What is the purpose of the blank identifier (_) in Go?
In Go, the blank identifier, represented by an underscore (_), serves as a placeholder for values that are intentionally ignored or unused. It allows developers to indicate that a particular value returned by a function or assigned to a variable is not needed in the current context.
The blank identifier is particularly useful in scenarios where a function returns multiple values, but only a subset of those values is relevant to the current operation. Instead of explicitly declaring variables for all returned values, you can use the blank identifier to discard values that are not needed, improving code clarity and conciseness.
For example, consider a function that returns a result and an error:
go func compute() (int, error) { // Perform computation } func main() { result, _ := compute() // Ignore the error using the blank identifier fmt.Println(result) }
In this example, we use the blank identifier to discard the error returned by the compute function because we’re not interested in handling errors in the main function. This allows us to focus on the primary task of printing the result without cluttering the code with error handling logic that is unnecessary in this context.
The blank identifier is also commonly used in variable declarations and assignments to indicate that a variable is intentionally unused. This prevents compiler errors or warnings about unused variables while still allowing the code to compile successfully.
The blank identifier is a powerful and idiomatic feature of Go that promotes readability, expressiveness, and simplicity in code. It provides a clear and concise way to handle situations where certain values are irrelevant or intentionally ignored, improving the maintainability and clarity of Go programs.
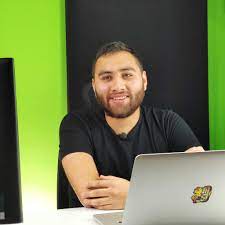
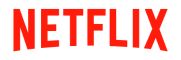