Can you define custom error types in Go?
Yes, Go allows developers to define custom error types to represent specific error conditions within their applications. Custom error types in Go are simply structs that implement the error interface, which consists of a single method, Error() string, that returns a human-readable string describing the error.
Defining custom error types in Go offers several benefits:
Semantic Clarity: Custom error types enable developers to define error conditions that are meaningful and relevant to their application domain. By using descriptive error types, developers can communicate the nature of errors more effectively and provide better context for error handling and troubleshooting.
Error Wrapping: Go’s error handling mechanism allows errors to be wrapped and annotated with additional information as they propagate through the call stack. Custom error types can encapsulate relevant context and metadata along with the original error, making it easier to diagnose and handle errors at different layers of the application.
Type Assertions: Custom error types allow for type assertions, enabling developers to inspect and differentiate between different error types in a type-safe manner. This enables fine-grained error handling logic and facilitates the implementation of error recovery strategies tailored to specific error conditions.
Here’s an example demonstrating how to define and use a custom error type in Go:
go package main import ( "fmt" ) // CustomError represents a custom error type type CustomError struct { Code int Message string } // Error returns the string representation of the error func (e *CustomError) Error() string { return fmt.Sprintf("Error %d: %s", e.Code, e.Message) } func main() { err := &CustomError{ Code: 500, Message: "Internal Server Error", } fmt.Println(err.Error()) }
In this example, we define a custom error type CustomError that encapsulates an error code and a message. The Error() method of the CustomError struct implements the error interface, allowing instances of CustomError to be treated as errors.
By defining custom error types, developers can create a clear and consistent error handling strategy that aligns with the requirements and constraints of their applications.
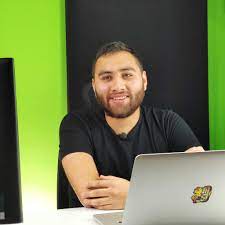
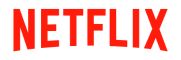