Introduction to Go’s Context Package: Managing Requests and Timeouts
In the world of concurrent and networked programming, managing resources efficiently is paramount. One of the challenges developers face is gracefully handling request cancellations, timeouts, and sharing data among goroutines. This is where Go’s Context package comes to the rescue. In this blog post, we’ll delve into the basics of the Context package, understand its significance, and explore how it simplifies the management of requests and timeouts in Go applications.
1. Understanding the Need for Context
In a Go application, where multiple goroutines can run concurrently, it’s crucial to manage the lifecycle of these goroutines effectively. This includes scenarios like handling user requests, managing database connections, and even canceling operations if a user cancels their request or a timeout occurs.
Without a structured way to manage these situations, code can quickly become complex, error-prone, and challenging to maintain. The Context package was introduced to address these challenges and provide a clean and standardized way to handle request-scoped values, deadlines, and cancellations.
2. Anatomy of a Context
At its core, a context.Context is a data structure that carries deadlines, cancellations, and other values across API boundaries and between processes. It acts as a conduit to pass information down the call chain to goroutines spawned to handle incoming requests. Let’s break down the components of a context:
2.1. Context Creation
A context can be created using the context.Background() function, which returns an empty context. This empty context serves as the root of all contexts in a program. Alternatively, you can create a context with a parent context using context.WithContext(parent). This enables the propagation of values and cancellations from the parent context to child contexts.
go package main import ( "context" "fmt" ) func main() { parentContext := context.Background() childContext := context.WithValue(parentContext, "key", "value") value := childContext.Value("key").(string) fmt.Println(value) // Output: value }
2.2. Adding Values to Context
The context.WithValue(parent, key, value) function allows you to add key-value pairs to a context. While this can be useful for passing data down the call chain, it’s important to note that it should not be used for sensitive or large data, as contexts are meant for short-lived values.
2.3. Setting Deadlines
Contexts can also be associated with deadlines. This is extremely useful when you want to limit the duration of an operation. The context.WithDeadline(parent, deadline) function returns a new context with a deadline. Once the deadline is reached, the context becomes canceled, and all goroutines derived from it are signaled to clean up.
go package main import ( "context" "fmt" "time" ) func main() { parentContext := context.Background() deadline := time.Now().Add(5 * time.Second) childContext, cancel := context.WithDeadline(parentContext, deadline) defer cancel() select { case <-childContext.Done(): fmt.Println("Operation timed out") case <-time.After(10 * time.Second): fmt.Println("Operation completed successfully") } }
2.4. Handling Cancellations
Cancellations are a fundamental aspect of contexts. When a context is canceled, all contexts derived from it are also canceled. The context.WithCancel(parent) function returns a child context and a cancellation function. Calling this function signals that the work associated with the context should be canceled.
go package main import ( "context" "fmt" "time" ) func main() { parentContext := context.Background() childContext, cancel := context.WithCancel(parentContext) go func() { // Simulate some work time.Sleep(2 * time.Second) cancel() // Cancel the context after the work is done }() select { case <-childContext.Done(): fmt.Println("Operation canceled") case <-time.After(5 * time.Second): fmt.Println("Operation completed successfully") } }
3. Use Cases for Contexts
The Context package finds its applications in various scenarios where managing requests, deadlines, and cancellations is essential. Some of these use cases include:
3.1. HTTP Servers
In an HTTP server, each incoming request should ideally have its own context. This ensures that the request’s scope is appropriately managed, and any resources associated with it are cleaned up if the request is canceled or times out.
go package main import ( "context" "fmt" "net/http" "time" ) func handler(w http.ResponseWriter, r *http.Request) { requestContext, cancel := context.WithTimeout(context.Background(), 3*time.Second) defer cancel() select { case <-requestContext.Done(): fmt.Fprintln(w, "Request timed out") case <-time.After(2 * time.Second): fmt.Fprintln(w, "Request completed successfully") } } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
3.2. Database Operations
When dealing with database connections or queries, a context can be used to manage the duration of these operations. If an operation takes longer than expected, it can be canceled, preventing resource leaks.
go package main import ( "context" "database/sql" "fmt" "time" _ "github.com/mattn/go-sqlite3" ) func main() { db, err := sql.Open("sqlite3", "test.db") if err != nil { panic(err) } defer db.Close() ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() _, err = db.ExecContext(ctx, "INSERT INTO users (name) VALUES (?)", "Alice") if err != nil { fmt.Println("Error:", err) } else { fmt.Println("User inserted successfully") } }
Conclusion
The Context package in Go provides a robust and standardized way to manage requests, deadlines, and cancellations in concurrent applications. By utilizing contexts, developers can simplify code, improve resource management, and enhance the overall reliability of their applications. Whether you’re building an HTTP server, interacting with databases, or handling any other scenario involving concurrent tasks, understanding and effectively using the Context package is an essential skill for any Go developer.
Table of Contents
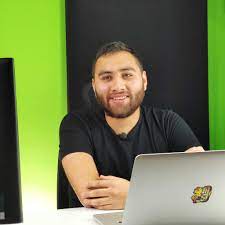
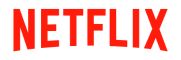