Efficient File Handling in Go: Reading, Writing, and Manipulating Files
File handling is a fundamental aspect of software development, allowing programs to interact with data stored on disk. In the world of Go programming, a language known for its simplicity and performance, efficient file handling is crucial for building robust and high-performance applications. In this blog post, we will dive into the intricacies of handling files in Go, covering techniques for reading, writing, and manipulating files while keeping performance in mind. Let’s explore the world of file handling in Go step by step.
1. Reading Files
When it comes to reading files in Go, the os package provides the necessary functionalities. Let’s explore the process step by step.
1.1. Opening a File
Before you can read the contents of a file, you need to open it. The os.Open function is used for this purpose. Here’s an example:
go package main import ( "fmt" "os" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Make sure to close the file when done // Read file contents here }
1.2. Reading File Contents
Once the file is open, you can read its contents using the Read method from the os.File type. Here’s how you can read the entire content of the file:
go package main import ( "fmt" "os" "io/ioutil" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() content, err := ioutil.ReadAll(file) if err != nil { fmt.Println("Error:", err) return } fmt.Println("File contents:", string(content)) }
1.3. Buffered Reading
To optimize reading large files, you can use a buffered approach. This involves using a bufio.Reader to read the file in chunks, reducing the number of system calls. Here’s an example:
go package main import ( "fmt" "os" "bufio" ) func main() { file, err := os.Open("large_file.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() reader := bufio.NewReader(file) buffer := make([]byte, 1024) // Read in chunks of 1KB for { n, err := reader.Read(buffer) if err != nil { break } fmt.Print(string(buffer[:n])) } }
2. Writing Files
Writing files in Go is just as important as reading them. The os package provides functionalities for creating, opening, and writing to files.
2.1. Creating and Opening a File for Writing
To create a new file or overwrite an existing one for writing, you can use the os.Create or os.OpenFile functions. Here’s an example using os.Create:
go package main import ( "fmt" "os" ) func main() { file, err := os.Create("output.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Write content to the file here }
2.2. Writing Text and Bytes
You can write text or byte slices to a file using the WriteString and Write methods of the os.File type, respectively. Here’s an example:
go package main import ( "fmt" "os" ) func main() { file, err := os.Create("output.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() content := "Hello, world!" _, err = file.WriteString(content) if err != nil { fmt.Println("Error:", err) return } }
2.3. Buffered Writing
Similar to reading, buffered writing can improve performance when dealing with large amounts of data. You can achieve this using the bufio.Writer type. Here’s an example:
go package main import ( "fmt" "os" "bufio" ) func main() { file, err := os.Create("large_output.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() writer := bufio.NewWriter(file) content := "A large amount of data..." _, err = writer.WriteString(content) if err != nil { fmt.Println("Error:", err) return } writer.Flush() // Flush the buffer to write data to the file }
3. Manipulating Files
In addition to reading and writing, Go provides tools for file manipulation, such as renaming, moving, and deleting files.
3.1. Renaming and Moving Files
The os.Rename function allows you to rename or move a file. Here’s an example of renaming a file:
go package main import ( "fmt" "os" ) func main() { err := os.Rename("old_file.txt", "new_file.txt") if err != nil { fmt.Println("Error:", err) return } }
3.2. Deleting Files
To delete a file, you can use the os.Remove function:
go package main import ( "fmt" "os" ) func main() { err := os.Remove("file_to_delete.txt") if err != nil { fmt.Println("Error:", err) return } }
3.3. Checking File Existence
Before performing file manipulation operations, it’s a good idea to check if a file exists. The os.Stat function can be used for this purpose:
go package main import ( "fmt" "os" ) func main() { _, err := os.Stat("file_to_check.txt") if os.IsNotExist(err) { fmt.Println("File does not exist") } else if err != nil { fmt.Println("Error:", err) return } fmt.Println("File exists") }
4. Efficiency Considerations
While working with files in Go, it’s important to consider efficiency to ensure optimal performance.
4.1. Using Buffers
We’ve already seen how using buffered reading and writing can improve performance when dealing with large files. Buffers reduce the number of system calls by reading and writing data in chunks.
4.2. Error Handling
File operations can result in errors, such as files not being found or permission issues. Always handle errors appropriately to prevent unexpected crashes and ensure graceful degradation.
4.3. Defer Statements
In Go, defer statements can be used to ensure that certain actions, like closing files, are performed even if an error occurs. This can help prevent resource leaks.
go package main import ( "fmt" "os" ) func main() { file, err := os.Open("example.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Will be executed when the function exits // Read file contents here }
Conclusion
Efficient file handling is a crucial aspect of Go programming, enabling you to build robust and high-performance applications. In this blog post, we’ve explored techniques for reading, writing, and manipulating files in Go, covering everything from opening files to using buffers for optimized operations. By keeping efficiency considerations in mind and following best practices, you can ensure that your file handling code is both effective and performant. Happy coding with Go’s powerful file handling capabilities!
Table of Contents
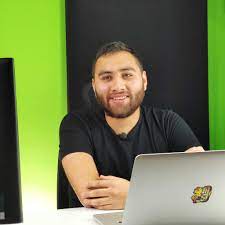
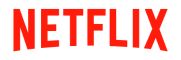