachedData)); } else { const data = await fetchData(key); redisClient.setex(key, 3600, JSON.stringify(data)); res.send(data); } }); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); ```
In this example, we check if the requested data exists in the Redis cache. If it does, we return the cached data, reducing the need to fetch it from the external API. If not, we fetch the data, cache it in Redis with a 1-hour expiration, and return the response.
3. Expiring and Invalidating Cache
Redis allows you to set expiration times on cache entries, ensuring that stale data is removed automatically. You can also manually invalidate or update cache entries when necessary.
Example: Manually Invalidating Cache Entries
```javascript app.post('/invalidate-cache/:key', (req, res) => { const { key } = req.params; redisClient.del(key, (err, response) => { if (err) throw err; res.send(response ? `Cache for ${key} cleared` : `Cache for ${key} not found`); }); }); ```
This route allows you to manually clear the cache for a specific key, ensuring that subsequent requests fetch fresh data.
Conclusion
By integrating Redis with Express, you can significantly improve the performance and scalability of your web applications. Whether you’re using Redis for caching to speed up data access or for managing user sessions, its powerful features can enhance your application’s efficiency and reliability.
Further Reading:
Table of Contents
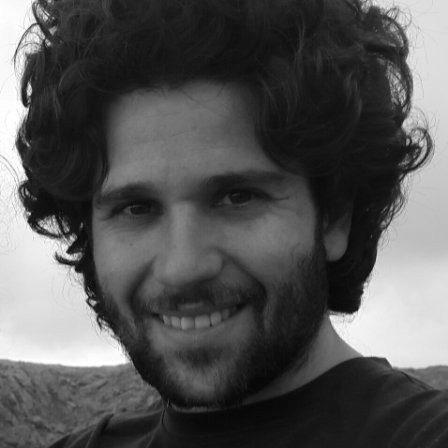
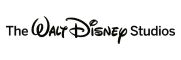