Building Web Crawlers with Go: Extracting Data from the Web
In today’s data-driven world, extracting information from the web is a crucial task for businesses, researchers, and developers. Web crawling, the process of navigating through websites and gathering data, is an essential technique for collecting valuable insights. In this tutorial, we will delve into building web crawlers using the Go programming language. With its efficiency and concurrency support, Go is an excellent choice for this task. Let’s explore how you can harness its power to scrape and extract data from the web.
1. Understanding Web Crawlers
1.1. What are Web Crawlers?
Web crawlers, also known as web spiders or web robots, are automated scripts that navigate through websites and extract information. They play a pivotal role in various applications, such as search engines, price comparison websites, and data mining. Web crawlers systematically browse web pages, follow links, and gather data for indexing, analysis, or storage.
1.2. Components of a Web Crawler:
- Downloader: Responsible for fetching web pages and their content.
- Parser: Processes the downloaded content and extracts relevant data.
- Storage: Stores the extracted data in a structured format for further use.
- Scheduler: Manages the order of URLs to be crawled and enforces politeness rules.
2. Getting Started with Go and Web Crawling
2.1. Setting Up Go:
Before diving into building web crawlers, ensure you have Go installed on your system. You can download and install it from the official Go website.
2.2. Installing Dependencies:
We’ll be using a third-party package called colly to build our web crawler. To install it, open your terminal and run the following command:
shell go get github.com/gocolly/colly/v2
2.3. Building Your First Web Crawler
Let’s start by building a simple web crawler that extracts the titles of articles from a news website.
2.3.1. Creating the Crawler:
go package main import ( "fmt" "log" "github.com/gocolly/colly/v2" ) func main() { c := colly.NewCollector() c.OnHTML("h2", func(e *colly.HTMLElement) { fmt.Println(e.Text) }) err := c.Visit("https://example-news-site.com") if err != nil { log.Fatal(err) } }
In this code, we import the colly package, create a new collector, and define an OnHTML callback function. This function is triggered whenever an HTML element with the <h2> tag is encountered on the page. We extract and print the text content of these elements, which typically represent article titles.
2.3.2. Running the Crawler:
Save the code in a file named main.go and execute it using the following command:
shell go run main.go
The crawler will visit the specified URL and output the titles of the articles.
3. Advanced Web Crawling Techniques
3.1. Handling Links:
Web crawlers navigate through websites by following links. Let’s enhance our crawler to extract article titles and URLs.
go package main import ( "fmt" "log" "strings" "github.com/gocolly/colly/v2" ) func main() { c := colly.NewCollector() c.OnHTML("h2", func(e *colly.HTMLElement) { title := e.Text link := e.ChildAttr("a", "href") if link != "" && strings.HasPrefix(link, "https://example-news-site.com") { fmt.Printf("Title: %s\nLink: %s\n\n", title, link) } }) err := c.Visit("https://example-news-site.com") if err != nil { log.Fatal(err) } }
In this version of the crawler, we extract both the article title and the corresponding URL by using the ChildAttr method. Additionally, we check if the link is within the same domain to avoid crawling external websites.
3.2. Concurrent Crawling:
Go’s concurrency support enables efficient web crawling. We can parallelize requests to multiple pages for faster data extraction.
go package main import ( "fmt" "log" "strings" "github.com/gocolly/colly/v2" ) func main() { c := colly.NewCollector() c.OnHTML("h2", func(e *colly.HTMLElement) { title := e.Text link := e.ChildAttr("a", "href") if link != "" && strings.HasPrefix(link, "https://example-news-site.com") { fmt.Printf("Title: %s\nLink: %s\n\n", title, link) } }) c.OnHTML("a[href]", func(e *colly.HTMLElement) { link := e.Attr("href") if strings.HasPrefix(link, "https://example-news-site.com") { c.Visit(e.Request.AbsoluteURL(link)) } }) err := c.Visit("https://example-news-site.com") if err != nil { log.Fatal(err) } }
In this version, we add a new callback to find and visit links within the same domain. This allows our crawler to traverse multiple pages concurrently, significantly speeding up the data extraction process.
4. Best Practices and Considerations
- Politeness: Respect the robots.txt file of websites to ensure you’re not crawling restricted content. Implement a delay between requests to avoid overloading servers.
- User-Agent: Set a user agent for your crawler to identify it to the web server. This helps maintain a positive relationship with the website and its administrators.
- Error Handling: Handle errors gracefully. Websites may change their structure, return errors, or be temporarily unavailable. Implement retry mechanisms and error logging.
- Data Storage: Store the extracted data in a structured format, such as a database or a CSV file, for further analysis and processing.
Conclusion
Web crawling is a powerful technique for extracting valuable data from the web. With Go’s efficiency and concurrency features, building web crawlers becomes an accessible task. In this tutorial, we’ve covered the basics of creating web crawlers using the colly package, advanced techniques like handling links and concurrent crawling, and best practices to ensure a smooth and respectful crawling process. As you continue to explore the world of web crawling, remember to stay ethical, adhere to website terms of use, and use this knowledge responsibly to unlock insights that can drive innovation and understanding. Happy crawling!
Table of Contents
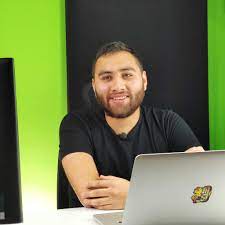
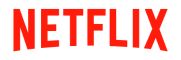