Deploying Go Applications on Kubernetes: Container Orchestration
Containerization and orchestration have revolutionized the way applications are deployed and managed in modern software development. Kubernetes, a powerful container orchestration platform, has become the go-to choice for managing containerized applications at scale. If you’re a Go developer looking to deploy your applications on Kubernetes, you’re in the right place.
In this comprehensive guide, we will walk you through the process of deploying Go applications on Kubernetes, covering everything from setting up your development environment to deploying your application in a production-ready Kubernetes cluster. By the end of this tutorial, you’ll have a solid understanding of how to leverage Kubernetes for container orchestration of your Go applications.
1. Prerequisites
Before we start deploying our Go application on Kubernetes, we need to set up our development environment and ensure we have the necessary tools installed.
1.1. Setting up a Go Development Environment
If you haven’t already, you’ll need to install Go on your local machine. You can download the latest version of Go from the official website.
To verify that Go is installed correctly, open a terminal and run:
shell go version
You should see the installed Go version displayed.
1.2. Installing Docker
Docker is essential for containerizing our Go application. You can download and install Docker from the Docker website.
After installing Docker, verify it’s working by running:
shell docker --version
1.3. Installing Kubernetes (Minikube or Other Options)
You have several options for installing Kubernetes locally, but for simplicity, we’ll use Minikube, which is a tool that sets up a single-node Kubernetes cluster on your local machine. You can install Minikube following the instructions on the official website.
After installing Minikube, start the cluster with:
shell minikube start
Your Kubernetes cluster is now up and running on your local machine. Ensure it’s working correctly by running:
shell kubectl version --short
You should see both the Client and Server versions displayed.
With our development environment and Kubernetes cluster set up, we can move on to containerizing our Go application.
2. Containerizing Your Go Application
Containerizing your Go application involves creating a Docker image that encapsulates your application and its dependencies. To do this, we’ll create a Dockerfile that defines how the image should be built.
2.1. Writing a Dockerfile
Create a file named Dockerfile in your Go application’s root directory with the following content:
dockerfile # Use an official Go runtime as the base image FROM golang:1.16 # Set the working directory inside the container WORKDIR /app # Copy the Go application source code into the container COPY . . # Build the Go application RUN go build -o main # Expose a port for the Go application to listen on EXPOSE 8080 # Command to run the Go application CMD ["./main"]
This Dockerfile uses the official Go base image, sets up the working directory, copies your Go application’s source code, builds it, and defines the command to run the application. Make sure to adjust the version of Go in the FROM line to match your application’s requirements.
2.2. Building and Pushing the Docker Image
Now that we have our Dockerfile, we can build the Docker image. Open a terminal, navigate to your application’s directory, and run:
shell docker build -t my-go-app:v1 .
This command builds a Docker image with the tag my-go-app:v1 from the current directory (.). Replace my-go-app:v1 with the desired image name and version.
Next, we need to push the Docker image to a container registry. Popular container registries include Docker Hub, Google Container Registry, and Amazon Elastic Container Registry (ECR). For this example, we’ll use Docker Hub.
First, log in to Docker Hub using the docker login command, providing your Docker Hub credentials.
shell docker login
Now, push the image to Docker Hub (replace my-dockerhub-username with your Docker Hub username):
shell docker push my-dockerhub-username/my-go-app:v1
Your Go application is now containerized and ready to be deployed on Kubernetes.
3. Kubernetes Basics
Before diving into deploying our Go application on Kubernetes, let’s understand some key Kubernetes concepts.
3.1. Understanding Kubernetes Components
Kubernetes has several essential components:
- Nodes: These are the worker machines where your containers run.
- Pods: The smallest deployable units in Kubernetes, containing one or more containers.
- Deployment: A higher-level abstraction that manages sets of identical pods, ensuring a specified number of replicas are running.
- Service: An abstraction that exposes a set of pods as a network service.
- Ingress: Manages external access to services, typically HTTP.
- ConfigMap and Secret: Store configuration data separately from application code.
- Namespace: A way to divide cluster resources into multiple virtual clusters.
3.2. Creating a Kubernetes Cluster
In our earlier steps, we set up Minikube, a single-node Kubernetes cluster for local development. In a production environment, you would typically use a managed Kubernetes service such as Google Kubernetes Engine (GKE), Amazon EKS, or Azure Kubernetes Service (AKS).
3.3. Deploying Your First Pod
Let’s start by deploying a simple pod on our Kubernetes cluster to get a feel for how it works.
Create a file named pod.yaml with the following content:
yaml apiVersion: v1 kind: Pod metadata: name: my-pod spec: containers: - name: my-container image: my-dockerhub-username/my-go-app:v1
This YAML defines a pod named my-pod with a single container using the Docker image we built earlier. Replace my-dockerhub-username with your Docker Hub username and adjust the image tag if needed.
Apply the pod configuration to your cluster:
shell kubectl apply -f pod.yaml
Check the status of your pod:
shell kubectl get pods
You should see your pod in the “Running” state.
With this basic knowledge of Kubernetes, we’re ready to move on to deploying our Go application.
4. Deploying a Go Application on Kubernetes
Now that we’ve containerized our Go application and understand the basics of Kubernetes, let’s deploy our Go application on Kubernetes.
4.1. Creating Kubernetes Deployment YAML
We’ll create a Kubernetes Deployment to manage our application. Create a file named deployment.yaml with the following content:
yaml apiVersion: apps/v1 kind: Deployment metadata: name: my-go-app spec: replicas: 3 selector: matchLabels: app: my-go-app template: metadata: labels: app: my-go-app spec: containers: - name: my-go-app image: my-dockerhub-username/my-go-app:v1 ports: - containerPort: 8080
This YAML defines a Deployment named my-go-app with three replicas. It specifies the container image we built earlier and exposes port 8080.
Apply the deployment configuration:
shell kubectl apply -f deployment.yaml
4.2. Exposing Your Application to the Internet
To make your application accessible from the internet, you can create a Kubernetes Service of type LoadBalancer. Create a file named service.yaml with the following content:
yaml apiVersion: v1 kind: Service metadata: name: my-go-app-service spec: selector: app: my-go-app ports: - protocol: TCP port: 80 targetPort: 8080 type: LoadBalancer
Apply the service configuration:
shell kubectl apply -f service.yaml
To access your application, find the external IP address of the LoadBalancer service:
shell kubectl get svc my-go-app-service
Open a web browser and navigate to the external IP address. You should see your Go application running on Kubernetes!
5. Scaling and Managing Your Application
Kubernetes makes it easy to scale and manage your Go application.
5.1. Scaling Your Deployment
To scale your deployment, you can use the kubectl scale command. For example, to scale your deployment to five replicas, run:
shell kubectl scale deployment my-go-app --replicas=5
Kubernetes will automatically create or remove pods to match the desired replica count.
5.2. Updating Your Application
When you make changes to your Go application’s code, you can update the Docker image and apply the changes to your Kubernetes deployment. Here are the steps:
- Build a new Docker image with the updated code and increment the version tag (e.g., v2).
- Push the new image to your container registry.
- Update the image in your deployment.yaml file to use the new version.
- Apply the updated deployment configuration:
shell kubectl apply -f deployment.yaml
Kubernetes will perform a rolling update, ensuring zero downtime.
5.3. Monitoring and Logging
Kubernetes provides various tools and integrations for monitoring and logging your applications, such as Prometheus, Grafana, and ELK Stack (Elasticsearch, Logstash, and Kibana). Implementing these tools can help you gain insights into your Go application’s performance and troubleshoot issues.
6. Best Practices
To ensure your Go application runs smoothly on Kubernetes, consider implementing the following best practices:
6.1. Secrets Management
Avoid hardcoding sensitive information like API keys or database passwords in your application code. Instead, use Kubernetes Secrets to securely store and manage such information.
6.2. Configuring Resource Limits
Specify resource limits and requests for your containers to ensure they don’t consume too many resources or get starved of resources. This helps with resource management and ensures consistent performance.
6.3. Implementing Health Checks
Set up liveness and readiness probes for your containers. Liveness probes determine if a container is healthy and should be restarted, while readiness probes indicate when a container is ready to accept traffic. These probes enhance the reliability of your application.
Conclusion
In this guide, we’ve explored the process of deploying Go applications on Kubernetes, from setting up your development environment to running a production-ready application. Kubernetes offers a powerful platform for container orchestration, allowing you to scale and manage your Go applications with ease.
As you continue to work with Kubernetes and Go, you’ll discover even more ways to optimize your application’s deployment, monitoring, and management. With these tools and best practices at your disposal, you’re well on your way to building robust, scalable, and resilient Go applications in a Kubernetes environment. Happy coding!
Table of Contents
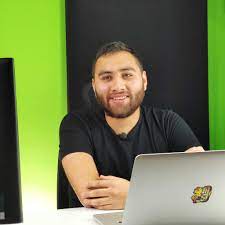
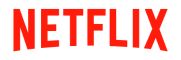