Hire Full Stack Developers Interview Questions Guide
Full Stack Developers are versatile professionals proficient in both frontend and backend technologies, capable of developing end-to-end solutions. When hiring Full Stack Developers, it’s crucial to assess their proficiency in multiple programming languages, web frameworks, and their ability to work seamlessly across the entire development stack. This guide will help you navigate the hiring process effectively, enabling you to identify candidates with the right skills for your full stack development projects.
Table of Contents
1. Reasons to Hire Full Stack Developers
Embarking on the journey to hire Full Stack Developers? Here are key reasons to consider:
1.1 End-to-End Development
Full Stack Developers can handle both frontend and backend development, streamlining the development process and reducing dependencies.
1.2 Versatility
Proficient in multiple technologies, Full Stack Developers can adapt to changing project requirements and contribute to various aspects of a project.
1.3 Faster Problem Resolution
With knowledge across the entire stack, Full Stack Developers can identify and resolve issues more efficiently, ensuring a smoother development process.
2. How to Hire Full Stack Developers
Follow these steps for a successful Full Stack Developer hiring process:
2.1 Job Requirements
Define specific job prerequisites, emphasizing skills in both frontend and backend technologies, proficiency in databases, and experience with full stack development.
2.2 Search Channels
Utilize CloudDevs’ expertise to connect with potential Full Stack Developers. Leverage job postings, online platforms, and tech communities to discover talented individuals.
2.3 Screening
Scrutinize candidates’ proficiency in multiple programming languages, experience with popular frameworks, and their ability to work across the entire development stack.
2.4 Technical Assessment
Develop a comprehensive technical assessment covering both frontend and backend development, including coding challenges and real-world scenarios.
3. Core Skills of Full Stack Developers to Look For
When evaluating Full Stack Developers, focus on these core skills:
- Frontend Technologies: Proficiency in HTML, CSS, JavaScript, and popular frontend frameworks like React, Angular, or Vue.js.
- Backend Technologies: Experience with server-side programming languages such as Node.js, Python, Java, or others.
- Databases: Knowledge of database management systems, both SQL and NoSQL.
- API Development: Ability to design and implement APIs for seamless communication between frontend and backend components.
- Version Control: Familiarity with version control systems like Git for collaborative development.
- Problem-Solving: Aptitude for identifying challenges and implementing efficient solutions across the entire development stack.
4. Overview of the Full Stack Developer Hiring Process
Here’s an overview of the Full Stack Developer hiring process:
4.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking in Full Stack Developers.
4.2 Crafting Compelling Job Descriptions
Create engaging job descriptions that accurately convey the role, emphasizing Full Stack-specific skills required.
4.3 Crafting Full Stack Developer Interview Questions
Develop a comprehensive set of interview questions covering both frontend and backend development, problem-solving aptitude, and relevant technologies.
5. Sample Full Stack Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ Full Stack development skills:
Q1. Implement a React component that fetches data from a backend API and displays it.
A:
// Sample Answer: React component fetching and displaying data import React, { useState, useEffect } from 'react'; function DataComponent() { const [data, setData] = useState([]); useEffect(() => { fetchData(); }, []); const fetchData = async () => { try { const response = await fetch('https://api.example.com/data'); const result = await response.json(); setData(result); } catch (error) { console.error('Error:', error); } }; return ( <div> {data.map(item => ( <div key={item.id}>{item.name}</div> ))} </div> ); } export default DataComponent;
Q2. Write a Node.js script to create a simple HTTP server and serve a static HTML file.
A:
// Sample Answer: Node.js script to create an HTTP server const http = require('http'); const fs = require('fs'); const server = http.createServer((req, res) => { fs.readFile('index.html', (error, data) => { if (error) { res.writeHead(500, { 'Content-Type': 'text/plain' }); res.end('Internal Server Error'); } else { res.writeHead(200, { 'Content-Type': 'text/html' }); res.end(data); } }); }); const PORT = 3000; server.listen(PORT, () => { console.log(`Server running at http://localhost:${PORT}/`); });
Q3. Explain the concept of RESTful APIs and how they work.
A:
RESTful APIs (Representational State Transfer) use standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources. They follow the principles of statelessness, allowing clients to interact with resources on the server. Resources are identified by URIs, and data is transferred as JSON or XML.
Q4. Describe the process of handling user authentication in a full stack application.
A:
User authentication involves the following steps:
- User Registration: Collect user credentials and store them securely in the database.
- User Login: Verify user credentials during login.
- Token Generation: After successful login, generate and send an authentication token to the client.
- Token Verification: For each subsequent request, verify the authentication token on the server to ensure the user is authenticated.
Q5. Write a SQL query to retrieve data from two tables with a foreign key relationship.
A:
-- Sample Answer: SQL query to retrieve data from two tables SELECT * FROM table1 JOIN table2 ON table1.id = table2.table1_id;
Q6. Implement a simple Express.js server with a RESTful API endpoint.
A:
// Sample Answer: Express.js server with a RESTful API endpoint const express = require('express'); const app = express(); const PORT = 3000; app.get('/api/data', (req, res) => { const data = { id: 1, name: 'Sample Data' }; res.json(data); }); app.listen(PORT, () => { console.log(`Server running at http://localhost:${PORT}`); });
Q7. Explain the concept of middleware in the context of Express.js.
A:
Middleware functions in Express.js have access to the request, response, and the next middleware function. They can modify the request and response objects, end the request-response cycle, and call the next middleware function in the stack.
Q8. What is CORS, and how does it affect web applications?
A:
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers. It controls how web pages in one domain can request and interact with resources from another domain. CORS headers must be present on the server to allow or restrict cross-origin requests.
Q9. Write a JavaScript function to add an item to the end of an array.
A:
// Sample Answer: JavaScript function to add an item to the end of an array function addItemToArray(arr, item) { arr.push(item); } // Example usage: const myArray = [1, 2, 3]; addItemToArray(myArray, 4); console.log(myArray); // Output: [1, 2, 3, 4]
Q10. Describe the differences between localStorage and sessionStorage in web browsers.
A:
- Scope: localStorage has a larger scope and persists even when the browser is closed. sessionStorage has a smaller scope and is limited to the duration of the page session.
- Data Retention: Data stored in localStorage remains until explicitly cleared. Data in sessionStorage is cleared when the page session ends.
6. Hiring Full Stack Developers through CloudDevs
Step 1: Connect with CloudDevs
Initiate a conversation with a CloudDevs consultant to discuss your project requirements, preferred skills, and expected experience.
Step 2: Discover Your Ideal Match
Within a short timeframe, CloudDevs presents you with carefully selected Full Stack Developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial
Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional Full Stack Developers, ensuring your team possesses the skills required to build robust and comprehensive web applications.
7. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess Full Stack Developers comprehensively. Whether you’re developing web applications, e-commerce platforms, or complex business solutions, securing the right Full Stack Developers for your team is pivotal to the success of your full stack development projects.
Table of Contents
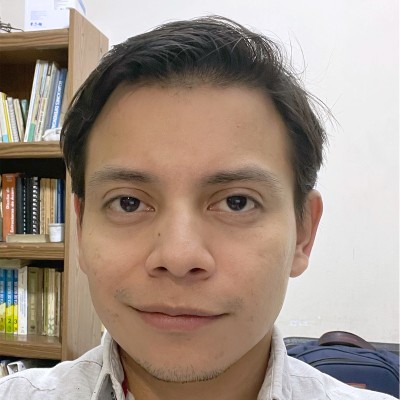
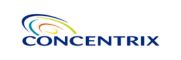