Go for Cloud Computing: Deploying Applications on Cloud Platforms
In today’s fast-paced digital landscape, businesses and developers are increasingly turning to cloud computing to meet their computing and infrastructure needs. Cloud computing offers scalability, flexibility, and cost-efficiency, making it an attractive option for deploying applications. Among the many programming languages used for cloud development, Go (also known as Golang) stands out as an excellent choice. In this comprehensive guide, we’ll delve into the world of cloud computing and explore how to deploy applications on cloud platforms using Go.
1. Why Cloud Computing?
1.1. The Need for Scalability
One of the primary reasons for embracing cloud computing is scalability. Traditional on-premises solutions often struggle to handle sudden spikes in traffic or resource demands. In contrast, cloud platforms provide the ability to scale resources up or down dynamically, ensuring that your application can handle any load. Whether you’re building a web app, a microservices-based system, or a data analytics platform, the cloud’s scalability can be a game-changer.
1.2. Cost-Efficiency
Cloud computing can also lead to significant cost savings. With on-demand resources, you only pay for what you use, eliminating the need for costly upfront investments in hardware and data centers. This pay-as-you-go model can be especially beneficial for startups and small businesses looking to minimize their initial capital expenditure.
1.3. Global Reach
Cloud providers have data centers strategically located around the world. This global reach enables your applications to be closer to your users, reducing latency and improving performance. Additionally, it simplifies the process of expanding your application’s presence in different regions as your user base grows.
1.4. Flexibility and Agility
Cloud platforms offer a wide range of services and tools that enable developers to build, deploy, and manage applications with ease. Whether you need to store data, run containers, or implement machine learning algorithms, the cloud has you covered. This flexibility and agility empower developers to focus on creating value for their users rather than managing infrastructure.
2. Choosing the Right Cloud Provider
When embarking on your cloud computing journey, selecting the right cloud provider is crucial. Some of the major cloud providers include Amazon Web Services (AWS), Microsoft Azure, Google Cloud Platform (GCP), and IBM Cloud. Each provider offers a unique set of services, pricing structures, and global data center locations. Here are some key factors to consider when choosing a cloud provider:
2.1. Service Offerings
Different cloud providers excel in different areas. AWS, for instance, is known for its extensive service catalog, including Amazon EC2 for virtual machines, Amazon S3 for object storage, and AWS Lambda for serverless computing. Azure is popular among enterprises and integrates well with Microsoft technologies, while GCP is known for its data analytics and machine learning capabilities. Evaluate your project’s requirements and choose a provider that aligns with your needs.
2.2. Pricing
Pricing structures can vary significantly between cloud providers. It’s essential to understand the pricing models, including on-demand, reserved instances, and spot instances. Consider your budget and usage patterns to determine which pricing option is the most cost-effective for your application.
2.3. Compliance and Security
Security and compliance are critical considerations, especially if you’re handling sensitive data. Ensure that your chosen cloud provider meets industry-specific compliance standards and offers robust security features, such as encryption, identity and access management (IAM), and network security.
2.4. Support and Documentation
The availability of documentation, support channels, and a thriving community can greatly facilitate your cloud development journey. Look for a provider that offers comprehensive documentation, active forums, and responsive customer support.
3. Getting Started with Go and Cloud Computing
Now that you’ve decided to embrace cloud computing, let’s dive into how you can leverage the Go programming language to build and deploy applications in the cloud. Go is renowned for its simplicity, efficiency, and strong support for concurrency, making it an excellent choice for developing cloud-native applications.
4. Setting Up Your Development Environment
Before you can start building cloud applications with Go, you’ll need to set up your development environment. Here are the essential tools and components you’ll need:
4.1. Go Programming Language
If you haven’t already, install the Go programming language on your system. You can download the latest version from the official Go website.
4.2. Code Editor
Choose a code editor or integrated development environment (IDE) that suits your preferences. Popular options include Visual Studio Code, GoLand, and Goland.
4.3. Cloud SDK
To interact with your chosen cloud provider’s services, you’ll need to install the respective cloud SDK. For example, if you’re using AWS, you can install the AWS Command Line Interface (CLI). Similarly, Azure offers the Azure CLI, and GCP provides the Cloud SDK.
4.4. Version Control
Use a version control system like Git to track changes in your codebase. Hosting your code on platforms like GitHub or GitLab can facilitate collaboration and code sharing.
5. Creating a Simple Go Application
Let’s start with a straightforward Go application to get a feel for the language and its capabilities. We’ll create a “Hello, Cloud” web server that listens on a port and responds with a friendly greeting when accessed.
go package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, Cloud!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
Save this code to a file named main.go. To run the application locally, open your terminal, navigate to the directory containing main.go, and execute:
bash go run main.go
You can access the application by opening a web browser and navigating to http://localhost:8080.
6. Containerization with Docker
Containerization is a popular approach for packaging applications and their dependencies into portable containers. Docker is the go-to tool for creating and managing containers. Let’s containerize our Go application:
6.1. Create a Dockerfile
In the same directory as your Go code, create a file named Dockerfile with the following contents:
Dockerfile # Use the official Go base image FROM golang:latest # Set the working directory inside the container WORKDIR /app # Copy the Go application code into the container COPY . . # Build the Go application RUN go build -o main . # Expose port 8080 for the application EXPOSE 8080 # Command to run the application CMD ["./main"]
6.2. Build the Docker Image
Open your terminal and navigate to the directory containing Dockerfile. Run the following command to build the Docker image:
bash docker build -t hello-cloud-app
6.3. Run the Container
Once the image is built, you can run a container from it using the following command:
bash docker run -p 8080:8080 hello-cloud-app
Your Go application is now running inside a Docker container and accessible at http://localhost:8080.
7. Deploying to the Cloud
Now that you have a containerized Go application, it’s time to deploy it to the cloud. We’ll focus on deploying to AWS, one of the leading cloud providers, using Amazon Elastic Container Service (ECS).
7.1. Create an Amazon ECS Cluster
Log in to your AWS account and navigate to the ECS dashboard. Create an ECS cluster, which is a logical grouping of container instances.
7.2. Configure AWS CLI
If you haven’t already, configure the AWS CLI with your access credentials by running:
bash aws configure
7.3. Push Docker Image to Amazon ECR
Amazon Elastic Container Registry (ECR) is a managed container image repository service. We’ll push our Docker image to ECR:
Create an ECR repository:
bash aws ecr create-repository --repository-name hello-cloud-app
Authenticate Docker to your ECR registry:
bash aws ecr get-login-password --region <your-region> | docker login --username AWS --password-stdin <your-account-id>.dkr.ecr.<your-region>.amazonaws.com
Tag your Docker image:
bash docker tag hello-cloud-app:latest <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/hello-cloud-app:latest
Push the image to ECR:
bash docker push <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/hello-cloud-app:latest
7.4. Create an ECS Task Definition
A task definition is a blueprint for running containers on ECS. Create a task-definition.json file with the following content:
json { "family": "hello-cloud-app", "containerDefinitions": [ { "name": "hello-cloud-app", "image": "<your-account-id>.dkr.ecr.<your-region>.amazonaws.com/hello-cloud-app:latest", "memory": 512, "cpu": 256, "essential": true, "portMappings": [ { "containerPort": 8080, "hostPort": 8080 } ] } ] }
7.5. Register the Task Definition
Register the task definition with ECS:
bash aws ecs register-task-definition --cli-input-json file://task-definition.json
7.6. Create an ECS Service
Finally, create an ECS service that uses your task definition:
bash aws ecs create-service --cluster <your-cluster-name> --service-name hello-cloud-service --task-definition hello-cloud-app --desired-count 1 --launch-type EC2
Congratulations! Your Go application is now running in an AWS ECS cluster, fully deployed and ready to serve users on the cloud.
Conclusion
In this guide, we’ve explored the benefits of cloud computing, discussed how to choose the right cloud provider, and demonstrated how to get started with Go and cloud-native development. We’ve created a simple Go application, containerized it with Docker, and deployed it to Amazon ECS. This is just the beginning of your cloud computing journey.
As you continue your exploration of cloud computing, remember to leverage the power of cloud services, automation, and best practices to build scalable, reliable, and cost-effective applications. Whether you’re building web apps, microservices, or data processing pipelines, Go and the cloud provide the tools and infrastructure to support your vision.
The cloud offers a world of possibilities. Embrace it, and let your imagination soar as you build the next generation of cloud-native applications with Go.
Start your cloud journey today and watch your applications soar to new heights in the cloud!
Table of Contents
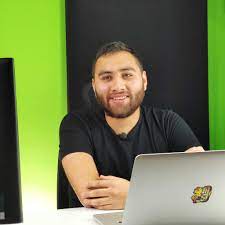
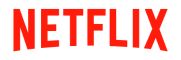