Creating Cross-Platform Mobile Apps with Go and Flutter
In the ever-evolving landscape of mobile app development, cross-platform solutions have gained immense popularity due to their ability to streamline development processes and reach a wider audience. One such powerful combination for creating cross-platform mobile apps is using the Go programming language for the backend and Flutter for the frontend. In this guide, we’ll delve into the fusion of Go and Flutter, exploring how these technologies complement each other and provide a robust platform for building feature-rich and efficient mobile applications.
1. Introduction
1.1. The Rise of Cross-Platform Development
Gone are the days when developers had to choose between building separate apps for each platform – iOS, Android, and sometimes even more. Cross-platform development frameworks have emerged as a game-changer in the industry, allowing developers to write code once and deploy it across multiple platforms. This not only accelerates development but also reduces maintenance efforts and ensures consistent user experiences.
1.2. Go and Flutter: A Perfect Match
Go, also known as Golang, is a statically typed, compiled language developed by Google. It’s known for its simplicity, performance, and excellent concurrency support. On the other hand, Flutter, a UI toolkit from Google, enables developers to create natively compiled applications for mobile, web, and desktop from a single codebase. The synergy between Go’s backend capabilities and Flutter’s elegant UI components makes for an ideal combination in building cross-platform mobile apps.
2. Getting Started
2.1. Setting Up the Development Environment
Before diving into development, you need to set up your development environment. Install Go by downloading the installer for your platform from the official website. Flutter also provides detailed installation instructions on its official website, ensuring you have all the necessary dependencies installed.
2.2. Installing Go and Flutter
Once you have Go and Flutter set up, you can create a new project directory for your mobile app. To create a Go project, use the following commands:
bash mkdir go-flutter-app cd go-flutter-app go mod init github.com/your-username/go-flutter-app
For creating a Flutter project:
bash flutter create flutter_app cd flutter_app
With these initial steps completed, you’re ready to start building your cross-platform mobile app.
3. Building the Backend with Go
The backend serves as the foundation of your mobile app, handling data processing, business logic, and communication with external services. Go’s simplicity and performance make it an excellent choice for backend development.
3.1. Designing RESTful APIs
RESTful APIs provide a structured way for your frontend and backend to communicate. Go makes it easy to create RESTful APIs using packages like “net/http” and “gorilla/mux”. Define routes, handle requests, and send responses seamlessly.
3.2. Handling Data and Logic
Go’s strong typing and structured approach help you manage your data and logic efficiently. Leverage structs for data modeling and interfaces for modular design. With Go’s concurrency support, you can manage multiple tasks simultaneously, enhancing the responsiveness of your app.
3.3. Integrating Database Connectivity
Go offers a variety of libraries to connect to databases. Whether you prefer SQL or NoSQL, you’ll find libraries like “database/sql” and “gorm” that simplify database interactions. This allows you to store and retrieve data securely from your mobile app.
Code Sample: Creating an API Endpoint in Go
go package main import ( "fmt" "net/http" "github.com/gorilla/mux" ) func HelloWorldHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { r := mux.NewRouter() r.HandleFunc("/hello", HelloWorldHandler) http.Handle("/", r) http.ListenAndServe(":8080", nil) }
In this code sample, we create a simple Go HTTP server using the Gorilla Mux router. The server responds with “Hello, World!” when accessed at the “/hello” endpoint.
4. Crafting the Frontend with Flutter
Flutter’s UI toolkit empowers you to create stunning and responsive user interfaces. Its widget-based architecture allows you to compose complex UIs using simple components.
4.1. Building User Interfaces with Widgets
Widgets are the building blocks of a Flutter app. They range from basic elements like buttons and text fields to complex layouts. Combine widgets to create intricate UIs while maintaining a consistent look and feel across platforms.
4.2. Managing State Effectively
State management is crucial for interactive apps. Flutter provides various options for state management, including setState, Provider, Riverpod, and more. Choose the one that suits your app’s complexity and requirements.
4.3. Navigating Between Screens
Most mobile apps consist of multiple screens. Flutter’s Navigator class facilitates screen navigation and transition animations. Push, pop, and manage routes to create a smooth user experience.
4.4. Implementing Responsive Design
Flutter simplifies responsive design by allowing you to adapt layouts based on screen size and orientation. Use media queries and layout builders to ensure your app looks great on various devices.
Code Sample: Creating a Flutter UI Layout
dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter App'), ), body: Center( child: Text('Hello, Flutter!'), ), ), ); } }
In this code sample, we create a simple Flutter app with a centered “Hello, Flutter!” text displayed on the screen.
5. Connecting Frontend and Backend
The magic of cross-platform apps happens when the frontend and backend seamlessly communicate. In this section, we’ll explore how to establish this connection.
5.1. Making HTTP Requests from Flutter to Go
Flutter’s “http” package allows you to send HTTP requests to your Go backend. This enables your app to fetch data, submit forms, and interact with the server.
5.2. Sending and Receiving Data
Send data in JSON format from your Flutter app and parse it in your Go backend. This enables the exchange of structured information between the frontend and backend.
5.3. Error Handling and Validation
Handle errors gracefully by implementing error codes and proper error handling mechanisms. Validate incoming data on both ends to ensure data integrity.
Code Sample: Consuming Go APIs in Flutter
dart import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { Future<void> fetchData() async { final response = await http.get(Uri.parse('http://localhost:8080/hello')); if (response.statusCode == 200) { print(response.body); } else { print('Failed to fetch data'); } } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter App'), ), body: Center( child: ElevatedButton( onPressed: fetchData, child: Text('Fetch Data from Go'), ), ), ), ); } }
In this code sample, pressing the button triggers an HTTP GET request to the Go backend, fetching the “Hello, World!” response.
6. Enhancing User Experience
To create engaging mobile apps, you can enhance the user experience with additional features and functionalities.
6.1. Integrating Third-Party Packages
Both Go and Flutter offer a rich ecosystem of third-party packages. Leverage these packages to integrate features like networking, caching, and more.
6.2. Adding Authentication and Authorization
Secure your app by implementing authentication and authorization mechanisms. Use Go to handle user authentication and Flutter to provide seamless login and registration experiences.
6.3. Utilizing Device Features
Access device features like the camera, GPS, and sensors using Flutter’s plugins. These plugins simplify interaction with hardware components, enhancing your app’s capabilities.
Code Sample: Implementing Authentication in Flutter
dart // Import necessary packages class AuthProvider with ChangeNotifier { String? _token; String? get token => _token; Future<void> login(String username, String password) async { // Send login request to backend // Set _token upon successful login notifyListeners(); } Future<void> logout() async { // Clear token on logout notifyListeners(); } }
In this code sample, an AuthProvider class manages user authentication state. Upon successful login, the token is set, and the UI is updated accordingly.
7. Testing and Debugging
Ensuring the reliability of your app requires thorough testing and effective debugging techniques.
7.1. Unit Testing Go Code
Write unit tests for your Go backend using the built-in testing framework. Test critical components, API endpoints, and business logic to catch bugs early.
7.2. Widget Testing in Flutter
Flutter provides widget testing capabilities to verify the behavior of UI components. Write widget tests to validate UI responsiveness and user interactions.
7.3. Debugging Tips and Tricks
Utilize debugging tools like breakpoints, logs, and Flutter DevTools to identify and resolve issues in both your backend and frontend code.
8. Optimization and Deployment
Optimize your app for performance and deploy it to various platforms.
8.1. Profiling Go Code for Performance
Use Go’s profiling tools to identify bottlenecks and optimize your backend code for better performance.
8.2. Compiling Flutter Code for Various Platforms
Flutter’s compilation process allows you to generate native binaries for iOS, Android, and the web. Compile and test your app on different platforms to ensure consistent behavior.
8.3. Deploying Apps to App Stores
Package your app and submit it to app stores like the Apple App Store and Google Play Store. Follow platform-specific guidelines for a successful submission.
Code Sample: Compiling Flutter App for Android and iOS
bash flutter build apk # For Android flutter build ios # For iOS
Running these commands compiles your Flutter app for Android and iOS platforms respectively.
9. Best Practices
Efficient app development involves following best practices to ensure code quality, collaboration, and security.
9.1. Code Organization and Project Structure
Maintain a clean and organized project structure. Separate frontend and backend codebases for clarity and modularity.
9.2. Version Control and Collaboration
Utilize version control systems like Git to collaborate with team members efficiently. Use branching strategies and commit conventions for seamless collaboration.
9.3. Continuous Integration and Delivery
Set up continuous integration pipelines to automate testing and deployment processes. This ensures that changes are thoroughly tested before being deployed.
9.4. Ensuring Security in Go and Flutter Apps
Follow security best practices to safeguard your app against vulnerabilities. Implement secure authentication mechanisms, sanitize user inputs, and keep dependencies updated.
Conclusion
The combination of Go and Flutter offers a powerful platform for building cross-platform mobile apps. Go’s robust backend capabilities and Flutter’s elegant UI components create a synergy that enables developers to create feature-rich and performant applications. As the world of mobile app development continues to evolve, embracing this fusion of technologies can streamline your development process and position your apps for success in a competitive market. By following the practices outlined in this guide and staying updated with the latest advancements, you can harness the power of Go and Flutter to deliver innovative and user-friendly mobile experiences.
Table of Contents
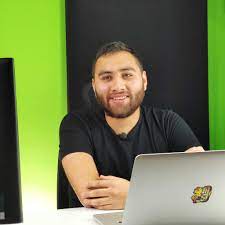
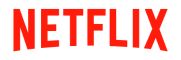