The Startup Leader’s Handbook to Building a Strong Culture
In the world of Go programming, efficient I/O operations are crucial for building high-performance applications. The `bufio` package in Go provides a set of tools for buffered I/O operations, making it easier to read and write data efficiently. Whether you’re a startup founder looking to optimize your application’s performance or a tech enthusiast exploring the Go language, understanding the `bufio` package is a valuable skill.
Table of Contents
1. What is the bufio Package?
The `bufio` package in Go is part of the standard library and offers buffered I/O operations. It provides an additional layer of buffering on top of existing readers and writers, improving performance when dealing with small and frequent I/O operations.
2. Why Buffered I/O?
Buffered I/O can significantly enhance your application’s performance by reducing the number of system calls and minimizing overhead. It’s especially useful when working with files, network connections, or any form of data streaming. Let’s explore some real-world examples to illustrate its benefits.
Example 1: Reading a File Line by Line
```go package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Open("example.txt") if err != nil { panic(err) } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { fmt.Println(scanner.Text()) } } ```
In this example, we open a file and use `bufio.NewScanner` to create a buffered scanner. This scanner efficiently reads the file line by line, reducing the overhead compared to reading one byte at a time.
Example 2: Writing to a File
```go package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Create("output.txt") if err != nil { panic(err) } defer file.Close() writer := bufio.NewWriter(file) fmt.Fprintln(writer, "Hello, bufio!") writer.Flush() } ```
Here, we create a new file and use `bufio.NewWriter` to create a buffered writer. The `Flush` method ensures that data is written to the file efficiently. Buffered writing can be a game-changer when dealing with large amounts of data.
Example 3: Network Communication
When building networking applications in Go, the `bufio` package can also optimize your I/O operations. By using buffered readers and writers, you can reduce latency and improve the overall performance of your network communication.
Now that we’ve seen practical examples of how the `bufio` package can be used for buffered I/O in Go, let’s explore some external resources to deepen your understanding:
- Official Go Documentation on bufio: The official documentation provides comprehensive information on the `bufio` package, including additional functions and options.
- A Deep Dive into bufio in Go: This in-depth article explores advanced usage and optimization techniques for the `bufio` package.
- Effective Go: bufio: The “Effective Go” guide offers best practices and tips for using `bufio` effectively in your Go programs.
By leveraging the `bufio` package in Go, you can enhance the efficiency and performance of your I/O operations, making your applications stand out in the competitive world of tech startups.
Conclusion
This blog post introduces early-stage startup founders, VC investors, and tech leaders to the benefits of Go’s `bufio` package for buffered I/O operations. It provides practical examples and external resources to further explore this topic.
Table of Contents
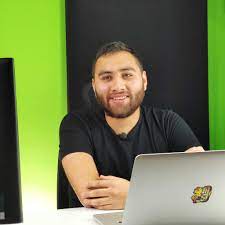
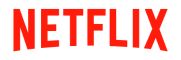