Concurrency Control in Go: Mutexes, Read-Write Locks, and Atomic Operations
Concurrency is a fundamental aspect of modern software development, especially in Go, a programming language renowned for its support of concurrent programming. But with great power comes great responsibility, and managing concurrent access to shared resources can be a tricky task. In this blog, we’ll delve into the world of concurrency control in Go, exploring the use of Mutexes, Read-Write Locks, and Atomic Operations to ensure safe and efficient concurrent programming.
1. Understanding the Need for Concurrency Control
Before we dive into the specifics of concurrency control in Go, let’s take a moment to understand why it’s necessary. In a multi-threaded or concurrent environment, multiple goroutines (Go’s lightweight threads) may access and modify shared data concurrently. This concurrent access can lead to data races, where two or more goroutines try to access or modify the same data simultaneously, resulting in unpredictable and often erroneous behavior.
Concurrency control mechanisms are essential to prevent data races and ensure the integrity of shared data. Go provides several tools for this purpose, with Mutexes, Read-Write Locks, and Atomic Operations being some of the most commonly used.
2. Mutexes: The Basics
A Mutex, short for mutual exclusion, is the most basic form of concurrency control in Go. It allows only one goroutine to access a critical section of code at a time. Here’s a simple example of how to use a Mutex:
go package main import ( "fmt" "sync" ) var ( counter int mu sync.Mutex ) func increment() { mu.Lock() defer mu.Unlock() counter++ } func main() { var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("Counter:", counter) }
In this example, we have a shared counter variable, which multiple goroutines increment concurrently. The sync.Mutex ensures that only one goroutine can execute the increment function at a time by locking and unlocking the Mutex before and after the critical section, respectively.
3. Read-Write Locks: Optimizing for Reading and Writing
While Mutexes work well when you need exclusive access to a resource, they can be overly restrictive in scenarios where multiple goroutines primarily want to read data concurrently but allow exclusive access for writing. This is where Read-Write Locks come into play.
Go provides the sync.RWMutex type for read-write locking. It allows multiple goroutines to read data simultaneously but ensures exclusive access for writing. Let’s see an example:
go package main import ( "fmt" "sync" "time" ) var ( data map[string]string mutex sync.RWMutex ) func init() { data = make(map[string]string) data["key1"] = "value1" data["key2"] = "value2" } func readData(key string) string { mutex.RLock() defer mutex.RUnlock() return data[key] } func writeData(key, value string) { mutex.Lock() defer mutex.Unlock() data[key] = value } func main() { var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go func() { defer wg.Done() fmt.Println("Read Data:", readData("key1")) }() } time.Sleep(time.Second) // Let the readers start first for i := 0; i < 2; i++ { wg.Add(1) go func() { defer wg.Done() writeData("key1", "newvalue1") fmt.Println("Write Data: key1 -> newvalue1") }() } wg.Wait() }
In this example, we use a sync.RWMutex to protect access to the data map. Multiple goroutines can read the data using RLock(), allowing for concurrent reading. When writing data, we use Lock() to ensure exclusive access, preventing any concurrent reads or writes.
4. Atomic Operations: Low-Level Concurrency Control
While Mutexes and Read-Write Locks provide high-level abstractions for concurrency control, Go also offers atomic operations for more fine-grained control over shared variables. These operations allow you to perform read-modify-write operations on variables atomically, without the need for locks.
Let’s look at an example of using atomic operations to increment a counter:
go package main import ( "fmt" "sync" "sync/atomic" ) var ( counter int32 wg sync.WaitGroup ) func increment() { atomic.AddInt32(&counter, 1) wg.Done() } func main() { const numGoroutines = 1000 wg.Add(numGoroutines) for i := 0; i < numGoroutines; i++ { go increment() } wg.Wait() fmt.Println("Counter:", counter) }
In this example, we use the atomic.AddInt32 function to atomically increment the counter variable. Since atomic operations are executed without locks, they are highly efficient and are suitable for scenarios where fine-grained control is needed with minimal overhead.
5. Choosing the Right Concurrency Control Mechanism
When it comes to choosing the right concurrency control mechanism in Go, you need to consider the specific requirements of your application. Here are some guidelines to help you make an informed decision:
5.1. Mutexes:
Use Mutexes when you need exclusive access to a resource.
They are suitable for scenarios where only one goroutine should access a critical section at a time.
5.2. Read-Write Locks:
Use Read-Write Locks when you have multiple goroutines that primarily read data and can tolerate concurrent reading.
They are beneficial when you want to optimize for read-heavy workloads.
5.3. Atomic Operations:
Use Atomic Operations when you need fine-grained control over shared variables.
They are efficient and work well for scenarios where you need to perform read-modify-write operations atomically.
5.4. Hybrid Approaches:
In some cases, a combination of these mechanisms may be beneficial. For example, you might use Mutexes to protect a critical section and atomic operations for fine-grained control within that section.
6. Pitfalls and Best Practices
When working with concurrency control mechanisms in Go, it’s crucial to be aware of potential pitfalls and follow best practices:
Pitfall 1: Deadlocks
Always ensure proper locking and unlocking of Mutexes or Read-Write Locks to prevent deadlocks, where goroutines are stuck waiting for a lock that will never be released.
Pitfall 2: Shared State
Minimize shared mutable state as much as possible. This reduces the complexity of concurrency control and the chances of encountering data races.
Pitfall 3: Avoiding Overuse
Don’t overuse locks. Excessive locking can lead to performance bottlenecks. Use locks only where necessary to avoid unnecessary contention.
Best Practice 1: Testing
Thoroughly test your concurrent code to identify and fix any race conditions or synchronization issues.
Best Practice 2: Documentation
Document the concurrency control mechanisms used in your code to make it clear to other developers and yourself why they are necessary.
Conclusion
Concurrency control is a crucial aspect of writing robust and efficient Go programs. By using Mutexes, Read-Write Locks, and Atomic Operations appropriately, you can ensure safe concurrent access to shared resources while optimizing for performance. Understanding the strengths and weaknesses of each mechanism is key to making informed decisions in your Go projects. So, choose your concurrency control wisely and embrace the power of Go’s concurrent programming capabilities.
Table of Contents
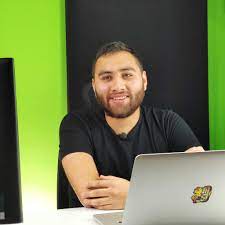
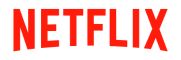