Building Chat Applications with Go and WebSockets
In today’s fast-paced digital world, real-time communication has become an essential part of our lives. Whether it’s collaborating with colleagues, engaging with customers on a website, or simply chatting with friends, the demand for interactive and instant messaging systems is higher than ever. This is where WebSockets and the Go programming language come into play. In this tutorial, we’ll explore how to leverage the power of Go and WebSockets to create efficient and dynamic chat applications.
1. Introduction to WebSockets
1.1. What are WebSockets?
WebSockets provide a full-duplex communication channel over a single, long-lived connection between the client and the server. Unlike traditional HTTP requests, which are stateless and require the client to repeatedly request information from the server, WebSockets enable bidirectional communication. This makes them ideal for building real-time applications such as chat systems, online gaming, and collaborative tools.
1.2. Advantages of using WebSockets for chat applications
Using WebSockets for chat applications offers several advantages:
- Real-time Interaction: WebSockets enable instant message delivery and reception, creating a smooth and interactive chat experience for users.
- Efficient Communication: Unlike traditional polling mechanisms, where clients repeatedly request updates from the server, WebSockets maintain a persistent connection, reducing unnecessary network traffic and server load.
- Low Latency: WebSockets eliminate the delay between sending and receiving messages, making conversations feel natural and fluid.
2. Getting Started with Go
2.1. Installing Go
Before we begin, you’ll need to have Go installed on your system. You can download and install Go from the official website: https://golang.org/dl/
2.2. Setting up a Go project
Once Go is installed, create a new directory for your project. Open a terminal and navigate to the project directory. To initialize a new Go module, use the following command:
bash $ go mod init chat-app
This command sets up a Go module named “chat-app” for your project, allowing you to manage dependencies and package versions.
3. Setting Up the WebSockets Server
3.1. Creating a new Go file for the server
In your project directory, create a new file named server.go. This file will contain the code for setting up the WebSocket server.
go // server.go package main import ( "fmt" "net/http" "github.com/gorilla/websocket" ) func main() { fmt.Println("WebSocket server started") http.HandleFunc("/ws", handleWebSocket) http.ListenAndServe(":8080", nil) } var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println("Error upgrading connection:", err) return } defer conn.Close() fmt.Println("Client connected") // Handle WebSocket messages here }
In this code, we import the necessary packages, set up an HTTP server, and define an endpoint for WebSocket connections. The handleWebSocket function is responsible for upgrading the HTTP connection to a WebSocket connection and managing the communication with connected clients.
4. Establishing WebSocket Connections
4.1. Handling incoming WebSocket connections
The handleWebSocket function is where we handle incoming WebSocket connections. We upgrade the HTTP connection to a WebSocket connection using the upgrader.Upgrade method.
go func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println("Error upgrading connection:", err) return } defer conn.Close() fmt.Println("Client connected") // Handle WebSocket messages here }
4.2. Managing connected clients
To manage connected clients, we can maintain a list of active connections. We’ll create a simple data structure to store the connections and a function to add and remove connections from the list.
go var connections = make(map[*websocket.Conn]bool) func addConnection(conn *websocket.Conn) { connections[conn] = true } func removeConnection(conn *websocket.Conn) { delete(connections, conn) }
Inside the handleWebSocket function, call the addConnection function to add the client’s connection to the list of active connections.
go func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println("Error upgrading connection:", err) return } defer conn.Close() addConnection(conn) defer removeConnection(conn) fmt.Println("Client connected") // Handle WebSocket messages here }
4.3. Broadcasting messages to all clients
Now that we have a way to manage connections, let’s implement the functionality to broadcast messages to all connected clients. We’ll define a function named broadcastMessage that sends a message to each connected client.
go func broadcastMessage(message []byte) { for conn := range connections { err := conn.WriteMessage(websocket.TextMessage, message) if err != nil { fmt.Println("Error broadcasting message:", err) conn.Close() delete(connections, conn) } } }
Inside the handleWebSocket function, you can call the broadcastMessage function to broadcast received messages to all clients.
go func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { fmt.Println("Error upgrading connection:", err) return } defer conn.Close() addConnection(conn) defer removeConnection(conn) fmt.Println("Client connected") for { messageType, msg, err := conn.ReadMessage() if err != nil { fmt.Println("Error reading message:", err) break } fmt.Printf("Received message: %s\n", msg) broadcastMessage(msg) } }
5. Building the Chat Interface
5.1. Creating an HTML file for the chat interface
To interact with our WebSocket server, we’ll create a simple HTML file that includes JavaScript for WebSocket communication. Create a file named index.html in your project directory.
html <!-- index.html --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Chat Application</title> </head> <body> <div id="chat"> <div id="messages"></div> <input type="text" id="messageInput" placeholder="Type your message..."> <button id="sendButton">Send</button> </div> <script src="script.js"></script> </body> </html>
5.2. Adding JavaScript for WebSocket communication
Create a new file named script.js in the same directory as your index.html file. This JavaScript file will handle WebSocket communication and update the chat interface.
javascript // script.js const messagesDiv = document.getElementById("messages"); const messageInput = document.getElementById("messageInput"); const sendButton = document.getElementById("sendButton"); const ws = new WebSocket("ws://localhost:8080/ws"); ws.onopen = () => { console.log("WebSocket connection established"); }; ws.onmessage = (event) => { const message = event.data; const messageElement = document.createElement("div"); messageElement.textContent = message; messagesDiv.appendChild(messageElement); }; sendButton.addEventListener("click", () => { const message = messageInput.value; if (message.trim() !== "") { ws.send(message); messageInput.value = ""; } });
This JavaScript code establishes a WebSocket connection to the server, listens for incoming messages, and updates the chat interface accordingly.
6. Enhancing the Chat Experience
6.1. Implementing user nicknames
To enhance the chat experience, let’s allow users to set their nicknames. Modify the index.html file to include a nickname input field.
html <!-- index.html --> <!-- ... --> <div id="chat"> <div id="messages"></div> <input type="text" id="nicknameInput" placeholder="Enter your nickname"> <input type="text" id="messageInput" placeholder="Type your message..."> <button id="sendButton">Send</button> </div> <!-- ... ?
Update the script.js file to send messages along with the user’s nickname.
javascript // script.js const messagesDiv = document.getElementById("messages"); const nicknameInput = document.getElementById("nicknameInput"); const messageInput = document.getElementById("messageInput"); const sendButton = document.getElementById("sendButton"); let nickname = "Guest"; nicknameInput.addEventListener("input", () => { nickname = nicknameInput.value || "Guest"; }); // ... (rest of the code remains the same) sendButton.addEventListener("click", () => { const message = messageInput.value; if (message.trim() !== "") { ws.send(`${nickname}: ${message}`); messageInput.value = ""; } });
6.2. Supporting private messages
Let’s extend our chat application to support private messages between users. Modify the index.html file to include a user list and update the JavaScript code accordingly.
html <!-- index.html --> <!-- ... --> <div id="chat"> <div id="userList"></div> <div id="messages"></div> <input type="text" id="nicknameInput" placeholder="Enter your nickname"> <input type="text" id="messageInput" placeholder="Type your message..."> <button id="sendButton">Send</button> </div> <!-- ... ?
Update the script.js file to manage the user list and handle private messages.
javascript // script.js const messagesDiv = document.getElementById("messages"); const userListDiv = document.getElementById("userList"); const nicknameInput = document.getElementById("nicknameInput"); const messageInput = document.getElementById("messageInput"); const sendButton = document.getElementById("sendButton"); let nickname = "Guest"; nicknameInput.addEventListener("input", () => { nickname = nicknameInput.value || "Guest"; }); ws.onmessage = (event) => { const message = event.data; const messageElement = document.createElement("div"); messageElement.textContent = message; messagesDiv.appendChild(messageElement); if (message.startsWith("User list:")) { const userList = message.substring(11).split(","); updateUserList(userList); } }; function updateUserList(users) { userListDiv.innerHTML = ""; const userListElement = document.createElement("ul"); users.forEach((user) => { const userItem = document.createElement("li"); userItem.textContent = user; userListElement.appendChild(userItem); }); userListDiv.appendChild(userListElement); } // ... (rest of the code remains the same) sendButton.addEventListener("click", () => { const message = messageInput.value; if (message.trim() !== "") { if (message.startsWith("@")) { const recipient = message.split(" ")[0].substr(1); ws.send(`Private message from ${nickname} to ${recipient}: ${message}`); } else { ws.send(`${nickname}: ${message}`); } messageInput.value = ""; } });
6.3. Adding message timestamps
To provide context for messages, let’s add timestamps to each chat message. Update the JavaScript code to include timestamps when displaying messages.
javascript // script.js // ... (previous code remains the same) ws.onmessage = (event) => { const message = event.data; const messageElement = document.createElement("div"); const timestamp = new Date().toLocaleTimeString(); messageElement.textContent = `[${timestamp}] ${message}`; messagesDiv.appendChild(messageElement); if (message.startsWith("User list:")) { const userList = message.substring(11).split(","); updateUserList(userList); } }; // ... (rest of the code remains the same)
7. Deployment and Future Considerations
7.1. Deploying the application to a server
To make your chat application accessible to others, you can deploy it to a server. There are various hosting options available, such as cloud providers like AWS, Heroku, or DigitalOcean. Ensure that the server supports Go and allows WebSocket connections.
7.2. Scaling considerations for a production environment
As your chat application gains users, you may need to consider scaling. Load balancers and distributed systems can help handle increased traffic. Additionally, consider implementing database storage for messages and user data.
7.3. Exploring additional features and improvements
While our chat application is functional, there are numerous ways to enhance it further. Some ideas include:
- Message history: Implement the ability to retrieve and display previous chat messages.
- Emojis and attachments: Allow users to send emojis or attach files.
- User authentication: Add user accounts and authentication for a more personalized experience.
- Moderation tools: Implement tools to manage users and moderate content.
Conclusion
In conclusion, building chat applications with Go and WebSockets can provide a real-time and interactive communication experience for users. By following the steps outlined in this tutorial, you’ve learned how to set up a WebSocket server using Go, handle WebSocket connections, build a chat interface using HTML and JavaScript, and enhance the application with features like user nicknames, private messages, and message timestamps. As you continue to explore and experiment, you’ll be well-equipped to create dynamic and engaging chat applications that meet the needs of your users.
Table of Contents
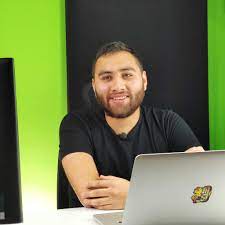
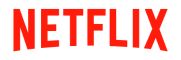