How do you handle CORS in Go?
CORS (Cross-Origin Resource Sharing) is a security feature implemented by web browsers to prevent unauthorized cross-origin requests from accessing resources on a server. In Go, handling CORS involves configuring the HTTP headers sent by the server to allow or deny cross-origin requests from client-side JavaScript applications.
To handle CORS in Go, you can use middleware provided by the github.com/rs/cors package, which simplifies the process of configuring CORS headers in Go web applications.
Here’s how you can handle CORS in Go using the github.com/rs/cors package:
- Install the CORS middleware: First, you need to install the github.com/rs/cors package using the go get command:
bash go get github.com/rs/cors
- Import the CORS package: Next, import the github.com/rs/cors package in your Go application:
go import "github.com/rs/cors"
- Create a CORS middleware instance: Create a new CORS middleware instance with the desired CORS options, such as allowed origins, methods, headers, and credentials:
go c := cors.New(cors.Options{ AllowedOrigins: []string{"http://example.com", "https://example.com"}, AllowedMethods: []string{"GET", "POST", "PUT", "DELETE"}, AllowedHeaders: []string{"Authorization", "Content-Type"}, AllowCredentials: true, })
- Wrap your HTTP handler with CORS middleware: Wrap your existing HTTP handler or router with the CORS middleware using the Handler method:
go handler := http.HandlerFunc(yourHandlerFunction) http.ListenAndServe(":8080", c.Handler(handler))
By wrapping your HTTP handler with the CORS middleware, you ensure that the appropriate CORS headers are added to HTTP responses, allowing or denying cross-origin requests based on the specified configuration.
Handling CORS in Go ensures that your web server complies with browser security policies and allows client-side JavaScript applications to make cross-origin requests safely and securely.
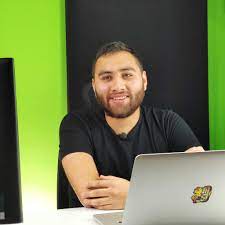
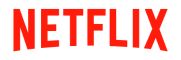