Secure Coding in Go: Best Practices for Building Robust and Safe Applications
Building software applications can be a daunting task, and the complexity can multiply when the goal is to ensure these applications are secure. This is where Golang, also known as Go, shines as a popular language for building secure, high-performance applications. Recognized for its simplicity and robust standard library, Go has become a preferred language for many software engineers and system administrators. Indeed, the growing demand to hire Golang developers is testament to its rising popularity.
In this blog post, we’ll unveil the best practices for secure coding in Go. We’ll journey from input validation to error handling, from mastering concurrency control to employing secure cryptography, providing you with a comprehensive roadmap to fortify your Go applications. Whether you’re looking to hire Golang developers or you’re a developer keen on upskilling, this guide will illuminate a variety of strategies to make your Go applications as secure as possible.
1. Input Validation
One of the most common security vulnerabilities is unvalidated or improperly validated input. This can lead to SQL Injection, Cross-Site Scripting (XSS), and other security issues.
In Go, input validation should be done at the point where user input is received. Let’s consider an example where we use the `net/http` package to handle a simple POST request:
```go package main import ( "net/http" "fmt" "io/ioutil" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { if r.Method != http.MethodPost { http.Error(w, "Invalid request method", http.StatusMethodNotAllowed) return } body, err := ioutil.ReadAll(r.Body) if err != nil { http.Error(w, "Error reading request body", http.StatusInternalServerError) return } fmt.Fprintf(w, "Received: %s\n", body) }) http.ListenAndServe(":8080", nil) } ```
In this code, we handle a POST request and read the body. However, there’s no input validation performed on the `body`. To rectify this, we can use Go’s `regexp` package for regular expressions, and implement input validation:
```go import ( "net/http" "fmt" "io/ioutil" "regexp" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { // existing code here... bodyStr := string(body) match, _ := regexp.MatchString("^[a-zA-Z0-9 ]*$", bodyStr) if !match { http.Error(w, "Invalid input", http.StatusBadRequest) return } fmt.Fprintf(w, "Received: %s\n", bodyStr) }) http.ListenAndServe(":8080", nil) } ```
In this improved code, we convert the `body` to a string and check if it matches the regular expression `^[a-zA-Z0-9 ]*$`, which only allows alphanumeric characters and spaces.
2. Error Handling
Proper error handling is paramount in building robust applications. In Go, the idiomatic way to handle errors is not to ignore them but to deal with them explicitly in your code.
Let’s consider an example where we try to open a file:
```go package main import ( "fmt" "os" ) func main() { file, _ := os.Open("/tmp/dat") defer file.Close() fmt.Println("Opened the file successfully") } ```
In the above code, we’re ignoring the error returned by `os.Open()`. However, if there’s any error while opening the file (e.g., file doesn’t exist, permission issues), our program will panic. The correct approach would be to handle the error:
```go package main import ( "fmt" "os" ) func main() { file, err := os.Open("/tmp/dat") if err != nil { fmt.Println("Failed to open the file:", err) return } defer file.Close() fmt.Println("Opened the file successfully") } ```
In this code, we check if `err` is `nil`. If it’s not, there was an error, and we log the error and return from the function.
3. Concurrency Control
Go is famous for its simple and efficient handling of concurrency with goroutines and channels. However, one should be careful to avoid common concurrency issues like race conditions and deadlocks.
A common way to avoid race conditions in Go is by using mutexes from the `sync` package:
```go package main import ( "fmt" "sync" ) func main() { var count int var lock sync.Mutex increment := func() { lock.Lock() defer lock.Unlock() count++ fmt.Printf("Incrementing: %d\n", count) } decrement := func() { lock.Lock() defer lock.Unlock() count-- fmt.Printf("Decrementing: %d\n", count) } var arithmetic sync.WaitGroup for i := 0; i <= 5; i++ { arithmetic.Add(1) go func() { defer arithmetic.Done() increment() }() } for i := 0; i <= 5; i++ { arithmetic.Add(1) go func() { defer arithmetic.Done() decrement() }() } arithmetic.Wait() fmt.Println("Arithmetic complete.") } ```
Here, we use a `sync.Mutex` to ensure that only one goroutine can access the `count` variable at a time, preventing race conditions.
4. Secure Cryptography
For creating secure applications, it’s crucial to use secure and proven cryptographic libraries instead of creating your own. Go’s standard library includes packages like `crypto/tls`, `crypto/hmac`, and `crypto/aes`, which you should use for encryption and other cryptographic operations.
Let’s consider an example of secure communication with a server using `crypto/tls`:
```go package main import ( "crypto/tls" "fmt" "net/http" ) func main() { tr := &http.Transport{ TLSClientConfig: &tls.Config{InsecureSkipVerify: false}, } client := &http.Client{Transport: tr} _, err := client.Get("https://secure.example.com") if err != nil { fmt.Println("Failed to connect:", err) return } fmt.Println("Connected successfully") } ```
In this code, we use `InsecureSkipVerify: false` to ensure that the client verifies the server’s certificate before establishing a connection.
Conclusion
Building secure applications in Go isn’t merely about possessing a handful of security tips or tricks. Instead, it necessitates the adoption of a robust and secure coding philosophy, a philosophy that any competent professional you hire, Golang developers included, must espouse. This approach encompasses everything from input validation to error handling, from concurrency control to cryptography.
By effectively using Go’s standard library and adhering to these best practices, you are not only optimizing performance but also reinforcing security. Hence, whether you’re looking to hire Golang developers or enhance your own coding skills, remembering these principles will guide you in building applications that are both robust and secure.
Table of Contents
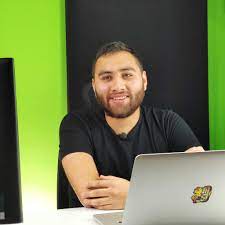
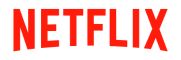