Creating RESTful APIs with Go and the Echo Framework
In the world of web development, building robust and efficient RESTful APIs is a common requirement. These APIs serve as the backbone for countless web and mobile applications, enabling them to interact with server-side data and services seamlessly. When it comes to developing RESTful APIs with Go, the Echo Framework stands out as a popular and highly performant choice.
In this comprehensive guide, we’ll dive into the world of RESTful API development with Go and Echo. Whether you’re a seasoned Go developer or just getting started with the language, you’ll find valuable insights, step-by-step tutorials, and code samples to help you create RESTful APIs that are both powerful and maintainable.
1. Why Choose Go and Echo for RESTful APIs?
Before we get into the nitty-gritty of creating RESTful APIs with Go and Echo, let’s understand why this combination is an excellent choice for your next API project.
1.1. The Power of Go
- Performance: Go is known for its remarkable performance. It compiles to native machine code, resulting in highly efficient programs. This makes Go an ideal choice for building APIs that need to handle a large number of concurrent requests.
- Concurrency: Go’s built-in concurrency features, such as goroutines and channels, make it easy to write concurrent and parallel code. This is crucial for developing APIs that can scale and handle multiple requests simultaneously.
- Statically Typed: Go is statically typed, which means you catch many errors at compile time, rather than at runtime. This helps ensure the reliability and stability of your API.
- Rich Standard Library: Go comes with a rich standard library that includes packages for handling HTTP requests, JSON encoding/decoding, and more. This makes building APIs in Go both efficient and convenient.
1.2. The Echo Framework
Echo is a high-performance, minimalist Go web framework specifically designed for building web APIs. Here’s why Echo is a fantastic choice:
- Lightweight: Echo is lightweight and does not impose unnecessary abstractions or overhead. This allows you to have fine-grained control over your API.
- Performance: Echo is designed for high performance and low latency. It’s one of the fastest Go web frameworks available.
- Robust Routing: Echo provides a robust and flexible routing system, making it easy to define routes, parameters, and middleware.
- Middleware Support: Echo has excellent middleware support, enabling you to add features like authentication, logging, and request/response modification easily.
Now that we’ve established why Go and Echo make an excellent combination for building RESTful APIs, let’s get started with the practical aspects of creating APIs.
2. Setting Up Your Development Environment
Before we start coding, you’ll need to set up your development environment. Make sure you have Go installed on your system. You can download it from the official Go website.
Once Go is installed, create a new directory for your project and set up a Go module:
shell mkdir my-api cd my-api go mod init my-api
Now, you’re ready to install Echo and start building your RESTful API.
3. Creating Your First API Endpoint
Let’s begin by creating a simple API endpoint that responds with a “Hello, World!” message. We’ll gradually build upon this example to demonstrate more complex features of Echo.
Create a file named main.go in your project directory and add the following code:
go package main import ( "net/http" "github.com/labstack/echo/v4" ) func main() { e := echo.New() // Define a route e.GET("/", func(c echo.Context) error { return c.String(http.StatusOK, "Hello, World!") }) // Start the server e.Start(":8080") }
In this code:
- We import the necessary packages, including Echo, which we installed earlier.
- We create an instance of the Echo framework.
- We define a simple route using the GET method. When a request is made to the root URL (“/”), it responds with a “Hello, World!” message.
- Finally, we start the Echo server on port 8080.
To run your API, execute the following command in your project directory:
shell go run main.go
You should see a message indicating that the server is running. Open your web browser and navigate to http://localhost:8080, and you should see the “Hello, World!” message displayed.
Congratulations! You’ve just created your first API endpoint with Go and Echo. Now, let’s explore some essential concepts and features of building RESTful APIs.
4. Handling Route Parameters
In many real-world scenarios, APIs need to accept parameters from the URL to perform specific actions or retrieve specific data. Echo makes it easy to handle route parameters.
4.1. Path Parameters
Let’s create an API endpoint that accepts a username as a path parameter and responds with a personalized message.
go e.GET("/hello/:username", func(c echo.Context) error { username := c.Param("username") return c.String(http.StatusOK, "Hello, "+username+"!") })
In this code, we define a route /hello/:username, where :username is a placeholder for the actual username provided in the URL. We extract this parameter using c.Param(“username”) and include it in the response message.
To test this endpoint, visit http://localhost:8080/hello/John, and you should see a personalized greeting.
4.2. Query Parameters
In addition to path parameters, APIs often accept query parameters in the URL. Query parameters are typically used for filtering, sorting, or providing additional information to an API endpoint.
Let’s create an API endpoint that accepts a name query parameter and responds with a personalized message.
go e.GET("/greet", func(c echo.Context) error { name := c.QueryParam("name") if name == "" { name = "Guest" } return c.String(http.StatusOK, "Hello, "+name+"!") })
In this code, we use c.QueryParam(“name”) to extract the name query parameter from the URL. If the parameter is not provided, we default to “Guest.”
To test this endpoint, visit http://localhost:8080/greet?name=Alice, and you should see a personalized greeting. If you visit http://localhost:8080/greet without the name parameter, it will default to “Guest.”
5. Handling Request Data
So far, we’ve seen how to create API endpoints that respond to GET requests with static data. However, most APIs need to handle POST, PUT, or DELETE requests that involve sending data to the server. Let’s explore how to handle request data in Echo.
5.1. Handling POST Requests
To handle POST requests, you’ll often need to parse the incoming JSON or form data. Echo provides convenient methods to do this.
Let’s create an API endpoint that accepts a JSON object with a message field and echoes it back.
go type Message struct { Message string `json:"message"` } e.POST("/echo", func(c echo.Context) error { msg := new(Message) if err := c.Bind(msg); err != nil { return err } return c.JSON(http.StatusOK, msg) })
In this code, we define a Message struct to represent the JSON data we expect to receive. We then use c.Bind(msg) to parse the JSON data from the request body into the msg variable. Finally, we respond with the received message in JSON format.
To test this endpoint, you can use a tool like curl or Postman to send a POST request with JSON data:
shell curl -X POST -H "Content-Type: application/json" -d '{"message":"Hello, Echo!"}' http://localhost:8080/echo
You should receive a JSON response echoing the message you sent.
5.2. Handling Form Data
Handling form data is just as straightforward. Let’s create an API endpoint that accepts form data with a name field and responds with a greeting.
go e.POST("/greet-form", func(c echo.Context) error { name := c.FormValue("name") if name == "" { name = "Guest" } return c.String(http.StatusOK, "Hello, "+name+"!") })
In this code, we use c.FormValue(“name”) to retrieve the name field from the form data. If the field is not provided, we default to “Guest.”
To test this endpoint, you can create an HTML form or use a tool like curl:
shell curl -X POST -d "name=Alice" http://localhost:8080/greet-form
You should receive a text response with a personalized greeting.
6. Middleware for Authentication and Logging
Middleware functions in Echo provide a way to intercept and process requests and responses. This allows you to add various functionalities like authentication, logging, and request/response modification to your API.
6.1. Logging Middleware
Logging is a crucial aspect of API development. It helps in debugging, monitoring, and analyzing the behavior of your API. Echo makes it easy to set up logging middleware.
go // Logger middleware e.Use(middleware.Logger())
By simply adding middleware.Logger(), you enable request and response logging for your API. The logs will include details like the HTTP method, URL, status code, and response time. This is incredibly useful for diagnosing issues and tracking the usage of your API.
6.2. Authentication Middleware
Implementing authentication in an API is often a complex task. Echo simplifies this by allowing you to define custom authentication middleware.
Let’s create a basic authentication middleware that checks for a custom Authorization header with a predefined token.
go // Authentication middleware func authMiddleware(next echo.HandlerFunc) echo.HandlerFunc { return func(c echo.Context) error { token := c.Request().Header.Get("Authorization") if token != "my-secret-token" { return echo.ErrUnauthorized } return next(c) } } // Protected route e.GET("/protected", func(c echo.Context) error { return c.String(http.StatusOK, "This is a protected resource.") }, authMiddleware)
In this code, we define a custom authMiddleware function that checks for the presence of the Authorization header with a specific token. If the token is valid, the middleware calls the next handler; otherwise, it returns an unauthorized error.
The authMiddleware is applied to the /protected route by passing it as the second argument to e.GET.
Now, when you make a request to http://localhost:8080/protected without the correct Authorization header, you will receive a 401 Unauthorized response.
7. Error Handling
Robust error handling is crucial for any API. Echo provides mechanisms for handling errors gracefully and returning meaningful responses to clients.
7.1. Custom Error Handling
You can create custom error handling middleware to centralize error processing in your API. This middleware can capture errors and format responses consistently.
go // Custom error handler middleware func errorHandler(next echo.HandlerFunc) echo.HandlerFunc { return func(c echo.Context) error { err := next(c) if err != nil { // Log the error fmt.Println("Error:", err) // Send an error response return c.JSON(http.StatusInternalServerError, map[string]interface{}{ "error": err.Error(), }) } return nil } } // Simulate an error in a route e.GET("/error", func(c echo.Context) error { return errors.New("Something went wrong") }, errorHandler)
In this code, we define an errorHandler middleware that captures errors returned by the next handler, logs them, and sends a JSON response with an error message and a 500 Internal Server Error status code.
The errorHandler middleware is applied to the /error route, which simulates an error by returning an error object.
Now, when you visit http://localhost:8080/error, you will receive a JSON response with the error message.
7.3. HTTP Errors
Echo provides a set of predefined HTTP error types that you can return from your handlers. These error types come with built-in error messages and HTTP status codes.
go // Return a 404 Not Found error return echo.NewHTTPError(http.StatusNotFound, "Resource not found")
You can use echo.NewHTTPError() to create custom error responses with specific status codes and messages.
8. Testing Your API
Testing is a critical part of API development. Echo provides a testing package that allows you to write unit tests for your API handlers.
Let’s create a simple test for our /hello/:username endpoint:
go func TestHelloEndpoint(t *testing.T) { // Create a new Echo instance e := echo.New() // Define the request req := httptest.NewRequest(http.MethodGet, "/hello/Alice", nil) rec := httptest.NewRecorder() c := e.NewContext(req, rec) // Call the handler function if assert.NoError(t, helloEndpoint(c)) { assert.Equal(t, http.StatusOK, rec.Code) assert.Equal(t, "Hello, Alice!", rec.Body.String()) } }
In this test:
- We create a new Echo instance.
- We define a request using httptest.NewRequest() with the desired URL and method.
- We create a recorder to capture the response.
- We create a context using e.NewContext() with the request and response.
- We call the helloEndpoint handler function and use assertions to check the response status code and body.
You can write similar tests for all your API endpoints to ensure they behave as expected.
Conclusion
In this guide, we’ve explored the fundamentals of creating RESTful APIs with Go and the Echo Framework. We covered essential topics such as routing, request handling, middleware, error handling, and testing.
Go’s performance and concurrency features, combined with Echo’s simplicity and performance, make them an excellent choice for building powerful and efficient APIs. Whether you’re building a simple JSON API or a complex microservice, Go and Echo have you covered.
Remember that building RESTful APIs is just the beginning. As your API evolves, you can explore additional features like database integration, authentication with OAuth or JWT, and more. The Echo Framework provides the flexibility and extensibility to adapt to your evolving needs.
Now that you have a solid foundation in Go and Echo for API development, you’re ready to embark on your journey to create scalable and reliable RESTful APIs. Happy coding!
Table of Contents
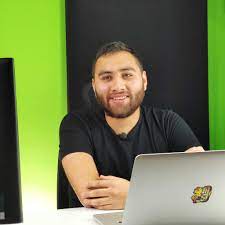
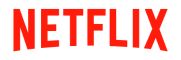