Debugging Go Applications: Tools and Techniques for Efficient Debugging
Bugs are an inevitable part of software development. No matter how skilled a programmer you are, you’ll encounter them sooner or later. Debugging is the process of identifying and resolving bugs in your code to ensure that your application functions as intended. When it comes to debugging Go applications, you’re in luck. Go, also known as Golang, provides a powerful set of tools and techniques that can help you debug your code efficiently and effectively.
1. Why Debugging Matters
Debugging is an essential skill for any developer. It’s not just about fixing issues in your code; it’s also about understanding the logic of your program and improving its performance. Proper debugging can save you a considerable amount of time and frustration, as well as prevent potential problems for your users. A well-debugged application is more reliable, maintainable, and secure.
2. Common Debugging Challenges in Go
Before diving into the tools and techniques, let’s briefly discuss some common debugging challenges you might face when working with Go applications:
2.1. Concurrency Bugs
Go’s strong suit is concurrent programming, but it can also lead to complex concurrency bugs. These bugs can be tricky to reproduce and debug due to the inherent nature of concurrent execution.
2.2. Memory Leaks
Go has a garbage collector that manages memory, but memory leaks can still occur if references aren’t managed correctly. Identifying the source of a memory leak can be challenging.
2.3. Panics
A panic in Go is similar to an exception in other languages. Identifying the root cause of a panic and preventing it from crashing your application is crucial.
3. Essential Tools for Debugging Go Applications
Go provides a variety of tools that can assist you in debugging your applications. Let’s explore some of the essential tools that should be in your debugging toolbox:
3.1. fmt Package
The fmt package is your best friend when it comes to quick and dirty debugging. Use functions like fmt.Printf and fmt.Println to print out variables, messages, and other relevant information to the console. This is a simple yet effective way to get insight into what’s happening in your code.
go package main import "fmt" func main() { name := "Alice" age := 30 fmt.Printf("Name: %s, Age: %d\n", name, age) }
3.2. GDB (GNU Debugger)
GDB is a powerful debugger that can be used with Go applications. It allows you to set breakpoints, inspect variables, and step through your code. To use GDB with Go, you’ll need to install the delve debugger, which provides a Go-compatible interface to GDB.
3.3. Delve
Delve is a debugger specifically designed for Go. It’s a fantastic tool for debugging Go applications, as it provides features like interactive debugging, attaching to running processes, and examining goroutines.
To install Delve, use the following command:
bash go get github.com/go-delve/delve/cmd/dlv
Once installed, you can use it to start debugging your Go code:
bash dlv debug
3.4. pprof
Performance profiling is an essential aspect of debugging. Go’s built-in pprof package allows you to analyze CPU and memory usage, helping you identify bottlenecks and memory-related issues in your application.
go package main import ( _ "net/http/pprof" "net/http" ) func main() { go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() // Your application code here }
Access the pprof web interface by navigating to http://localhost:6060/debug/pprof/ in your browser.
4. Effective Debugging Techniques
Having the right tools is essential, but knowing how to use them effectively is just as important. Here are some techniques to help you debug your Go applications more efficiently:
4.1. Start with a Reproducible Case
Before you start debugging, make sure you can reproduce the issue consistently. Write test cases that trigger the problem reliably. This will save you time in the long run and help you verify when you’ve successfully fixed the bug.
4.2. Use Version Control
Version control, such as Git, can be a lifesaver when debugging. Create a new branch for debugging purposes, so you can experiment without affecting the main codebase. If your debugging attempts lead to a dead-end, you can easily discard the branch.
4.3. Isolate the Problem
Narrow down the problem by identifying the smallest piece of code that exhibits the issue. This will help you isolate the root cause and reduce the scope of your debugging efforts.
4.4. Rubber Duck Debugging
Explaining the problem to someone else (or even an inanimate object like a rubber duck) can help you clarify your thoughts. Often, the act of explaining the issue can lead you to spot the mistake yourself.
Conclusion
Debugging is an essential skill for every developer, and Go provides a robust set of tools and techniques to help you tackle bugs effectively. From basic fmt package usage to more advanced tools like Delve and pprof, you have a range of options to choose from. Remember that effective debugging isn’t just about tools; it’s about adopting a systematic approach, isolating issues, and staying patient until you identify and squash those bugs. Happy debugging!
Table of Contents
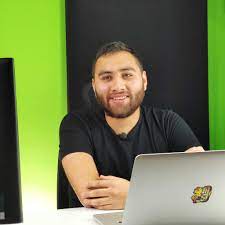
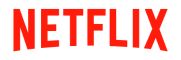