Securing Express Applications: Best Practices and Strategies
In today’s digital landscape, application security is of utmost importance. With the rising number of cyber threats, it is crucial to adopt robust security measures to protect your Express applications. This blog will guide you through the best practices and strategies to secure your Express applications effectively. From input validation and authentication to handling sensitive data and preventing common security vulnerabilities, we’ll cover it all. Let’s dive in!
Input Validation: Protecting Against Injection Attacks
One of the most common security vulnerabilities is injection attacks. By ensuring proper input validation, you can prevent malicious code from being executed. Use libraries like express-validator to validate and sanitize user input before processing it in your routes. Here’s an example:
javascript const { body, validationResult } = require('express-validator'); app.post('/login', [ body('username').isLength({ min: 3 }), body('password').isLength({ min: 8 }), ], (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Process login request });
Authentication and Authorization: Safeguarding User Access
Implement secure authentication and authorization mechanisms to ensure that only authorized users can access your application’s resources. Use strong password hashing algorithms like bcrypt or Argon2 and employ techniques like session-based authentication or token-based authentication (JWT). Here’s an example using Passport.js for authentication:
javascript const passport = require('passport'); const LocalStrategy = require('passport-local').Strategy; passport.use(new LocalStrategy( (username, password, done) => { // Validate username and password // Call done(null, user) if authenticated // Call done(null, false) if authentication fails } )); app.post('/login', passport.authenticate('local'), (req, res) => { // Successful authentication });
Handling Sensitive Data: Encryption and Data Protection
To protect sensitive data, such as user passwords or API keys, ensure that it is encrypted both in transit and at rest. Use HTTPS for secure communication, and store sensitive information securely by utilizing encryption algorithms and secure storage mechanisms. Here’s an example using the crypto module for encryption:
javascript const crypto = require('crypto'); const encryptData = (data, key) => { const cipher = crypto.createCipher('aes-256-cbc', key); let encrypted = cipher.update(data, 'utf8', 'hex'); encrypted += cipher.final('hex'); return encrypted; }; const encryptedData = encryptData('sensitive information', 'encryptionKey');
Securing Express Routes: Implementing Access Control
Control access to your routes based on user roles and permissions. Create middleware functions to validate user access and implement role-based or attribute-based access control (RBAC/ABAC). Here’s an example of a middleware function for access control:
javascript const authorize = (requiredRole) => (req, res, next) => { if (req.user.role === requiredRole) { next(); // User has required role, proceed to the next middleware } else { res.status(403).json({ error: 'Access denied' }); } }; app.get('/admin', authorize('admin'), (req, res) => { // Only admin users can access this route });
Preventing Cross-Site Scripting (XSS) Attacks
Protect your application from XSS attacks by properly sanitizing user-generated content and utilizing output encoding. Use libraries like xss or template engines with built-in protection mechanisms, such as EJS or Handlebars. Here’s an example using EJS:
javascript const ejs = require('ejs'); app.get('/post/:id', (req, res) => { const postId = req.params.id; const post = // Fetch post from the database res.render('post', { post: ejs.escapeXML(post) }); });
Protecting Against Cross-Site Request Forgery (CSRF)
Implement CSRF protection to prevent unauthorized requests. Use CSRF tokens and verify them on sensitive operations. Libraries like csurf can simplify this process. Here’s an example using csurf:
javascript const csurf = require('csurf'); app.use(csurf({ cookie: true })); app.get('/transfer', (req, res) => { const csrfToken = req.csrfToken(); res.render('transfer', { csrfToken }); }); app.post('/transfer', (req, res) => { // Verify CSRF token before processing the transfer });
Implementing Rate Limiting and Request Throttling
Protect your application from abuse and DoS attacks by implementing rate limiting and request throttling. Use libraries like express-rate-limit or express-slow-down to control the number of requests from an IP address or a specific user. Here’s an example using express-rate-limit:
javascript const rateLimit = require('express-rate-limit'); const apiLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // Limit each IP to 100 requests per windowMs }); app.use('/api/', apiLimiter);
Logging and Error Handling: Monitoring for Security Issues
Implement robust logging and error handling mechanisms to detect and respond to security incidents. Log relevant security events and errors and set up alerts or monitoring systems to track suspicious activities. Use libraries like winston or integrate with logging services like Loggly or Papertrail.
javascript const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.simple(), transports: [ new winston.transports.Console(), new winston.transports.File({ filename: 'logs/error.log', level: 'error' }), ], }); app.use((err, req, res, next) => { logger.error(err.stack); res.status(500).json({ error: 'Internal Server Error' }); });
Keeping Dependencies Up to Date: Patching Vulnerabilities
Regularly update and patch your dependencies to address security vulnerabilities. Use tools like npm audit or snyk to scan for known vulnerabilities in your project dependencies and ensure they are up to date. Update your packages regularly and consider using tools like automated dependency update services.
Implementing Content Security Policies (CSP)
Content Security Policies (CSP) help mitigate the risks of cross-site scripting (XSS) attacks, clickjacking, and other malicious activities. Define and enforce a strong CSP to control the sources from which your application can load content. Here’s an example of setting up a CSP header:
javascript const helmet = require('helmet'); app.use(helmet.contentSecurityPolicy({ directives: { defaultSrc: ["'self'"], scriptSrc: ["'self'", "https://example.com"], styleSrc: ["'self'", "'unsafe-inline'"], // Add other directives as per your application's requirements }, }));
Conclusion
Securing Express applications is essential to protect against evolving cyber threats. By following the best practices and strategies outlined in this blog, you can significantly enhance the security of your applications. Remember to consistently update your dependencies, validate user input, implement proper authentication and authorization mechanisms, and protect sensitive data. Stay vigilant and up-to-date with the latest security practices to ensure a secure and trustworthy application for your users.
Incorporating these security measures will go a long way in safeguarding your Express applications from common vulnerabilities and ensuring the protection of your users’ data. Implement them diligently and remain proactive in staying ahead of potential threats. With robust security practices in place, you can build reliable and trustworthy applications that instill confidence in your users.
Remember, security is an ongoing effort, and it requires continuous monitoring and adaptation. Stay informed about the latest security trends, updates, and best practices to fortify your applications against emerging threats. Stay safe, stay secure!
Table of Contents
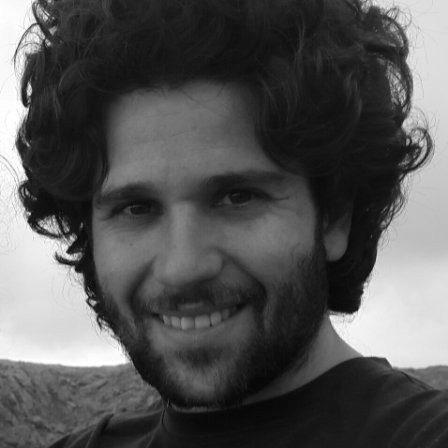
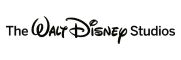