Creating GraphQL APIs with Go: Building Flexible and Efficient APIs
In the realm of modern web development, building APIs is a foundational aspect of creating dynamic and interactive applications. Traditional REST APIs have been widely used, but a newer approach known as GraphQL has gained popularity due to its flexibility and efficiency. In this tutorial, we will delve into the world of GraphQL APIs using the Go programming language. By the end of this guide, you’ll have a solid understanding of how to create powerful APIs that cater to your application’s unique data needs.
1. Understanding GraphQL
1.1. What is GraphQL?
GraphQL is a query language for APIs that was developed by Facebook in 2012 and released to the public in 2015. Unlike traditional REST APIs, where clients have limited control over the data they receive, GraphQL empowers clients to request exactly the data they need, and nothing more. This overcomes the problem of over-fetching or under-fetching data that can occur with REST APIs.
1.2. Key Advantages of GraphQL
- Flexible Queries: Clients can specify the structure of the response, eliminating over-fetching and under-fetching of data.
- Efficiency: GraphQL minimizes the number of requests by allowing multiple queries to be combined into a single request.
- Strongly Typed Schema: The schema defines the types of data that can be queried and the relationships between them.
- Real-time Data: GraphQL supports real-time data updates using subscriptions.
- Introspection: Clients can introspect the schema to discover the available types and queries.
2. Getting Started with Go
2.1. Setting Up Your Development Environment
Before diving into GraphQL with Go, you’ll need to set up your development environment. Ensure you have Go installed and a code editor of your choice ready.
2.3. Installing Go Libraries
To start building GraphQL APIs with Go, you’ll need to install the required libraries. Some popular libraries for working with GraphQL in Go include:
- github.com/graphql-go/graphql: This is the core library that allows you to define your schema, types, and resolvers.
- github.com/graphql-go/handler: This library helps you create a GraphQL HTTP handler for your server.
You can install these libraries using the following commands:
bash go get github.com/graphql-go/graphql go get github.com/graphql-go/handler
3. Building Your First GraphQL Schema
At the heart of any GraphQL API is the schema. The schema defines the types of data that can be queried and the relationships between them.
3.1. Defining Types and Fields
In Go, you can define your types using the graphql.NewObject function. Let’s say you’re building a simple blog application. You might have types for User, Post, and Comment. Here’s an example of how you might define the User type:
go import ( "github.com/graphql-go/graphql" ) var userType = graphql.NewObject( graphql.ObjectConfig{ Name: "User", Fields: graphql.Fields{ "id": &graphql.Field{ Type: graphql.Int, }, "username": &graphql.Field{ Type: graphql.String, }, // Other fields... }, }, )
3.2. Resolvers: Connecting Data and Types
Resolvers are functions that define how to fetch the data for a specific field. You’ll need to create resolvers for each field in your schema. Continuing with the User example, here’s how you might define a resolver for the username field:
go var rootQuery = graphql.NewObject( graphql.ObjectConfig{ Name: "Query", Fields: graphql.Fields{ "user": &graphql.Field{ Type: userType, Args: graphql.FieldConfigArgument{ "id": &graphql.ArgumentConfig{ Type: graphql.Int, }, }, Resolve: func(params graphql.ResolveParams) (interface{}, error) { // Fetch user data based on the provided ID id, _ := params.Args["id"].(int) // Fetch user data from your data source user := getUserByID(id) return user, nil }, }, // Other fields... }, }, )
4. Querying Data with GraphQL
With your schema and resolvers in place, you can start querying data using GraphQL.
4.1. Writing and Executing Queries
Queries in GraphQL are written using a syntax that resembles the shape of the desired response. Here’s an example query that fetches a user’s ID and username:
graphql { user(id: 1) { id username } }
4.2. Retrieving Specific Data
One of the strengths of GraphQL is the ability to retrieve specific data without over-fetching. Clients can request only the fields they need. For instance, if you only need the user’s username, you can modify the query accordingly:
graphql { user(id: 1) { username } }
5. Mutating Data with GraphQL
GraphQL doesn’t just handle data retrieval; it also allows you to modify data on the server using mutations.
5.1. Altering Data on the Server
Mutations are similar to queries but are used for making changes to data. Let’s say you want to implement a mutation for creating a new post:
go var rootMutation = graphql.NewObject( graphql.ObjectConfig{ Name: "Mutation", Fields: graphql.Fields{ "createPost": &graphql.Field{ Type: postType, Args: graphql.FieldConfigArgument{ "title": &graphql.ArgumentConfig{ Type: graphql.NewNonNull(graphql.String), }, "content": &graphql.ArgumentConfig{ Type: graphql.NewNonNull(graphql.String), }, }, Resolve: func(params graphql.ResolveParams) (interface{}, error) { // Extract and validate arguments title := params.Args["title"].(string) content := params.Args["content"].(string) // Create a new post and return it post := createNewPost(title, content) return post, nil }, }, // Other mutation fields... }, }, )
5.2. Input Types and Mutations
In the example above, the mutation takes arguments for the new post’s title and content. You can enhance this by using input types:
go var postInputType = graphql.NewInputObject( graphql.InputObjectConfig{ Name: "PostInput", Fields: graphql.InputObjectConfigFieldMap{ "title": &graphql.InputObjectFieldConfig{ Type: graphql.NewNonNull(graphql.String), }, "content": &graphql.InputObjectFieldConfig{ Type: graphql.NewNonNull(graphql.String), }, }, }, ) // ... "createPost": &graphql.Field{ Type: postType, Args: graphql.FieldConfigArgument{ "post": &graphql.ArgumentConfig{ Type: graphql.NewNonNull(postInputType), }, }, Resolve: func(params graphql.ResolveParams) (interface{}, error) { // Extract and validate the post input postInput := params.Args["post"].(map[string]interface{}) title := postInput["title"].(string) content := postInput["content"].(string) // Create a new post and return it post := createNewPost(title, content) return post, nil }, },
6. Best Practices for Efficient APIs
Efficiency is crucial in any API design. Here are some best practices to consider when building your GraphQL API with Go:
6.1. Data Fetching Techniques
- Batching and Caching: Reduce the number of database queries by batching and caching requests. Tools like DataLoader can help with batching.
- Lazy Loading: Only fetch data that’s requested. For example, if your schema has nested fields, fetch the related data only when those fields are queried.
- N+1 Query Problem: Be aware of the N+1 query problem, where a single query triggers N additional queries for related data. Use techniques like “field merging” or “batched resolvers” to address this issue.
6.2. Caching and Batching
- Caching: Implement caching mechanisms to store frequently accessed data in memory or an external cache. This reduces the load on your database.
- Batching: Group multiple similar queries together and process them in a single request to minimize overhead.
7. Securing Your GraphQL API
While building powerful APIs is essential, security is equally important. Here’s how you can secure your GraphQL API:
7.1. Authentication and Authorization
- Authentication: Implement user authentication mechanisms, such as JWT (JSON Web Tokens), OAuth, or API keys.
- Authorization: Control access to different parts of your API based on user roles and permissions. Use middleware to handle authorization logic.
7.2. Rate Limiting and Security Measures
- Rate Limiting: Prevent abuse and DDoS attacks by implementing rate limiting on your API endpoints.
- Input Validation: Sanitize and validate input data to prevent SQL injection, XSS attacks, and other security vulnerabilities.
8. Beyond the Basics
As you become more comfortable with GraphQL and Go, you can explore advanced topics to enhance your API:
8.1. Pagination and Filtering
Implement pagination for queries that return lists of data. Use arguments like first, last, before, and after to paginate through results.
Provide filtering options to allow clients to narrow down their queries based on specific criteria.
8.2. Handling Errors and Validation
GraphQL provides a structured way to handle errors. Return errors in a consistent format, including error codes and messages.
Implement input validation to ensure that data sent by clients adheres to your API’s rules and constraints.
9. Testing Your GraphQL API
Testing is crucial to ensure the reliability and correctness of your API. Here’s how you can test your GraphQL API:
9.1. Unit and Integration Testing
Write unit tests for individual resolvers and mutations to ensure they behave as expected.
Perform integration tests that cover entire query and mutation workflows.
9.2. Mocking Data for Testing
Use mocking libraries to simulate database responses and external services. This allows you to test without relying on real data sources.
10. Deploying Your GraphQL API
Once your GraphQL API is ready, it’s time to deploy it for the world to access. Consider the following deployment strategies:
10.1. Choosing Hosting Options
Deploy your API on cloud platforms like AWS, Google Cloud, or Azure.
Utilize serverless options for cost-effective scaling.
10.2. Containerization and Deployment Strategies
Package your API as a container using Docker for consistent deployment across different environments.
Use continuous integration and continuous deployment (CI/CD) pipelines for automated deployments.
Conclusion
In conclusion, building flexible and efficient GraphQL APIs with Go empowers you to create dynamic applications that cater to specific data needs. By understanding the core concepts of GraphQL, setting up your development environment, and following best practices, you can craft APIs that deliver data efficiently while maintaining security and scalability. As you gain expertise, you can explore advanced features, refine your testing strategies, and deploy your APIs with confidence. The combination of GraphQL and Go provides a powerful toolset for modern API development, enabling you to unlock the full potential of your applications.
Table of Contents
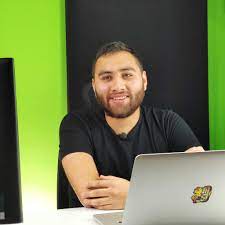
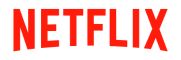