Creating Serverless Functions with Go and AWS Lambda
In today’s rapidly evolving technology landscape, serverless computing has emerged as a game-changer, enabling developers to build and deploy applications without the hassle of managing server infrastructure. AWS Lambda, a serverless compute service provided by Amazon Web Services, is at the forefront of this revolution. In this blog, we’ll explore how to create serverless functions using the Go programming language and AWS Lambda.
1. Why Serverless with AWS Lambda?
Before diving into the nitty-gritty of serverless functions with Go and AWS Lambda, let’s briefly discuss why this combination is worth exploring:
1.1. Scalability
One of the most significant advantages of using AWS Lambda is its ability to automatically scale your functions in response to incoming traffic. Whether you have one user or a million, AWS Lambda can handle the load without any manual intervention. This makes it an ideal choice for applications with unpredictable workloads.
1.2. Cost-effectiveness
With AWS Lambda, you only pay for the compute time your functions consume. There are no upfront costs or idle server expenses, making it a cost-effective solution for startups and enterprises alike. It’s an excellent way to optimize your infrastructure expenses.
1.3. Quick Deployment
AWS Lambda allows you to focus on code development without worrying about server provisioning, maintenance, or scaling. This means faster development cycles and quicker time-to-market for your applications.
Now that we understand the benefits let’s get started on creating serverless functions with Go and AWS Lambda.
2. Prerequisites
Before we begin, make sure you have the following prerequisites in place:
2.1. AWS Account
You’ll need an AWS account to use AWS Lambda. If you don’t have one, you can sign up here.
2.2. AWS Command Line Interface (CLI)
Install the AWS CLI by following the instructions provided here.
2.3. Go Programming Language
You should have Go installed on your local machine. You can download it from the official website here.
3. Setting up Your Development Environment
Now that you have the prerequisites in place, let’s set up your development environment. We’ll start by configuring the AWS CLI.
3.1. Configuring AWS CLI
Open your terminal and run the following command to configure the AWS CLI with your AWS credentials:
bash aws configure
You’ll be prompted to enter your AWS Access Key ID, Secret Access Key, default region, and output format. Make sure you provide the correct values.
3.2. Installing the AWS Lambda Go SDK
To interact with AWS Lambda from your Go code, you’ll need to install the AWS Lambda Go SDK. Run the following command to install it:
bash go get github.com/aws/aws-lambda-go/lambda
With your environment set up, let’s move on to creating your first serverless function.
4. Creating Your First Serverless Function
In this section, we’ll create a simple AWS Lambda function using Go. Our function will take an input event, process it, and return a response.
Step 1: Create a Go File
Create a new directory for your project and inside it, create a Go file, e.g., main.go. This file will contain your AWS Lambda function code.
go // main.go package main import ( "context" "fmt" "github.com/aws/aws-lambda-go/lambda" ) // Handler is our lambda handler invoked by the `lambda.Start` function call func Handler(ctx context.Context) (string, error) { return "Hello, Serverless World!", nil } func main() { lambda.Start(Handler) }
In this example, we’ve created a simple Lambda handler function that returns the string “Hello, Serverless World!”.
Step 2: Build the Go Binary
Before deploying your code to AWS Lambda, you need to build a Go binary that can run on the Lambda execution environment. Run the following command to build the binary:
bash GOOS=linux GOARCH=amd64 go build main.go
This command compiles your Go code for the Linux environment, which is the execution environment used by AWS Lambda.
Step 3: Deploy to AWS Lambda
To deploy your function to AWS Lambda, you’ll use the AWS CLI. Run the following command to create a new Lambda function:
bash aws lambda create-function \ --function-name MyGoLambdaFunction \ --runtime go1.x \ --handler main \ --zip-file fileb://main
This command does the following:
- –function-name: Specifies the name of your Lambda function.
- –runtime: Specifies the runtime environment, which is Go 1.x in our case.
- –handler: Specifies the name of the handler function in your Go code.
- –zip-file: Specifies the path to the Go binary we built earlier.
Step 4: Invoke Your Lambda Function
Once your function is deployed, you can invoke it using the AWS CLI:
bash aws lambda invoke \ --function-name MyGoLambdaFunction \ --payload '{}' \ output.txt
This command invokes your Lambda function with an empty JSON payload and writes the response to output.txt.
Congratulations! You’ve created and deployed your first serverless function with Go and AWS Lambda. But this is just the beginning.
5. Handling Events
So far, our Lambda function has been very basic. In practice, you’ll often want your function to respond to specific events, such as HTTP requests or changes in an S3 bucket. Let’s explore how to do this.
5.1. HTTP Events
To create a Lambda function that responds to HTTP events, you can use Amazon API Gateway in conjunction with Lambda. Here’s a high-level overview of the process:
- Create an API Gateway.
- Create a resource and method (e.g., POST).
- Connect the method to your Lambda function.
- Deploy your API.
Let’s walk through these steps:
Step 1: Create an API Gateway
In the AWS Management Console, navigate to API Gateway and click “Create API.” Choose “HTTP API” and click “Build.”
Step 2: Create a Resource and Method
In your API, create a resource (e.g., /myresource) and a method (e.g., POST) under that resource.
Step 3: Connect to Lambda
In the method configuration, choose “Lambda Function” as the integration type. Select your Lambda function from the list.
Step 4: Deploy Your API
Deploy your API to a stage (e.g., “prod”). You’ll be provided with an API URL that you can use to trigger your Lambda function via HTTP requests.
Now, when you send an HTTP request to the URL associated with your API, it will trigger your Lambda function.
S3 Events
AWS Lambda can also be triggered by events from various AWS services, including Amazon S3. For example, you can configure a Lambda function to run whenever a new file is uploaded to an S3 bucket. Here’s how to set it up:
5.2. Create an S3 bucket.
- Create a Lambda function.
- Configure the Lambda function to be triggered by S3 events.
Step 1: Create an S3 Bucket
In the AWS Management Console, navigate to S3 and click “Create Bucket.” Follow the prompts to create your bucket.
Step 2: Create a Lambda Function
Create a new Lambda function as we did earlier.
Step 3: Configure S3 Trigger
In your Lambda function configuration, add an S3 trigger and specify the bucket and event type (e.g., object creation).
Now, whenever a new file is uploaded to the specified S3 bucket, your Lambda function will automatically run, allowing you to process the file or perform any required actions.
6. Working with Dependencies
In real-world scenarios, your Lambda functions may have dependencies, such as external libraries or packages. You can include these dependencies by packaging them with your Lambda deployment package.
Here’s a step-by-step guide on how to work with dependencies in your Lambda function:
Step 1: Create a Deployment Package
Before you can package your dependencies, you need to create a deployment package that includes your Lambda function code and its dependencies. Here’s how you can do this:
bash # Create a directory for your deployment package mkdir deployment-package # Copy your Lambda function binary into the deployment package directory cp main deployment-package/ # Create a Go module to manage your dependencies cd deployment-package go mod init my-lambda-function
Step 2: Add Dependencies
Using the Go module created in the previous step, you can now add your project’s dependencies. For example, let’s say your Lambda function depends on the popular Go package “github.com/aws/aws-sdk-go/aws.” You can add it like this:
bash go get github.com/aws/aws-sdk-go/aws
This command will download the package and add it to your Go module.
Step 3: Build the Deployment Package
Now that you’ve added your dependencies, build the deployment package:
bash GOOS=linux GOARCH=amd64 go build main
This command compiles your Lambda function binary along with its dependencies.
Step 4: Deploy Your Function
Finally, deploy your Lambda function using the AWS CLI as we did earlier. Make sure to reference the binary inside your deployment package directory:
bash aws lambda create-function \ --function-name MyGoLambdaFunction \ --runtime go1.x \ --handler main \ --zip-file fileb://deployment-package/main
Your Lambda function is now configured to use the dependencies included in the deployment package.
7. Testing and Debugging
Testing and debugging serverless functions with Go and AWS Lambda is essential to ensure your code functions as expected. Here are some tips and techniques:
7.1. Local Testing
Before deploying your function to AWS Lambda, you can test it locally using the AWS SAM CLI. SAM (Serverless Application Model) provides a local environment that mimics the Lambda execution environment.
You can define a SAM template (usually named template.yaml) that describes your function and its event sources. Then, use SAM CLI to invoke your function locally, passing sample event data.
7.2. Logging and Monitoring
AWS Lambda provides built-in monitoring and logging through Amazon CloudWatch. You can instrument your code with logging statements using the log package in Go. These logs will be available in CloudWatch, allowing you to track the execution of your functions and diagnose issues.
7.3. Debugging
For debugging purposes, you can use traditional Go debugging tools, such as fmt.Println for simple debugging or a debugger like Delve for more complex scenarios. To debug Lambda functions locally with Delve, you can follow the official guide.
8. Best Practices
To make the most out of serverless functions with Go and AWS Lambda, consider the following best practices:
8.1. Keep Functions Small and Focused
Break down your application into small, focused functions. This allows for better code maintainability and easier testing.
8.2. Optimize Cold Starts
Cold starts can add latency to your function invocations. To mitigate this, use layers for common dependencies, and consider warming up your functions using scheduled CloudWatch Events.
8.3. Error Handling
Implement robust error handling in your functions to gracefully handle failures and provide meaningful error messages.
8.4. Security
Follow AWS security best practices, and consider using AWS Identity and Access Management (IAM) roles to restrict permissions.
8.5. Monitoring and Alerts
Set up monitoring and alerting in CloudWatch to proactively detect and respond to issues in your functions.
Conclusion
Creating serverless functions with Go and AWS Lambda can unlock the benefits of scalability, cost-effectiveness, and quick deployment for your applications. With the right setup and practices, you can build efficient and reliable serverless solutions that scale effortlessly to meet your application’s demands.
In this blog, we’ve covered the fundamentals of creating serverless functions with Go and AWS Lambda, from setting up your development environment to handling various types of events, managing dependencies, testing, debugging, and best practices. Armed with this knowledge, you’re ready to embark on your serverless journey and build powerful, serverless applications using Go and AWS Lambda.
Table of Contents
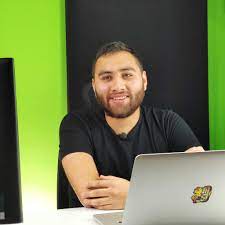
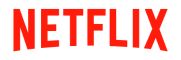