Creating Command-Line Tools with Go and Cobra
Command-line tools are a crucial part of a developer’s toolkit. They allow you to interact with your applications and perform various tasks efficiently, whether you’re a software engineer, system administrator, or DevOps practitioner. Go, also known as Golang, is a popular programming language known for its simplicity, performance, and strong support for building command-line tools. When it comes to building command-line applications in Go, Cobra is a fantastic framework that simplifies the development process and provides a consistent and user-friendly interface. In this blog post, we will dive into the world of command-line tool development using Go and Cobra.
1. Why Use Go for Command-Line Tools?
Before we delve into the specifics of using Cobra for building command-line tools, let’s briefly discuss why Go is an excellent choice for this purpose.
1.1. Performance
Go is a statically typed language that compiles to native machine code, making it incredibly fast and efficient. This performance is crucial for command-line tools, which often need to execute tasks quickly and with minimal overhead.
1.2. Cross-Platform Support
Go supports cross-compilation, allowing you to build command-line tools for multiple operating systems and architectures with ease. This means you can create tools that work on Windows, macOS, Linux, and more without major modifications.
1.3. Strong Standard Library
Go’s standard library includes packages for handling files, networking, and various other tasks commonly required in command-line applications. This means you can focus on building the core functionality of your tool without reinventing the wheel.
1.4. Simplicity
Go’s simple and concise syntax makes it easier to write and maintain code, reducing the learning curve for new developers and helping experienced developers be more productive.
1.5. Community and Ecosystem
The Go community is vibrant and active, with a wealth of libraries and resources available to help you develop command-line tools efficiently.
2. Getting Started with Cobra
Now that we’ve established why Go is a great choice for building command-line tools, let’s explore how to get started with Cobra.
2.1. What is Cobra?
Cobra is a popular and widely adopted Go library for creating powerful command-line applications. It provides a rich set of features to build complex, interactive, and user-friendly command-line tools. Some of Cobra’s key features include:
- Command Hierarchies: Cobra allows you to define nested commands and subcommands, making it easy to organize and structure your application’s functionality.
- Flag Support: It offers a robust flag and argument parsing system, enabling you to specify command-line options and arguments for your tools effortlessly.
- Automatic Help Generation: Cobra automatically generates help messages and usage information for your commands, improving the user experience.
- Bash Completion: You can integrate Bash completion for your commands, enhancing the usability of your tools.
2.2. Installing Cobra
To get started with Cobra, you first need to install it. Open your terminal and run the following command:
bash go get -u github.com/spf13/cobra/cobra
2.3. Creating a New Cobra Project
Let’s create a simple Cobra-based command-line tool to demonstrate its capabilities. Suppose we want to build a tool called “mycli” that can greet the user with a personalized message. Here are the steps to create this project:
Create a new Go module for your project:
bash go mod init mycli
Create a Go file for your Cobra-based application, typically named main.go. In this file, you’ll define your Cobra commands and their associated functionality.
go package main import ( "fmt" "github.com/spf13/cobra" "os" ) func main() { var rootCmd = &cobra.Command{Use: "mycli"} var name string var cmdGreet = &cobra.Command{ Use: "greet", Short: "Greet the user", Run: func(cmd *cobra.Command, args []string) { if name == "" { fmt.Println("Hello, World!") } else { fmt.Printf("Hello, %s!\n", name) } }, } cmdGreet.Flags().StringVarP(&name, "name", "n", "", "Specify a name to greet") rootCmd.AddCommand(cmdGreet) if err := rootCmd.Execute(); err != nil { fmt.Fprintln(os.Stderr, err) os.Exit(1) } }
In this code:
- We import the necessary packages, including “github.com/spf13/cobra” for Cobra functionality.
- We define the root command for our application as “mycli.”
- We create a subcommand called “greet” with a brief description.
- The Run function is where the actual logic for greeting the user is implemented. We use the name flag to determine whether to personalize the greeting.
- We define a flag named “name” with a shorthand “n” and a description.
- Finally, we add the “greet” subcommand to the root command and execute the Cobra application.
2.4. Building and Testing the Cobra Application
To build and test our newly created Cobra application, follow these steps:
Build the application:
bash go build -o mycli
Run the application without any arguments to see the default greeting:
bash ./mycli
You should see the output: “Hello, World!”
Run the application with a personalized greeting:
bash ./mycli greet --name Alice
You should see the output: “Hello, Alice!”
Congratulations! You’ve successfully created a simple command-line tool using Cobra.
3. Extending Your Command-Line Tool
Now that you have a basic understanding of how to create a command-line tool with Cobra, let’s explore some advanced features and best practices.
3.1. Adding More Commands
One of Cobra’s strengths is its ability to define complex command hierarchies. You can add more commands to your tool by following a similar pattern as we did with the “greet” command. For example, you could add commands to perform various tasks, such as creating, updating, or deleting resources.
3.2. Organizing Your Code
As your command-line tool grows, it’s essential to organize your code for maintainability. Consider structuring your project like this:
go mycli/ ??? cmd/ ? ??? cmd1.go ? ??? cmd2.go ? ??? ... ??? internal/ ? ??? pkg1/ ? ??? pkg2/ ? ??? ... ??? main.go ??? go.mod
- The cmd/ directory contains separate Go files for each command, helping keep your codebase modular.
- The internal/ directory can house shared internal packages or libraries.
- The go.mod file tracks your project’s dependencies.
3.4. Using Flags and Arguments
Cobra provides a flexible system for defining flags and arguments for your commands. You can specify flags with default values, required flags, and even use custom validation functions.
Here’s an example of how to define a required flag:
go cmd.Flags().StringVarP(&name, "name", "n", "", "Specify a name (required)") cmd.MarkFlagRequired("name")
3.5. Providing Help Information
Cobra automatically generates help messages for your commands. To provide additional information and examples, you can use the Long and Example fields in your command definitions.
go var cmdGreet = &cobra.Command{ Use: "greet", Short: "Greet the user", Long: `Greet the user with a personalized message. You can provide a name using the --name flag.`, Example: "mycli greet --name Alice", Run: func(cmd *cobra.Command, args []string) { // Greeting logic here }, }
3.6. Bash Completion
Enabling Bash completion for your command-line tool can greatly enhance the user experience. Cobra makes it easy to generate Bash completion scripts for your commands. You can enable this feature by adding the following line to your main.go file:
go rootCmd.CompletionOptions.DisableDefaultCmd = true
Then, generate the Bash completion script:
bash ./mycli completion bash > mycli_completion.sh
Source the completion script in your shell’s profile (e.g., .bashrc or .zshrc) to enable completion for your tool:
bash source mycli_completion.sh
3.7. Testing Your Commands
Writing tests for your Cobra commands is essential to ensure that they work correctly. You can use the standard Go testing framework to write unit tests for your commands and their associated functions.
go func TestGreetCmd(t *testing.T) { cmd := &cobra.Command{} cmd.SetArgs([]string{"greet", "--name", "Alice"}) // Capture the command's output var outBuf bytes.Buffer cmd.SetOutput(&outBuf) // Run the command if err := cmd.Execute(); err != nil { t.Errorf("Expected no error, but got %v", err) } // Verify the command's output expectedOutput := "Hello, Alice!\n" if outBuf.String() != expectedOutput { t.Errorf("Expected output %q, but got %q", expectedOutput, outBuf.String()) } }
4. Building and Distributing Your Tool
Once your command-line tool is complete, you can build it for distribution. You can cross-compile your tool for various platforms and architectures using the GOOS and GOARCH environment variables.
For example, to build your tool for Linux, macOS, and Windows, you can use the following commands:
bash # Linux GOOS=linux GOARCH=amd64 go build -o mycli-linux # macOS GOOS=darwin GOARCH=amd64 go build -o mycli-macos # Windows GOOS=windows GOARCH=amd64 go build -o mycli-windows.exe
Now, you have executable files for different platforms. You can distribute these files to your users or package them as part of your application.
Conclusion
Creating command-line tools with Go and Cobra is a powerful and efficient way to extend your software’s capabilities. Cobra provides a user-friendly framework for building complex command-line applications, making it easier to develop intuitive and robust tools. By following best practices and structuring your code effectively, you can create command-line tools that are not only functional but also a joy to use.
In this blog post, we covered the basics of getting started with Go and Cobra, including installation, project setup, and building a simple command-line tool. We also explored advanced features such as organizing your code, using flags and arguments, providing help information, enabling Bash completion, writing tests, and building and distributing your tool. Armed with this knowledge, you can embark on your journey to create powerful and user-friendly command-line tools in Go with confidence. Happy coding!
Note: Don’t forget to update the code and examples according to your specific project requirements and use cases. Cobra provides extensive customization options to tailor your command-line tool to your needs.
Table of Contents
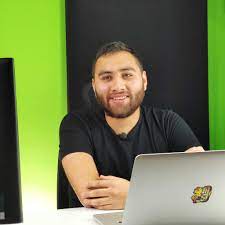
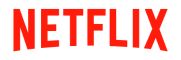