Concurrency Patterns in Go: Unlocking the Power of Parallelism
In today’s era of multi-core processors and distributed computing, harnessing the power of parallelism has become crucial for building high-performance applications. Go, with its built-in support for concurrency, provides developers with a powerful set of tools to achieve parallelism effectively. In this blog, we will explore various concurrency patterns in Go and learn how to unlock the true potential of parallelism.
1. Introduction to Concurrency in Go:
Concurrency in Go is achieved through goroutines and channels. Goroutines are lightweight threads of execution that allow functions to run concurrently. Channels, on the other hand, provide a means of communication and synchronization between goroutines. By combining goroutines and channels, developers can build elegant and efficient concurrent programs.
2. Goroutines: Lightweight Concurrency:
2.1. Creating Goroutines:
In Go, creating a goroutine is as simple as prefixing a function call with the keyword “go.” For example:
go func main() { go process() // Other code } func process() { // Do some work }
2.2. Synchronization with WaitGroups:
To ensure that the main goroutine waits for all other goroutines to finish their execution, we can use the sync.WaitGroup type. This allows us to synchronize goroutines by incrementing and decrementing a counter. Here’s an example:
go func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() // Goroutine 1 work }() go func() { defer wg.Done() // Goroutine 2 work }() wg.Wait() // Other code }
2.3. Using the sync package for Mutual Exclusion:
The sync package in Go provides synchronization primitives like Mutex and RWMutex for achieving mutual exclusion. These primitives ensure that only one goroutine can access a shared resource at a time. Here’s an example using Mutex:
go var counter int var mu sync.Mutex func increment() { mu.Lock() defer mu.Unlock() counter++ }
3. Channels: Communicating Sequential Processes:
Channels enable communication and synchronization between goroutines by providing a way to send and receive values. They follow the principle of “Do not communicate by sharing memory; instead, share memory by communicating.”
3.1. Creating Channels:
Channels can be created using the make function and specifying the type of values that will be passed through the channel. Here’s an example:
go ch := make(chan int) // Unbuffered channel
3.2. Channel Operations:
Channels support two main operations: sending and receiving values. Sending a value into a channel is achieved using the <- operator, and receiving a value is done by assigning the received value to a variable. Here’s an example:
go ch := make(chan int) go func() { ch <- 42 // Sending a value into the channel }() value := <-ch // Receiving a value from the channel
3.3. Buffered Channels:
In addition to unbuffered channels, Go also provides buffered channels. Buffered channels allow sending multiple values into the channel without the sender and receiver needing to be synchronized immediately. Here’s an example:
go ch := make(chan int, 3) // Buffered channel with a capacity of 3
3.4. Select Statement for Non-Blocking Communication:
The select statement in Go allows us to choose between multiple channel operations simultaneously. It helps prevent blocking and allows us to handle multiple channels efficiently. Here’s an example:
go select { case <-ch1: // Handle value received from ch1 case <-ch2: // Handle value received from ch2 default: // Default case if no value is ready }
4. Concurrency Patterns:
4.1. Fan-out/Fan-in:
The fan-out/fan-in pattern involves distributing work across multiple goroutines (fan-out) and collecting the results (fan-in). This pattern is useful when you have a large amount of work to be done concurrently and need to aggregate the results efficiently. Here’s an example:
go func worker(id int, jobs <-chan int, results chan<- int) { for j := range jobs { // Perform work results <- result } } func main() { jobs := make(chan int, 10) results := make(chan int, 10) // Start multiple workers for i := 0; i < numWorkers; i++ { go worker(i, jobs, results) } // Send jobs to be processed for j := 0; j < numJobs; j++ { jobs <- j } close(jobs) // Collect results for r := range results { // Process result } }
4.2. Worker Pools:
Worker pools involve creating a fixed number of goroutines that continuously listen to a job queue and perform the assigned work. This pattern is useful when you want to limit the number of concurrent goroutines and manage the work efficiently. Here’s an example:
go func worker(id int, jobs <-chan int, results chan<- int) { for j := range jobs { // Perform work results <- result } } func main() { jobs := make(chan int, 10) results := make(chan int, 10) // Start multiple workers for i := 0; i < numWorkers; i++ { go worker(i, jobs, results) } // Send jobs to be processed for j := 0; j < numJobs; j++ { jobs <- j } close(jobs) // Collect results for r := range results { // Process result } }
4.3. Pipeline Pattern:
The pipeline pattern involves connecting multiple stages together using channels, where each stage performs a specific operation on the input and passes the output to the next stage. This pattern is useful when you have a sequential data processing pipeline that can be parallelized. Here’s an example:
go func stage1(input <-chan int, output chan<- int) { for i := range input { // Process stage 1 output <- result } } func stage2(input <-chan int, output chan<- int) { for i := range input { // Process stage 2 output <- result } } func main() { input := make(chan int, 10) output := make(chan int, 10) // Start stage 1 go stage1(input, output) // Start stage 2 go stage2(output, input) // Send input to the pipeline for i := 0; i < numItems; i++ { input <- i } close(input) // Collect output from the pipeline for o := range output { // Process output } }
5. Error Handling in Concurrent Go Programs:
Proper error handling in concurrent Go programs is essential to ensure correctness and reliability. Techniques such as error propagation, error channels, and select statement with error handling can be used to handle errors effectively in concurrent scenarios.
Conclusion:
Concurrency patterns in Go, such as goroutines and channels, provide developers with a powerful toolbox for unlocking the power of parallelism. By understanding and applying these patterns, you can build high-performance concurrent applications that take full advantage of modern computing environments. Experiment with these patterns, explore the Go standard library, and embrace the power of concurrency in your projects. Happy coding!
Table of Contents
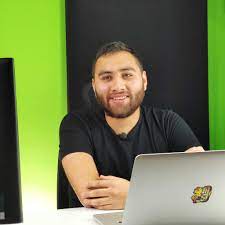
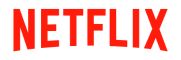