Advanced Networking in Go: Exploring TCP, UDP, and HTTP/2
In the world of modern applications, networking plays a crucial role in enabling communication between various components. As a developer, understanding advanced networking concepts is essential for building high-performance and scalable applications. Go, also known as Golang, is a powerful programming language that excels in concurrent and networked applications. In this blog, we will explore three essential networking protocols in Go: TCP, UDP, and HTTP/2. We’ll cover their fundamentals, how to work with them, and provide code samples for a better understanding.
1. Introduction to Networking in Go
Go’s standard library provides comprehensive support for networking, making it easy to work with various protocols. Networking in Go is built around the concepts of goroutines, which enable concurrent communication, and interfaces, which provide flexibility in working with different protocols.
Before diving into specific protocols, let’s briefly discuss how Go handles networking:
- Goroutines: Goroutines are lightweight threads that allow you to perform concurrent operations. This makes it easy to handle multiple connections simultaneously.
- Interfaces: Go’s interfaces allow you to abstract different types of network connections. This means you can write networking code that works with both TCP and UDP using the same interface methods.
Now, let’s explore TCP communication in Go.
2. TCP Communication in Go
TCP (Transmission Control Protocol) is a reliable and connection-oriented protocol. It ensures that data packets arrive in order and without corruption. Let’s see how to set up a simple TCP server and client in Go.
2.1. TCP Server Setup
go package main import ( "fmt" "net" ) func handleConnection(conn net.Conn) { defer conn.Close() // Read data from the connection buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } // Process data (In this case, we'll just print it) data := buffer[:n] fmt.Println("Received:", string(data)) } func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { fmt.Println("Error:", err.Error()) return } defer listener.Close() fmt.Println("TCP Server listening on :8080") for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting connection:", err.Error()) continue } go handleConnection(conn) } }
In this example, we create a TCP server listening on port 8080. When a client connects, the handleConnection function is called in a separate goroutine to process the incoming data.
2.2. TCP Client Setup
go package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "localhost:8080") if err != nil { fmt.Println("Error:", err.Error()) return } defer conn.Close() message := "Hello, TCP Server!" _, err = conn.Write([]byte(message)) if err != nil { fmt.Println("Error sending data:", err.Error()) return } fmt.Println("Message sent:", message) }
In this TCP client example, we connect to the server running on localhost:8080 and send a simple message.
2.3. Handling Multiple Connections
In real-world applications, the server needs to handle multiple client connections concurrently. Go’s concurrency model using goroutines makes it easy:
go // ... func main() { // ... for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting connection:", err.Error()) continue } go handleConnection(conn) } }
By launching a new goroutine for each incoming connection, the server can handle multiple clients simultaneously.
3. UDP Communication in Go
UDP (User Datagram Protocol) is a connectionless and lightweight protocol suitable for scenarios where data loss is acceptable or managed at a higher level. Unlike TCP, UDP doesn’t guarantee packet delivery or order. Let’s set up a simple UDP server and client in Go.
3.1. UDP Server Setup
go package main import ( "fmt" "net" ) func main() { // Listen for incoming UDP packets conn, err := net.ListenUDP("udp", &net.UDPAddr{ IP: net.IPv4(0, 0, 0, 0), Port: 8081, }) if err != nil { fmt.Println("Error:", err.Error()) return } defer conn.Close() fmt.Println("UDP Server listening on :8081") buffer := make([]byte, 1024) for { n, addr, err := conn.ReadFromUDP(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } // Process data (In this case, we'll just print it) data := buffer[:n] fmt.Println("Received from", addr.String(), ":", string(data)) } }
In this UDP server example, we bind it to port 8081 and listen for incoming UDP packets.
3.2. UDP Client Setup
go package main import ( "fmt" "net" ) func main() { conn, err := net.DialUDP("udp", nil, &net.UDPAddr{ IP: net.IPv4(127, 0, 0, 1), Port: 8081, }) if err != nil { fmt.Println("Error:", err.Error()) return } defer conn.Close() message := "Hello, UDP Server!" _, err = conn.Write([]byte(message)) if err != nil { fmt.Println("Error sending data:", err.Error()) return } fmt.Println("Message sent:", message) }
In the UDP client example, we use the DialUDP function to establish a connection and send a message to the server running on localhost:8081.
3.3. Broadcast and Multicast
UDP also supports broadcast and multicast communication. Broadcasting sends data to all devices in the network, while multicast sends data to a specific group of devices. Broadcasting is useful for server discovery, while multicast is efficient for group communication.
1. Broadcasting in Go:
go // ... func main() { conn, err := net.DialUDP("udp", nil, &net.UDPAddr{ IP: net.IPv4bcast, Port: 8081, }) if err != nil { fmt.Println("Error:", err.Error()) return } defer conn.Close() message := "Hello, UDP Broadcast!" _, err = conn.Write([]byte(message)) if err != nil { fmt.Println("Error sending data:", err.Error()) return } fmt.Println("Broadcast message sent:", message) }
2. Multicast in Go:
go // ... func main() { interfaceAddr := "en0" // Replace with the desired network interface multicastAddr := "239.0.0.1:8082" // Listen for incoming UDP packets on the multicast address multicastConn, err := net.ListenMulticastUDP("udp", nil, &net.UDPAddr{ IP: net.ParseIP(multicastAddr), Port: 8082, }) if err != nil { fmt.Println("Error:", err.Error()) return } defer multicastConn.Close() // Join the multicast group on the specified network interface interfaceIP := getInterfaceIP(interfaceAddr) if interfaceIP == nil { fmt.Println("Failed to find interface address.") return } multicastConn.JoinGroup(interfaceIP) fmt.Println("Multicast Server listening on", multicastAddr) buffer := make([]byte, 1024) for { n, addr, err := multicastConn.ReadFromUDP(buffer) if err != nil { fmt.Println("Error reading:", err.Error()) return } // Process data (In this case, we'll just print it) data := buffer[:n] fmt.Println("Received from", addr.String(), ":", string(data)) } } // Helper function to get the IP address of the network interface func getInterfaceIP(name string) *net.IP { interface, err := net.InterfaceByName(name) if err != nil { return nil } addrs, err := interface.Addrs() if err != nil { return nil } for _, addr := range addrs { if ipnet, ok := addr.(*net.IPNet); ok && !ipnet.IP.IsLoopback() && ipnet.IP.To4() != nil { return &ipnet.IP } } return nil }
Remember to replace en0 in the getInterfaceIP function with the desired network interface name.
4. Introducing HTTP/2 in Go
HTTP/2 is the latest version of the Hypertext Transfer Protocol, designed to improve web performance and security. It introduces features like multiplexing, server push, and header compression. Let’s see how to set up a simple HTTP/2 server and client in Go.
4.1. HTTP/2 Server Setup
go package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, HTTP/2 Server!") } func main() { http.HandleFunc("/", handler) err := http.ListenAndServeTLS(":8083", "server.crt", "server.key", nil) if err != nil { fmt.Println("Error:", err.Error()) return } }
In this example, we create an HTTP/2 server listening on port 8083. The server responds to all requests with “Hello, HTTP/2 Server!”
4.2. HTTP/2 Client Setup
go package main import ( "fmt" "net/http" "golang.org/x/net/http2" ) func main() { client := &http.Client{ Transport: &http2.Transport{}, } resp, err := client.Get("https://localhost:8083") if err != nil { fmt.Println("Error:", err.Error()) return } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println("Error reading response:", err.Error()) return } fmt.Println("Response:", string(body)) }
The HTTP/2 client example uses the golang.org/x/net/http2 package to enable HTTP/2 transport. The client sends an HTTP GET request to the server and prints the response.
4.3. Handling HTTP/2 Requests and Responses
To handle specific HTTP/2 requests and responses, you can use the standard http package’s Request and Response structs. Here’s an example of handling POST requests:
go package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { if r.Method == http.MethodPost { // Handle POST request // ... } else { http.Error(w, "Method not allowed", http.StatusMethodNotAllowed) } } func main() { http.HandleFunc("/", handler) err := http.ListenAndServeTLS(":8083", "server.crt", "server.key", nil) if err != nil { fmt.Println("Error:", err.Error()) return } }
5. Advanced Networking Concepts
In addition to basic communication, advanced networking involves various optimizations and security measures. Here are some concepts worth exploring:
5.1. TLS/SSL Encryption
TLS (Transport Layer Security) and SSL (Secure Sockets Layer) provide secure communication over a network by encrypting data during transmission. Go’s crypto/tls package enables you to implement TLS/SSL encryption in your network applications.
5.2. Connection Pooling
Connection pooling helps manage a pool of reusable network connections, reducing the overhead of establishing new connections for each request. By reusing connections, you can improve performance and reduce latency in your networked applications.
5.3. Load Balancing
Load balancing involves distributing network traffic across multiple servers to ensure optimal resource utilization and prevent server overload. Implementing load balancing in Go can significantly enhance the scalability and reliability of your applications.
Conclusion
In this blog, we explored the fundamentals of TCP, UDP, and HTTP/2 protocols in Go. We covered setting up servers and clients for each protocol with code samples and discussed advanced networking concepts like TLS/SSL encryption, connection pooling, and load balancing. Armed with this knowledge, you can build high-performance and scalable networked applications using Go. So go ahead and start experimenting with advanced networking in Go to take your applications to the next level! Happy coding!
Table of Contents
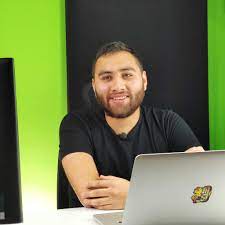
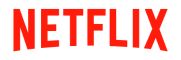