Go for Financial Applications: Handling Currency and Transactions
In today’s fast-paced world, financial applications play a pivotal role in managing our money and investments. Whether it’s online banking, stock trading, or budgeting tools, these applications need to handle currency and transactions accurately and securely. This blog post will delve into the powerful programming language, Go (also known as Golang), and how it can be a game-changer when developing financial applications. We will explore the fundamental concepts of handling currency and transactions, complete with code samples and practical insights.
1. Why Choose Go for Financial Applications?
1.1. Robust and Efficient
Go is renowned for its simplicity, efficiency, and reliability. These qualities make it an ideal choice for developing financial applications that require high performance and precision. Go’s built-in concurrency features also enable developers to create applications that can handle multiple transactions concurrently without compromising on stability.
1.2. Cross-Platform Compatibility
Financial applications often need to run seamlessly on various platforms, including web browsers, mobile devices, and desktops. Go’s cross-platform compatibility ensures that your code can be easily ported to different environments without extensive modification, saving you time and resources.
1.3. Strong Community Support
The Go community is vibrant and ever-growing, which means you can find a wealth of resources, libraries, and tools to accelerate your financial application development. From currency conversion libraries to cryptographic packages for securing transactions, the Go ecosystem has you covered.
1.4. Safety and Security
When dealing with sensitive financial data, security is paramount. Go’s strong typing system, memory safety, and support for concurrent programming help developers write code that is less prone to common vulnerabilities like buffer overflows and race conditions.
2. Handling Currency in Go
One of the core aspects of financial applications is dealing with different currencies. Currency handling requires precision, accuracy, and the ability to perform conversions between various currency units. Let’s explore how Go can assist in handling currency effectively.
2.1. Working with Decimal Arithmetic
When dealing with currency, floating-point arithmetic can lead to precision issues due to the inherent limitations of binary floating-point numbers. Go provides the decimal package, which allows you to perform decimal arithmetic with arbitrary precision. Here’s a code sample illustrating how to use the decimal package to add two currency values:
go package main import ( "fmt" "github.com/shopspring/decimal" ) func main() { // Create decimal values for currency amounts amount1 := decimal.NewFromFloat(19.99) amount2 := decimal.NewFromFloat(9.95) // Add the currency values total := amount1.Add(amount2) // Print the result fmt.Printf("Total: %s\n", total) }
In this example, we import the decimal package and use it to create decimal values for currency amounts. We then perform addition with arbitrary precision, ensuring that the result is accurate.
2.2. Currency Conversion
Financial applications often require currency conversion to support transactions involving different currencies. Go offers libraries like exchangeratesapi.io, which allows you to fetch and perform currency conversion rates easily. Here’s a code snippet demonstrating how to use this library to convert currency:
go package main import ( "fmt" "log" "net/http" "io/ioutil" "encoding/json" "github.com/shopspring/decimal" ) type ExchangeRate struct { Base string `json:"base"` Rates map[string]float64 `json:"rates"` } func main() { // Specify the base currency and target currency baseCurrency := "USD" targetCurrency := "EUR" // Fetch the latest exchange rates resp, err := http.Get(fmt.Sprintf("https://api.exchangeratesapi.io/latest?base=%s", baseCurrency)) if err != nil { log.Fatal(err) } defer resp.Body.Close() body, err := ioutil.ReadAll(resp.Body) if err != nil { log.Fatal(err) } // Unmarshal the JSON response var exchangeRate ExchangeRate if err := json.Unmarshal(body, &exchangeRate); err != nil { log.Fatal(err) } // Get the conversion rate conversionRate := exchangeRate.Rates[targetCurrency] // Convert currency amountInUSD := decimal.NewFromFloat(100.0) amountInTargetCurrency := amountInUSD.Mul(decimal.NewFromFloat(conversionRate)) fmt.Printf("%s %s is equivalent to %s %s\n", amountInUSD, baseCurrency, amountInTargetCurrency, targetCurrency) }
In this code, we make an API request to retrieve the latest exchange rates using the exchangeratesapi.io service. We then perform the currency conversion by multiplying the amount in the base currency by the conversion rate.
2.3. Formatting Currency
Properly formatting currency values is crucial in financial applications to ensure readability and compliance with regional standards. Go provides the currency package, which aids in formatting currency values according to specific locales. Here’s an example:
go package main import ( "fmt" "github.com/leekchan/accounting" ) func main() { // Create an accounting object for formatting ac := accounting.Accounting{Symbol: "$", Precision: 2} // Format a currency value formattedAmount := ac.FormatMoney(1234.56) fmt.Println("Formatted Amount:", formattedAmount) }
In this example, we use the accounting package to format a currency value with a specific currency symbol and precision.
3. Transaction Handling in Go
Transaction handling is another critical aspect of financial applications. Transactions need to be recorded, validated, and executed accurately to ensure the integrity of financial data. Let’s see how Go simplifies transaction handling.
3.1. Database Transactions
Many financial applications use databases to store transaction data. Go provides excellent support for working with databases, and you can easily manage database transactions using the database/sql package. Here’s a basic example of database transaction handling in Go:
go package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { // Open a database connection db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/mydb") if err != nil { log.Fatal(err) } defer db.Close() // Begin a transaction tx, err := db.Begin() if err != nil { log.Fatal(err) } // Execute SQL statements within the transaction _, err = tx.Exec("INSERT INTO transactions (amount, description) VALUES (?, ?)", 100.0, "Purchase") if err != nil { // Rollback the transaction in case of an error tx.Rollback() log.Fatal(err) } // Commit the transaction err = tx.Commit() if err != nil { log.Fatal(err) } fmt.Println("Transaction completed successfully") }
In this code, we open a database connection, begin a transaction, execute SQL statements within the transaction, and then either commit or rollback the transaction based on the success or failure of the operations.
3.2. Error Handling and Rollbacks
Robust error handling and the ability to roll back transactions are critical in financial applications to ensure data consistency. Go makes it easy to handle errors and roll back transactions in case of failures, as demonstrated in the previous example.
3.3. Distributed Transactions
For large-scale financial applications that operate across multiple services or microservices, distributed transactions may be necessary. Go provides libraries like go-tx that facilitate distributed transaction management. Here’s a simplified example of distributed transaction handling:
go package main import ( "context" "fmt" "github.com/jackc/pgx/v4" "github.com/jackc/pgx/v4/stdlib" "github.com/kardianos/service" ) func main() { // Connect to multiple databases dbConfig1 := "postgres://user1:password1@localhost:5432/database1" dbConfig2 := "postgres://user2:password2@localhost:5432/database2" conn1, err := pgx.Connect(context.Background(), dbConfig1) if err != nil { fmt.Println("Error connecting to database 1:", err) return } defer conn1.Close() conn2, err := pgx.Connect(context.Background(), dbConfig2) if err != nil { fmt.Println("Error connecting to database 2:", err) return } defer conn2.Close() // Begin a distributed transaction tx, err := conn1.Begin(context.Background()) if err != nil { fmt.Println("Error beginning transaction:", err) return } defer tx.Rollback(context.Background()) // Execute SQL statements in the first database _, err = tx.Exec(context.Background(), "INSERT INTO account1 (amount) VALUES ($1)", 100.0) if err != nil { fmt.Println("Error executing SQL in database 1:", err) return } // Execute SQL statements in the second database _, err = conn2.Exec(context.Background(), "INSERT INTO account2 (amount) VALUES ($1)", 100.0) if err != nil { fmt.Println("Error executing SQL in database 2:", err) return } // Commit the distributed transaction err = tx.Commit(context.Background()) if err != nil { fmt.Println("Error committing transaction:", err) return } fmt.Println("Distributed transaction completed successfully") }
In this example, we connect to two different databases and use a distributed transaction to perform operations across both databases. The ability to coordinate and commit or roll back distributed transactions is crucial in complex financial applications.
Conclusion
Developing financial applications that handle currency and transactions effectively is a challenging but rewarding endeavor. Go, with its simplicity, efficiency, and robustness, is an excellent choice for building such applications. In this blog post, we’ve explored how Go can assist in handling currency with decimal arithmetic, currency conversion, and formatting. We’ve also seen how Go simplifies transaction handling with features like database transactions, error handling, rollbacks, and distributed transactions.
Whether you’re building a personal finance app, a trading platform, or a banking system, Go provides the tools and libraries you need to ensure the accuracy, security, and efficiency of your financial application. As you embark on your financial application development journey, remember to leverage the power of Go to create software that meets the highest standards of reliability and performance in the financial industry.
Financial applications are a critical part of our lives, and they demand the utmost precision and security. With Go as your programming language of choice, you can build applications that not only meet these demands but also provide a seamless user experience. So, dive into the world of Go for financial applications and take your software development skills to new heights in the realm of finance.
Table of Contents
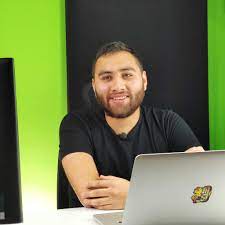
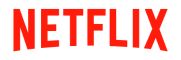