Unleashing the Power of Go: A Guide to Building High-Performance Web Applications
Web development is a critical component of today’s tech industry, with numerous languages and frameworks at a developer’s disposal. Amidst these, Google’s Go (or Golang) has risen in popularity, leading to an increasing demand to hire Golang developers. This is due to Go’s simplicity, robust standard library, and high-performance characteristics. In this post, we’ll explore why hiring Golang developers could be a strategic move for your business as we delve into how to use Go for building high-performance web applications.
Introduction to Go
Go is a statically typed, compiled language known for its simplicity, efficiency, and strong support for concurrent programming. It was developed by Robert Griesemer, Rob Pike, and Ken Thompson at Google in 2007 to address the shortcomings of other languages they were using at the time.
Go’s focus on performance means that it is particularly well-suited to building web servers, a fact that makes the decision to hire Golang developers a strategic one for businesses. It has excellent support for handling multiple requests concurrently, thanks to its goroutines and channels. Moreover, Go’s standard library includes packages for building HTTP servers and handling web requests, reducing reliance on third-party libraries or frameworks. This inherent robustness of Go serves as a compelling reason to hire Golang developers for your web-based projects.
Starting a Simple Web Server
To begin with, let’s start with a simple Go web server.
```go package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, %s", r.URL.Path[1:]) } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } ```
This script starts a server that listens on port 8080 and responds with a simple greeting that includes the request’s URL path. The `http.HandleFunc` and `http.ListenAndServe` functions come from Go’s `net/http` package. These functions are all it takes to start a basic web server in Go.
Using Goroutines for Concurrency
One of Go’s most powerful features is its support for concurrent programming through goroutines and channels. Goroutines are like lightweight threads, but they are managed by the Go runtime, not the operating system. This means you can easily spin up thousands of goroutines without significantly impacting performance.
Let’s see an example of a concurrent HTTP server that processes incoming requests in separate goroutines:
```go package main import ( "fmt" "net/http" "time" ) func handler(w http.ResponseWriter, r *http.Request) { time.Sleep(2 * time.Second) // simulate a long process fmt.Fprintf(w, "Hello, %s", r.URL.Path[1:]) } func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { go handler(w, r) }) http.ListenAndServe(":8080", nil) } ```
In this example, the server simulates a long process by sleeping for 2 seconds before responding. By calling the `handler` function in a separate goroutine, the server can start processing a new request as soon as it comes in, without waiting for the previous one to finish. This greatly increases the server’s throughput and responsiveness.
Building a RESTful API with Go
So far, we’ve seen how to handle simple HTTP requests. But for more complex applications, we need to be able to handle different types of requests (GET, POST, PUT, DELETE) and work with data in various formats.
In the following example, we’ll see how to build a simple RESTful API in Go using just the standard library. We’ll use an in-memory data store to keep things simple, but in a real-world application, you would probably use a database.
```go package main import ( "encoding/json" "net/http" "strconv" "sync" ) type Item struct { ID int `json:"id"` Name string `json:"name"` Price int `json:"price"` } var ( store = make(map[int]Item) mutex = &sync.RWMutex{} ) func getItems(w http.ResponseWriter, r *http.Request) { mutex.RLock() items := make([]Item, 0, len(store)) for _, item := range store { items = append(items, item) } mutex.RUnlock() json.NewEncoder(w).Encode(items) } func getItem(w http.ResponseWriter, r *http.Request) { id, _ := strconv.Atoi(r.URL.Path[len("/items/"):]) mutex.RLock() item, ok := store[id] mutex.RUnlock() if !ok { http.NotFound(w, r) return } json.NewEncoder(w).Encode(item) } func addItem(w http.ResponseWriter, r *http.Request) { var item Item json.NewDecoder(r.Body).Decode(&item) mutex.Lock() id := len(store) + 1 item.ID = id store[id] = item mutex.Unlock() json.NewEncoder(w).Encode(item) } func main() { http.HandleFunc("/items", getItems) http.HandleFunc("/items/", getItem) http.HandleFunc("/items/add", addItem) http.ListenAndServe(":8080", nil) } ```
This server provides three endpoints:
– `/items` returns a list of all items (GET)
– `/items/{id}` returns the item with the given id (GET)
– `/items/add` adds a new item (POST)
By taking advantage of Go’s standard library, we’ve managed to create a simple but functional RESTful API.
Wrapping Up
These examples have hopefully demonstrated how Go can be an effective language for building web applications. The combination of its simplicity, robust standard library, and high-performance characteristics makes it a great choice for many use cases. This is why businesses are increasingly looking to hire Golang developers.
Whether you’re building a simple web server or a complex RESTful API, Go offers the tools and performance you need to build efficient, high-performance web applications. If you haven’t already, consider hiring Golang developers for your next web development project. You might find their expertise in Go is exactly what your project needs to reach new heights of performance and efficiency.
Table of Contents
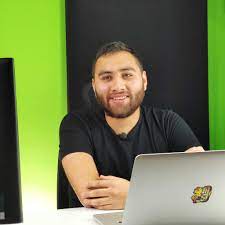
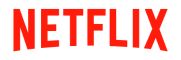