How is error handling done in Go?
Error handling in Go is based on the principle of explicit error checking and propagation. Go provides a built-in error type, error, which is an interface with a single method Error() string. Functions and methods in Go often return error values to indicate success or failure of an operation.
In Go, error handling typically involves calling a function or method and checking the error returned by it. If the error is not nil, it indicates that the operation failed, and appropriate error handling logic should be executed. Here’s an example of error handling in Go:
go file, err := os.Open("filename.txt") if err != nil { // Handle error log.Fatal(err) } defer file.Close()
In this example, we attempt to open a file named “filename.txt” using the os.Open() function. If the err variable is not nil, it means that an error occurred during the file opening operation. We handle the error by logging it using log.Fatal() and terminating the program.
Go also provides the defer statement, which is commonly used for cleanup actions such as closing files and releasing resources. Deferred function calls are executed just before the surrounding function returns, regardless of whether the function exits normally or panics.
Additionally, Go allows for custom error types by implementing the error interface. Custom error types can provide additional context and information about the error condition, enhancing the clarity and expressiveness of error messages in Go programs.
Error handling in Go promotes explicitness, simplicity, and reliability, making it easier to reason about the behavior of programs and handle unexpected conditions gracefully.
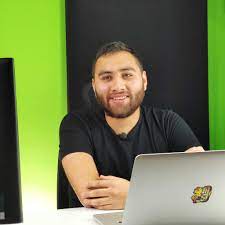
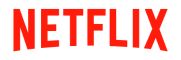