How do you create and use channels in Go?
In Go, channels are created using the make function with the chan keyword followed by the specified element type. Channels facilitate communication and synchronization between goroutines—the lightweight threads of execution in Go.
Here’s how you can create a channel of integers in Go:
go ch := make(chan int)
Channels support two primary operations: sending and receiving values using the <- operator. To send a value into a channel, you use the send operation <- followed by the channel variable and the value to be sent. To receive a value from a channel, you use the receive operation <- followed by the channel variable.
Here’s how you can send and receive values using a channel in Go:
go // Sending a value into the channel ch <- 42 // Receiving a value from the channel value := <-ch
By default, both send and receive operations on a channel block until the other side is ready. This synchronization mechanism enables goroutines to communicate effectively and avoid race conditions.
Channels can also be buffered, meaning they have a limited capacity to hold values. Buffered channels allow goroutines to send and receive values asynchronously up to a certain limit, after which further send operations will block until space becomes available in the buffer.
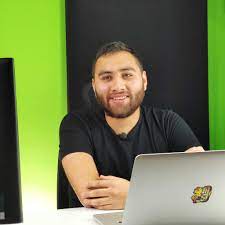
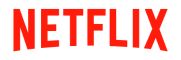