Go for Data Visualization: Creating Charts and Graphs
In today’s data-driven world, the ability to present data effectively is a valuable skill. Data visualization, in the form of charts and graphs, can turn raw data into meaningful insights, making it easier for decision-makers to understand and act upon information. Whether you’re a business analyst, data scientist, or simply someone interested in conveying data more clearly, this guide will take you on a journey through the world of data visualization.
1. Why Data Visualization Matters
1.1. Making Complex Data Accessible
Data can be overwhelming, especially when dealing with large datasets. Raw numbers and text can be difficult to digest and comprehend. However, when you transform this data into visual representations, patterns and trends become easier to identify. Charts and graphs serve as a bridge between the data and the human brain, making complex information accessible to a broader audience.
1.2. Enhancing Decision-Making
Effective data visualization can significantly impact decision-making processes. Whether it’s optimizing marketing campaigns, improving operational efficiency, or spotting market trends, data-driven decisions are more precise and informed when supported by visual data representations. Charts and graphs allow decision-makers to grasp key insights quickly and act accordingly.
1.3. Engaging Your Audience
Whether you’re presenting to colleagues, clients, or the public, engaging visuals can capture and maintain your audience’s attention. A well-designed chart or graph can convey your message more effectively than a dense spreadsheet or a lengthy report. It simplifies complex information, making it easier for your audience to connect with and understand your data.
2. Types of Data Visualizations
2.1. Bar Charts
Bar charts are a common choice for displaying categorical data. They consist of horizontal or vertical bars, where the length or height of each bar represents a specific value. Here’s a simple Python code snippet to create a bar chart using the Matplotlib library:
python import matplotlib.pyplot as plt categories = ['Category A', 'Category B', 'Category C', 'Category D'] values = [10, 25, 15, 30] plt.bar(categories, values) plt.xlabel('Categories') plt.ylabel('Values') plt.title('Bar Chart Example') plt.show()
2.2. Line Charts
Line charts are ideal for showing trends over time. They connect data points with lines, making it easy to see how values change. Here’s an example of creating a line chart with Matplotlib:
python import matplotlib.pyplot as plt months = ['Jan', 'Feb', 'Mar', 'Apr', 'May'] sales = [1000, 1200, 800, 1500, 2000] plt.plot(months, sales, marker='o') plt.xlabel('Months') plt.ylabel('Sales') plt.title('Monthly Sales Trend') plt.grid(True) plt.show()
2.3. Pie Charts
Pie charts are great for illustrating the composition of a whole. They divide a circle into sectors, where each sector represents a different category or proportion. Here’s how to create a simple pie chart using Matplotlib:
python import matplotlib.pyplot as plt categories = ['Category A', 'Category B', 'Category C', 'Category D'] sizes = [25, 30, 15, 30] plt.pie(sizes, labels=categories, autopct='%1.1f%%') plt.title('Pie Chart Example') plt.axis('equal') plt.show()
2.4. Scatter Plots
Scatter plots are useful for visualizing the relationship between two continuous variables. Each point on the plot represents an individual data point, making it easy to spot correlations or outliers. Here’s a Python code snippet to create a scatter plot:
python import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [10, 12, 8, 15, 20] plt.scatter(x, y) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Scatter Plot Example') plt.grid(True) plt.show()
2.5. Histograms
Histograms are excellent for showing the distribution of a dataset. They group data into bins and display the frequency of values within each bin. Here’s an example of creating a histogram with Matplotlib:
python import matplotlib.pyplot as plt data = [20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70] plt.hist(data, bins=5, edgecolor='k') plt.xlabel('Value Range') plt.ylabel('Frequency') plt.title('Histogram Example') plt.show()
3. Choosing the Right Visualization
Selecting the appropriate visualization for your data is crucial. Consider the following factors:
- Data Type: Is your data categorical or numerical? Different visualizations are suitable for different data types.
- Message: What message are you trying to convey? Do you want to show trends, comparisons, or distributions?
- Audience: Who will be viewing your visualization? Ensure it’s tailored to their level of expertise and needs.
- Clarity: Is your visualization easy to understand? Avoid clutter and choose a format that best represents your data.
4. Tools for Data Visualization
Several tools and libraries can help you create stunning data visualizations:
4.1. Matplotlib
Matplotlib is a widely used Python library for creating static, animated, and interactive visualizations. It provides a high level of customization, allowing you to create a wide range of charts and graphs.
4.2. Seaborn
Seaborn is built on top of Matplotlib and offers a higher-level interface for creating stylish and informative statistical graphics. It simplifies the process of creating complex visualizations.
4.3. Plotly
Plotly is an interactive graphing library for Python, R, and JavaScript. It’s particularly well-suited for creating interactive web-based visualizations with features like zoom, hover, and click events.
4.4. Tableau
Tableau is a powerful data visualization tool that allows you to create interactive and shareable dashboards without extensive coding. It’s user-friendly and suitable for both beginners and advanced users.
4.5. Power BI
Power BI is a Microsoft product that offers robust data visualization capabilities. It’s especially useful for businesses and organizations looking to create data-driven reports and dashboards.
5. Best Practices for Effective Data Visualization
5.1. Keep it Simple
Simplicity is key to effective data visualization. Avoid clutter, excessive decorations, and unnecessary elements that can distract from the message you’re trying to convey.
5.2. Label Clearly
Always label your axes, data points, and legends clearly. Ensure that anyone looking at your visualization can understand it without ambiguity.
5.3. Choose the Right Colors
Colors should enhance, not confuse, your visualization. Use color schemes that are easy on the eyes and consider colorblind users.
5.4. Use Interactivity Wisely
Interactive elements can engage your audience, but don’t overdo it. Use interactivity to provide additional details or insights, not to overwhelm.
5.5. Tell a Story
Every visualization should tell a story. Start with a clear narrative and guide your audience through the data to draw meaningful conclusions.
Case Study: Sales Performance Dashboard
Let’s put our knowledge to work by creating a simple sales performance dashboard using Python and Plotly. This dashboard will include a bar chart to display monthly sales and a pie chart to show the distribution of products sold.
python import plotly.express as px # Sample data months = ['Jan', 'Feb', 'Mar', 'Apr', 'May'] sales = [1000, 1200, 800, 1500, 2000] products = ['Product A', 'Product B', 'Product C', 'Product D'] product_sales = [30, 25, 20, 25] # Create a bar chart for monthly sales fig = px.bar(x=months, y=sales, labels={'x':'Months', 'y':'Sales'}, title='Monthly Sales Performance') fig.show() # Create a pie chart for product distribution fig = px.pie(names=products, values=product_sales, title='Product Sales Distribution') fig.show()
Conclusion
Data visualization is a powerful tool that can transform raw data into actionable insights. Whether you’re a data professional or someone looking to make data-driven decisions, mastering the art of creating charts and graphs is a valuable skill. Remember to choose the right visualization for your data, use appropriate tools, and follow best practices to effectively convey your message. With practice and creativity, you can create impactful data visualizations that drive better decision-making and engage your audience. Start exploring the world of data visualization today and unlock the potential of your data.
Now that you’ve learned the basics of data visualization, it’s time to put your skills to the test and start turning your data into compelling visuals. Happy charting!
Table of Contents
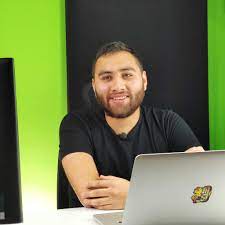
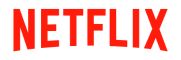