Building Command-Line Interfaces (CLIs) with Go: Enhancing User Experience
Command-Line Interfaces (CLIs) are essential tools for developers and power users alike. They provide a fast, efficient, and flexible way to interact with software applications without the need for graphical user interfaces. Building a well-designed CLI can significantly enhance the user experience, making your application more accessible and user-friendly.
In this blog post, we will explore how to build robust CLIs using the Go programming language. Go, also known as Golang, is a popular choice for developing CLI applications due to its simplicity, performance, and built-in support for concurrency. We will cover various aspects of building CLIs, including helpful libraries, best practices, and code samples to demonstrate each concept.
1. Getting Started with Go and CLI
Before diving into the specifics of building CLIs, let’s ensure you have Go installed on your system. You can download the latest version from the official Go website (golang.org) and follow the installation instructions for your operating system.
Once Go is set up, create a new project folder and open a terminal window to begin building your CLI application. Go follows the convention of keeping all code in a single workspace, which is the root of your project folder.
2. Understanding Flags and Arguments
Flags and arguments are fundamental components of any CLI. Flags are used to modify the behavior of a command, while arguments represent the actual data or input the command requires. Let’s create a simple example to illustrate the concept.
go package main import ( "flag" "fmt" ) func main() { // Define flags greeting := flag.String("greeting", "Hello", "Specify a greeting message") name := flag.String("name", "User", "Specify a name") // Parse flags flag.Parse() // Accessing flag values fmt.Printf("%s, %s!\n", *greeting, *name) }
In this example, we use the built-in flag package to define two flags, -greeting and -name, along with their default values and descriptions. After parsing the command-line arguments, we access the flag values using pointers to retrieve and display the output accordingly.
3. Building User-friendly Help Messages
Providing clear and concise help messages is crucial for any CLI application. Users need to understand the available commands, flags, and arguments to use the application effectively. Thankfully, Go’s flag package automatically generates help messages for us.
Let’s enhance the previous example with a help message:
go package main import ( "flag" "fmt" "os" ) func main() { // Define flags greeting := flag.String("greeting", "Hello", "Specify a greeting message") name := flag.String("name", "User", "Specify a name") // Custom usage message flag.Usage = func() { fmt.Fprintf(os.Stderr, "Usage: %s [flags]\n", os.Args[0]) flag.PrintDefaults() } // Parse flags flag.Parse() // Accessing flag values fmt.Printf("%s, %s!\n", *greeting, *name) }
Now, when the user runs the application with the -h or –help flag, they will see a detailed help message with the defined flags, their default values, and descriptions.
4. Handling User Input
In more complex CLI applications, you may need to handle user input beyond flags and arguments. For instance, you might want to prompt the user for additional information or provide a confirmation before executing a command.
Let’s see an example of prompting the user for input using the bufio package:
go package main import ( "bufio" "fmt" "os" "strings" ) func main() { reader := bufio.NewReader(os.Stdin) // Prompt the user for input fmt.Print("Enter your favorite programming language: ") language, _ := reader.ReadString('\n') // Trim the newline character language = strings.TrimSpace(language) fmt.Printf("You entered: %s\n", language) }
In this snippet, we utilize the bufio package to read input from the user and remove the trailing newline character for better formatting.
5. Structuring Commands
As your CLI application grows, organizing commands becomes essential to maintain clarity and improve user experience. Creating a modular structure for commands enables users to intuitively discover and use functionalities.
Let’s see how we can structure commands using subcommands:
go package main import ( "fmt" "os" "github.com/urfave/cli" ) func main() { app := cli.NewApp() app.Name = "MyApp" app.Usage = "A simple CLI application" app.Version = "1.0.0" // Define commands app.Commands = []*cli.Command{ { Name: "greet", Aliases: []string{"g"}, Usage: "Greet the user", Action: func(c *cli.Context) error { name := c.Args().First() fmt.Printf("Hello, %s!\n", name) return nil }, }, // Add more commands here... } err := app.Run(os.Args) if err != nil { fmt.Println(err) } }
In this example, we use the popular third-party library urfave/cli to structure our commands. The greet command is defined as a subcommand that takes the user’s name as an argument and greets them accordingly.
6. Adding Colors and Formatting
To make your CLI application visually appealing and highlight important information, you can add colors and formatting to the output. There are various Go libraries available for this purpose, such as github.com/fatih/color.
go package main import ( "fmt" "os" "github.com/fatih/color" "github.com/urfave/cli" ) func main() { app := cli.NewApp() app.Name = "ColorApp" app.Usage = "A CLI application with colors" app.Version = "1.0.0" // Define commands app.Commands = []*cli.Command{ { Name: "message", Aliases: []string{"m"}, Usage: "Display a colorful message", Action: func(c *cli.Context) error { green := color.New(color.FgGreen).SprintFunc() red := color.New(color.FgRed).SprintFunc() message := c.Args().First() if message == "success" { fmt.Printf("Success: %s\n", green(message)) } else if message == "error" { fmt.Printf("Error: %s\n", red(message)) } else { fmt.Println("Unknown message type.") } return nil }, }, // Add more commands here... } err := app.Run(os.Args) if err != nil { fmt.Println(err) } }
In this example, we use the github.com/fatih/color library to display colorful messages based on the user’s input.
Conclusion
Building Command-Line Interfaces with Go can significantly enhance the user experience and streamline interactions with your applications. We covered essential concepts like working with flags and arguments, providing user-friendly help messages, handling input, structuring commands, and adding colors and formatting.
As you continue to develop CLI applications with Go, explore additional libraries and best practices to make your applications even more powerful and user-friendly. Remember to document your commands thoroughly and design clear, intuitive interfaces to provide the best possible experience for your users. Happy coding!
Table of Contents
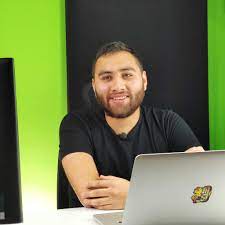
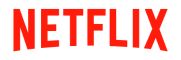