Working with Encryption and Hashing in Go: Protecting Sensitive Data
In today’s digital landscape, data security is of paramount importance. From personal information to financial records, sensitive data is exchanged and stored online every day. Ensuring the confidentiality and integrity of this information is crucial to prevent unauthorized access and potential breaches. In this article, we’ll explore how to use encryption and hashing techniques in Go to protect sensitive data, along with code examples and best practices.
1. Understanding Encryption and Hashing
Encryption and hashing are two fundamental techniques employed to secure data, but they serve different purposes:
- Encryption: Encryption involves transforming data into a format that can only be deciphered by someone who possesses the decryption key. This ensures that even if unauthorized individuals gain access to the encrypted data, they cannot make sense of it without the proper key.
- Hashing: Hashing, on the other hand, is a one-way process that converts data into a fixed-size string of characters. It’s designed to be irreversible, meaning you can’t convert the hash back to its original data. Hashing is commonly used for data verification and password storage.
2. Implementing Encryption in Go
When working with sensitive data, encrypting it before storage or transmission is crucial. Go provides a robust standard library that supports various encryption algorithms. Let’s see how to encrypt and decrypt data using the Advanced Encryption Standard (AES) algorithm.
2.1. Generating Encryption Keys
Before you can encrypt or decrypt data using AES, you need an encryption key. Here’s an example of generating a random AES key:
go package main import ( "crypto/aes" "crypto/rand" "fmt" ) func generateAESKey() ([]byte, error) { key := make([]byte, 32) // AES-256 requires a 32-byte key _, err := rand.Read(key) if err != nil { return nil, err } return key, nil } func main() { key, err := generateAESKey() if err != nil { fmt.Println("Error generating AES key:", err) return } fmt.Printf("Generated AES key: %x\n", key) }
3. Encrypting and Decrypting Data
Now that we have an AES key, let’s see how to use it for encrypting and decrypting data:
go package main import ( "crypto/aes" "crypto/cipher" "crypto/rand" "fmt" "io" ) func encryptAES(data []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } // Using Galois Counter Mode (GCM) for authenticated encryption gcm, err := cipher.NewGCM(block) if err != nil { return nil, err } nonce := make([]byte, gcm.NonceSize()) if _, err := io.ReadFull(rand.Reader, nonce); err != nil { return nil, err } ciphertext := gcm.Seal(nil, nonce, data, nil) return ciphertext, nil } func decryptAES(ciphertext []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } gcm, err := cipher.NewGCM(block) if err != nil { return nil, err } nonceSize := gcm.NonceSize() if len(ciphertext) < nonceSize { return nil, fmt.Errorf("ciphertext is too short") } nonce, ciphertext := ciphertext[:nonceSize], ciphertext[nonceSize:] plaintext, err := gcm.Open(nil, nonce, ciphertext, nil) if err != nil { return nil, err } return plaintext, nil } func main() { key, err := generateAESKey() if err != nil { fmt.Println("Error generating AES key:", err) return } data := []byte("Sensitive data to be encrypted") ciphertext, err := encryptAES(data, key) if err != nil { fmt.Println("Error encrypting data:", err) return } fmt.Printf("Ciphertext: %x\n", ciphertext) decryptedData, err := decryptAES(ciphertext, key) if err != nil { fmt.Println("Error decrypting data:", err) return } fmt.Println("Decrypted data:", string(decryptedData)) }
In this example, we’ve used the AES-GCM mode for authenticated encryption. This ensures the confidentiality and integrity of the data. Remember that the key should be securely stored and managed, as it’s the cornerstone of the encryption process.
4. Utilizing Hashing for Data Integrity
Hashing is essential for verifying the integrity of data. It’s commonly used to create digital signatures, verify data transmission, and store passwords securely. Let’s see how to hash data using SHA-256 in Go.
4.1. Hashing Data
Here’s an example of hashing data using the SHA-256 algorithm:
go package main import ( "crypto/sha256" "encoding/hex" "fmt" ) func hashSHA256(data []byte) string { hash := sha256.Sum256(data) return hex.EncodeToString(hash[:]) } func main() { data := []byte("Data to be hashed") hashedData := hashSHA256(data) fmt.Println("SHA-256 Hash:", hashedData) }
4.2. Salting for Password Hashing
When it comes to password hashing, salting is an essential technique. Salting involves adding a unique value to each password before hashing, which ensures that even if two users have the same password, their hashed values will differ due to the unique salt.
go package main import ( "crypto/rand" "crypto/sha256" "encoding/hex" "fmt" ) func generateSalt(length int) ([]byte, error) { salt := make([]byte, length) _, err := rand.Read(salt) if err != nil { return nil, err } return salt, nil } func hashPassword(password string, salt []byte) string { passwordBytes := []byte(password) combined := append(passwordBytes, salt...) hash := sha256.Sum256(combined) return hex.EncodeToString(hash[:]) } func main() { password := "securePassword123" salt, err := generateSalt(16) if err != nil { fmt.Println("Error generating salt:", err) return } hashedPassword := hashPassword(password, salt) fmt.Println("Hashed Password:", hashedPassword) fmt.Println("Salt:", hex.EncodeToString(salt)) }
5. Best Practices for Secure Data Handling
While encryption and hashing are powerful tools for protecting sensitive data, it’s essential to follow best practices to ensure maximum security:
- Use Strong Algorithms: Always choose well-established and secure encryption and hashing algorithms. Avoid using outdated or weak algorithms.
- Protect Encryption Keys: Store encryption keys securely, ideally in a hardware security module (HSM) or a secure key management service.
- Secure Salt Management: When using salting for password hashing, ensure that each user has a unique salt. Store salts alongside the hashed passwords, and never use a static or common salt.
- Key Rotation: Regularly rotate encryption keys to minimize the potential impact of a breach. This practice adds an extra layer of security to your encrypted data.
- Use Authenticated Encryption: For encryption, opt for modes like AES-GCM that provide both confidentiality and integrity checks.
- Hash Passwords with a Purpose-built Library: When handling passwords, use a purpose-built library for password hashing, like the “golang.org/x/crypto/bcrypt” package, which incorporates salting and multiple rounds of hashing.
- Implement Proper Access Controls: Limit access to sensitive data and cryptographic operations to authorized personnel only.
- Audit and Monitoring: Implement logging and monitoring for cryptographic operations to detect any unauthorized or suspicious activities.
Conclusion
In a world where data breaches have become a common occurrence, safeguarding sensitive information is not optional; it’s a necessity. Encryption and hashing are invaluable tools in your arsenal to ensure data confidentiality, integrity, and security. Go’s strong standard library and community-contributed packages make it relatively straightforward to implement encryption and hashing techniques in your applications. By following best practices and staying up-to-date with the latest security recommendations, you can bolster the protection of your users’ sensitive data and build a safer digital environment for everyone.
Table of Contents
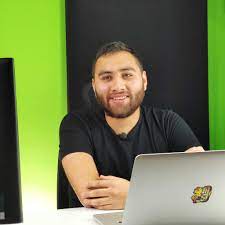
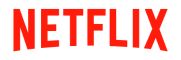