Building Secure Applications: A Comprehensive Guide to Safe and Robust Coding Practices in Go
As the world becomes more digitized, the demand for secure software development is increasingly paramount. This has led to a surge in demand to hire Golang developers, who are adept at leveraging languages like Go (also known as Golang). Go offers robust toolkits for writing secure, efficient, and scalable programs. While Go’s design inherently eliminates certain classes of security bugs, it doesn’t mean that Go programs are entirely exempt from security vulnerabilities. This blog post aims to introduce you to secure coding practices in Go, complete with practical examples. These practices are especially crucial for Golang developers to create safer code.
1. Input Validation
Input validation is a critical part of secure coding in any language. In Go, you might take input in many forms, such as command-line arguments, data from network clients, or user-supplied files. Here’s a basic example of input validation in Go:
```go import ( "fmt" "regexp" ) func validateInput(input string) bool { re := regexp.MustCompile(`^[a-zA-Z0-9]+ As the world becomes more digitized, the demand for secure software development is increasingly paramount. This has led to a surge in demand to hire Golang developers, who are adept at leveraging languages like Go (also known as Golang). Go offers robust toolkits for writing secure, efficient, and scalable programs. While Go's design inherently eliminates certain classes of security bugs, it doesn't mean that Go programs are entirely exempt from security vulnerabilities. This blog post aims to introduce you to secure coding practices in Go, complete with practical examples. These practices are especially crucial for Golang developers to create safer code.![]()
1. Input Validation
Input validation is a critical part of secure coding in any language. In Go, you might take input in many forms, such as command-line arguments, data from network clients, or user-supplied files. Here's a basic example of input validation in Go: ) return re.MatchString(input) } func main() { fmt.Println("Enter your username: ") var input string fmt.Scan(&input) if validateInput(input) { fmt.Println("Valid input") } else { fmt.Println("Invalid input. Only alphanumeric characters allowed.") } } ```
In this snippet, the `validateInput` function uses regular expressions to ensure the input only contains alphanumeric characters.
2. Error Handling
Go’s unique approach to error handling encourages developers to address errors directly. Since Go lacks exceptions, functions often return an error as the last return type. Here’s an example:
```go package main import ( "fmt" "os" ) func main() { f, err := os.Open("filename.ext") if err != nil { fmt.Println("Error opening file:", err) return } // Do something with the open *File f } ```
In this example, the error is explicitly checked and handled. Remember, ignoring errors can lead to unanticipated vulnerabilities.
3. Safe Concurrency
Go has built-in support for concurrent programming with Goroutines and channels. However, improper synchronization can lead to race conditions. To prevent this, you can use mutexes or channels for synchronization. Here’s an example using a mutex:
```go package main import ( "fmt" "sync" ) type SafeCounter struct { v map[string]int mux sync.Mutex } func (c *SafeCounter) Inc(key string) { c.mux.Lock() c.v[key]++ c.mux.Unlock() } func (c *SafeCounter) Value(key string) int { c.mux.Lock() defer c.mux.Unlock() return c.v[key] } func main() { c := SafeCounter{v: make(map[string]int)} var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { defer wg.Done() c.Inc("key") }() } wg.Wait() fmt.Println(c.Value("key")) } ```
In this example, a `SafeCounter` type is protected by a mutex, ensuring only one goroutine can access the `SafeCounter`‘s state at a time, preventing race conditions.
4. Securely Managing Secrets
Sensitive data such as API keys, passwords, and tokens should never be hardcoded into your Go code. This data should ideally be stored securely and retrieved when necessary. Here’s an example using environment variables:
```go package main import ( "fmt" "os" ) func main() { secret := os.Getenv("SECRET") fmt.Println("The secret is", secret) } ```
In this snippet, the `os.Getenv` function is used to fetch the value of an environment variable named “SECRET”.
5. Safe SQL Queries
SQL injection attacks can be prevented by using parameterized queries or prepared statements. Go’s `database/sql` package makes this easy:
```go package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@/dbname") if err != nil { log.Fatal(err) } defer db.Close() id := "1" rows, err := db.Query("SELECT * FROM users WHERE id = ?", id) if err != nil { log.Fatal(err) } defer rows.Close() // Handle rows here... } ```
This example shows a parameterized query to a MySQL database where user input is safely included in the query.
6. Avoid Data Races
Data races happen when two goroutines access the same variable concurrently, and at least one of the accesses is a write. You can use the `-race` flag with `go build`, `go run`, or `go test` to enable the race detector:
```bash go run -race mysrc.go ```
If the race detector finds a data race, it will report it and crash the program.
Conclusion
Secure coding in Go involves more than just understanding the language’s syntax. It requires a proactive approach to detect and eliminate potential vulnerabilities, which is a key skill when looking to hire Golang developers. From input validation to proper error handling, secure secret management, and concurrency control, these developers implement robust security measures in their coding practices. By adopting these secure coding practices, not only can you contribute to building a safer digital world, but you also improve your prospects if you’re looking to hire Golang developers for building secure applications.
Table of Contents
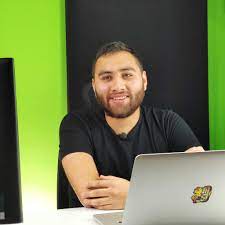
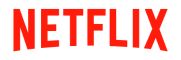