Gatsby and GraphQL: Harnessing the Full Potential
Gatsby is a blazing-fast, modern website generator that leverages React and GraphQL. It’s built on the JAMstack architecture, allowing developers to create performant websites that can be easily deployed to a CDN. Gatsby uses a static site generator to pre-render pages at build time, resulting in near-instantaneous page loads and enhanced SEO.
1. What is GraphQL?
GraphQL is a query language and runtime for APIs that enables efficient data fetching and manipulation. It provides a flexible approach to data querying, allowing clients to specify exactly what data they need from the server. With GraphQL, you can avoid over-fetching or under-fetching data, improving performance and reducing bandwidth usage.
2. Why Gatsby and GraphQL?
The combination of Gatsby and GraphQL offers several compelling advantages. Firstly, Gatsby’s static site generation paired with GraphQL’s efficient data retrieval allows for lightning-fast websites. Secondly, GraphQL’s declarative nature makes it easy to fetch data from multiple sources and merge them into a single response. This flexibility is especially useful when dealing with complex data structures. Finally, Gatsby’s plugin ecosystem provides excellent GraphQL integration, allowing you to seamlessly incorporate data from various sources.
3. Getting Started with Gatsby and GraphQL
3.1 Installation
To get started with Gatsby, ensure that you have Node.js and npm installed. Then, install the Gatsby CLI globally using the following command:
bash npm install -g gatsby-cli
3.2 Creating a Gatsby Project
Once the CLI is installed, you can create a new Gatsby project using the following command:
bash gatsby new my-gatsby-project
This command creates a new Gatsby project with the name “my-gatsby-project” in a directory of the same name.
4. Leveraging GraphQL in Gatsby
4.1 Understanding GraphQL Queries
GraphQL queries define the data structure and shape of the response. In Gatsby, you can write queries using the GraphiQL interface. Let’s consider an example where we want to fetch a list of blog posts:
graphql query { allMarkdownRemark { edges { node { frontmatter { title date } } } } } }
This query fetches the title and date fields from all MarkdownRemark nodes in the GraphQL schema.
4.2 Fetching Data with GraphQL
To fetch data in Gatsby, you can use the useStaticQuery hook provided by Gatsby. Here’s an example of fetching and rendering blog post data:
jsx import React from 'react'; import { useStaticQuery, graphql } from 'gatsby'; const BlogPosts = () => { const data = useStaticQuery(graphql` query { allMarkdownRemark { edges { node { frontmatter { title date } } } } } `); return ( <div> {data.allMarkdownRemark.edges.map(({ node }) => ( <div key={node.frontmatter.title}> <h2>{node.frontmatter.title}</h2> <p>{node.frontmatter.date}</p> </div> ))} </div> ); }; export default BlogPosts;
In this example, we’re using the useStaticQuery hook to fetch data and then rendering it dynamically.
4.3 Rendering Data with Gatsby
Gatsby’s static site generation allows you to pre-render data at build time. You can use GraphQL queries to populate static pages with data, improving performance. Here’s an example of dynamically generating pages for blog posts:
jsx // gatsby-node.js exports.createPages = async ({ actions, graphql }) => { const { createPage } = actions; const result = await graphql(` query { allMarkdownRemark { edges { node { frontmatter { slug } } } } } `); result.data.allMarkdownRemark.edges.forEach(({ node }) => { createPage({ path: node.frontmatter.slug, component: require.resolve('./src/templates/BlogPostTemplate.js'), context: { slug: node.frontmatter.slug, }, }); }); };
In this code snippet, we’re querying for all MarkdownRemark nodes and creating dynamic pages for each blog post using the createPage action.
5. Optimizing Performance with Gatsby and GraphQL
5.1 Using GraphQL Fragments
GraphQL fragments allow you to define reusable units of queries. By using fragments, you can minimize duplicate code and improve query maintainability. Here’s an example:
graphql fragment BlogPostFields on MarkdownRemark { frontmatter { title date } }
You can then use this fragment in multiple queries like so:
graphql query { allMarkdownRemark { edges { node { ...BlogPostFields } } } }
5.2 Implementing Pagination
To implement pagination in Gatsby with GraphQL, you can use the limit and skip arguments. Here’s an example of fetching blog posts with pagination:
graphql query { allMarkdownRemark(limit: 5, skip: 0) { edges { node { ...BlogPostFields } } } }
In this example, we’re fetching the first 5 blog posts.
5.3 Caching and Incremental Builds
Gatsby leverages GraphQL’s caching capabilities to improve build times. When data changes, Gatsby can invalidate the cache for affected queries and incrementally rebuild only the necessary parts of the site. This approach drastically reduces build times for large projects.
6. Real-time Updates with Gatsby and GraphQL
6.1 Webhooks and Triggers
Gatsby offers various plugins that enable real-time updates using webhooksand triggers. These plugins can listen for events such as content updates in a headless CMS or changes in a database. When triggered, Gatsby can automatically rebuild the affected pages, ensuring your site stays up to date.
6.2 Subscriptions with GraphQL
GraphQL subscriptions allow you to receive real-time updates from the server. You can use subscriptions to listen for changes in your data and update your Gatsby site accordingly. This is particularly useful for applications that require live data updates, such as chat applications or real-time analytics dashboards.
Conclusion
Gatsby and GraphQL form a powerful duo that enables developers to create blazing-fast and dynamic websites. Gatsby’s static site generation combined with GraphQL’s efficient data fetching capabilities provides unmatched performance and flexibility. With Gatsby’s plugin ecosystem and GraphQL’s declarative querying, you can seamlessly integrate various data sources and build complex websites with ease.
In this blog post, we explored the fundamentals of Gatsby and GraphQL, discussed their benefits, and provided code samples to help you get started. By harnessing the full potential of Gatsby and GraphQL, you can create high-performance websites that delight users and empower developers.
Start your journey with Gatsby and GraphQL today and unlock a new level of web development possibilities.
Table of Contents
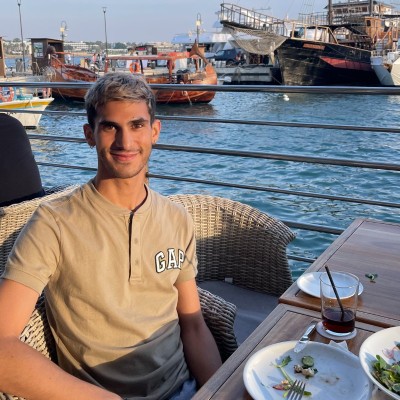
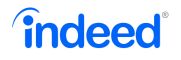