Go Q & A
How do you handle cross-origin requests in Go?
Cross-origin resource sharing (CORS) is a security feature implemented by web browsers to restrict web applications from making requests to domains other than the one that served the original web page. In Go, you can handle cross-origin requests using middleware or HTTP handlers to set appropriate CORS headers.
Here’s how you can handle cross-origin requests in Go:
- Middleware Approach: Use middleware to intercept incoming HTTP requests and add CORS headers to the response before passing it to the next handler in the chain. Middleware functions can inspect the request origin, method, headers, and other attributes to determine the appropriate CORS policy to apply.
- HTTP Handler Approach: Alternatively, you can implement CORS headers directly within individual HTTP handler functions by setting the appropriate response headers based on the request context and configuration settings.
- CORS Headers: The key CORS headers include Access-Control-Allow-Origin, Access-Control-Allow-Methods, Access-Control-Allow-Headers, Access-Control-Allow-Credentials, and Access-Control-Max-Age. These headers specify the allowed origins, HTTP methods, request headers, credentials, and preflight request cache duration for cross-origin requests.
- Options Requests Handling: In addition to handling regular HTTP requests, you should handle preflight OPTIONS requests sent by the browser to determine whether the actual request is safe to send. The server should respond to preflight requests with appropriate CORS headers and status codes to indicate the allowed methods, headers, and origins.
- CORS Middleware Libraries: Consider using third-party CORS middleware libraries for Go, such as github.com/rs/cors or github.com/gin-contrib/cors, which provide pre-configured middleware handlers for handling CORS headers and policies in Go web applications.
- Custom CORS Policy: Define a custom CORS policy that specifies the allowed origins, methods, headers, and other CORS parameters based on your application’s requirements and security considerations. Ensure that the CORS policy is consistent with the security policy of your web application and complies with CORS best practices.
By implementing appropriate CORS headers and policies in Go web applications, you can enable cross-origin requests while maintaining security, integrity, and compliance with web standards and browser security policies.
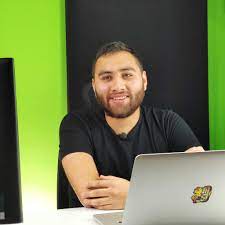
Previously at
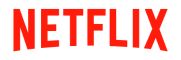
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.