Express and WebSocket: Building Real-Time Communication
Real-time communication is a critical component of modern web applications, enabling instant data exchange between clients and servers. Express, a fast and minimalistic web framework for Node.js, combined with WebSocket, a protocol providing full-duplex communication channels over a single TCP connection, offers a powerful solution for implementing real-time features. This article explores how to integrate WebSocket with Express, providing practical examples to help you build responsive, interactive web applications.
Understanding Real-Time Communication
Real-time communication allows data to be transmitted instantly between clients and servers, without the need for constant polling or refreshing. This capability is essential for applications like chat apps, live notifications, online gaming, and collaborative tools.
Setting Up Express with WebSocket
To start building a real-time application with Express and WebSocket, you’ll first need to set up a basic Express server. Then, you can integrate WebSocket to handle real-time communication.
1. Setting Up a Basic Express Server
First, let’s create a simple Express server. You’ll need to install Express using npm.
```bash npm install express ```
Now, create an `index.js` file to set up the server:
```javascript const express = require('express'); const app = express(); const http = require('http').Server(app); const port = 3000; app.get('/', (req, res) => { res.send('<h1>Hello, Express!</h1>'); }); http.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); }); ```
This code sets up a basic Express server that listens on port 3000.
2. Integrating WebSocket for Real-Time Communication
To add WebSocket support, you’ll use the `ws` library. Install it using npm:
```bash npm install ws ```
Next, modify your `index.js` file to include WebSocket:
```javascript const WebSocket = require('ws'); const wss = new WebSocket.Server({ server: http }); wss.on('connection', (ws) => { console.log('New client connected'); ws.on('message', (message) => { console.log(`Received message: ${message}`); ws.send(`Server: ${message}`); }); ws.on('close', () => { console.log('Client disconnected'); }); }); ```
With this setup, your Express server can now handle WebSocket connections. When a client sends a message, the server echoes it back, demonstrating a simple real-time interaction.
Real-Time Chat Application Example
Let’s build a basic chat application to illustrate how real-time communication works with Express and WebSocket.
1. Creating the Client Side
For the client side, create an `index.html` file:
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Chat App</title> </head> <body> <h1>Chat Room</h1> <div id="chat"></div> <input id="messageInput" type="text" placeholder="Type a message..." /> <button id="sendButton">Send</button> <script> const socket = new WebSocket('ws://localhost:3000'); socket.onmessage = function(event) { const chat = document.getElementById('chat'); chat.innerHTML += `<p>${event.data}</p>`; }; document.getElementById('sendButton').onclick = function() { const input = document.getElementById('messageInput'); socket.send(input.value); input.value = ''; }; </script> </body> </html> ```
This HTML file creates a simple interface for a chat room. It establishes a WebSocket connection and allows users to send messages, which are then displayed in the chat area.
2. Handling Multiple Clients
The example above works for a single client, but you often need to handle multiple clients. Modify the server code to broadcast messages to all connected clients:
```javascript wss.on('connection', (ws) => { ws.on('message', (message) => { wss.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(`Client: ${message}`); } }); }); }); ```
Now, when one client sends a message, it will be broadcast to all connected clients, enabling real-time communication between multiple users.
Scaling Real-Time Applications
For larger applications, you may need to scale your WebSocket implementation. Consider using solutions like Redis for message brokering or Socket.IO for a more feature-rich real-time framework.
Conclusion
By integrating Express and WebSocket, you can build powerful real-time communication features in your web applications. Whether you’re developing a chat app, live notifications, or collaborative tools, this combination provides the flexibility and performance needed to deliver dynamic user experiences. As you expand your application, remember to consider scalability and explore additional tools like Socket.IO and Redis to enhance your real-time communication capabilities.
Further Reading
Table of Contents
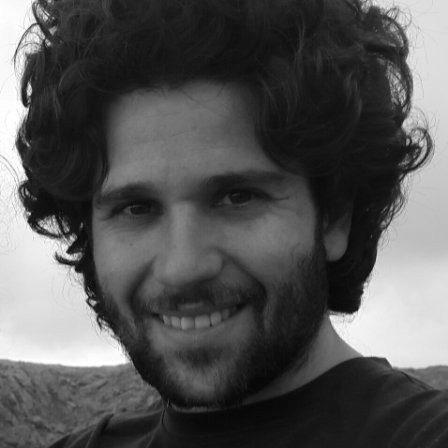
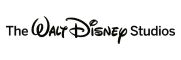