Go for Game Development: Building Games with the Ebiten Library
Are you a budding game developer looking to create exciting, cross-platform games with ease? Look no further than Ebiten, a powerful 2D game library for the Go programming language. In this blog, we’ll explore how Ebiten can help you unleash your creativity and build engaging games. From setting up your development environment to creating game mechanics and rendering graphics, we’ve got you covered.
1. Why Ebiten?
Before we dive into the nitty-gritty of game development with Ebiten, let’s understand why it’s a fantastic choice for aspiring game developers.
1.1. Simplicity and Ease of Use
Ebiten is designed with simplicity in mind. If you’re familiar with Go, you’ll appreciate how easy it is to get started. The library provides a straightforward API for handling input, rendering graphics, and managing game loops, making it perfect for beginners.
1.2. Cross-Platform Compatibility
One of Ebiten’s most significant advantages is its cross-platform support. You can develop games on Windows, macOS, Linux, and even browsers using WebAssembly. This means your games can reach a broad audience without much additional effort.
1.3. Performance
Ebiten is optimized for performance, thanks to its efficient rendering pipeline. It uses hardware acceleration to ensure your games run smoothly, even on less powerful devices.
1.4. Active Community and Resources
Being an open-source project, Ebiten has a thriving community that actively contributes to its development. You’ll find plenty of tutorials, documentation, and community support to help you along your game development journey.
2. Getting Started with Ebiten
Now that you know why Ebiten is a fantastic choice for game development, let’s roll up our sleeves and get started. Follow these steps to set up your development environment.
2.1. Install Go
If you haven’t already, download and install the Go programming language from the official website. Ebiten relies on Go, so make sure you have it up and running.
2.2. Create a New Project
Open your terminal and create a new directory for your game project. Inside the project directory, initialize a Go module.
bash mkdir mygame cd mygame go mod init mygame
2.3. Install Ebiten
To start using Ebiten, you need to install it. In your terminal, run the following command:
bash go get github.com/hajimehoshi/ebiten/v2
This command fetches the Ebiten library and its dependencies.
2.4. Writing Your First Game
With Ebiten installed, it’s time to write your first game. Create a new Go file, let’s call it main.go, and add the following code:
go package main import ( "fmt" "log" "github.com/hajimehoshi/ebiten/v2" ) const ( screenWidth = 640 screenHeight = 480 ) var ( gopher *ebiten.Image ) func update(screen *ebiten.Image) error { if ebiten.IsDrawingSkipped() { return nil } if ebiten.IsKeyPressed(ebiten.KeyEscape) { return fmt.Errorf("game closed") } screen.Fill(backgroundColor) op := &ebiten.DrawImageOptions{} op.GeoM.Translate(100, 100) screen.DrawImage(gopher, op) return nil } func main() { ebiten.SetWindowSize(screenWidth, screenHeight) ebiten.SetWindowTitle("My Ebiten Game") gopher, _, err := ebitenutil.NewImageFromFile("gopher.png") if err != nil { log.Fatal(err) } if err := ebiten.RunGame(&Game{}); err != nil { log.Fatal(err) } }
This simple example creates a window with a Gopher image displayed at coordinates (100, 100). Make sure to replace “gopher.png” with the path to your own image file.
2.5. Running Your Game
To run your game, execute the following command in your terminal:
bash go run main.go
You should see a window displaying your Gopher image. Congratulations, you’ve just created your first Ebiten game!
3. Building Game Mechanics
Now that you have a basic game window set up, let’s explore how to add game mechanics and interactivity to your game.
3.1. Handling Input
Ebiten makes it easy to handle user input. You can detect key presses, mouse clicks, and other events with minimal effort. Here’s an example of how to handle keyboard input to move a character:
go func update(screen *ebiten.Image) error { // ... if ebiten.IsKeyPressed(ebiten.KeyArrowLeft) { // Move character left } if ebiten.IsKeyPressed(ebiten.KeyArrowRight) { // Move character right } // ... return nil }
3.2. Game Loop
Ebiten uses a game loop to continuously update and render your game. You’ve already seen the update function, which is called at a consistent frame rate. You can use this function to update game logic, move objects, and check for collisions.
go func update(screen *ebiten.Image) error { // Update game logic here return nil }
3.3. Collision Detection
Collision detection is a crucial aspect of game development. Ebiten provides tools for checking collisions between objects. You can use bounding boxes or custom collision detection algorithms, depending on your game’s complexity.
go func isCollision(obj1, obj2 *ebiten.Image) bool { // Implement your collision detection logic here }
4. Rendering Graphics
Creating visually appealing games requires rendering graphics efficiently. Ebiten simplifies this process with its built-in rendering capabilities.
4.1. Drawing Images
You’ve already seen how to draw an image on the screen. Ebiten offers various options for rotating, scaling, and transforming images, allowing you to create dynamic animations and effects.
go op := &ebiten.DrawImageOptions{} op.GeoM.Translate(x, y) op.GeoM.Rotate(angle) op.GeoM.Scale(scaleX, scaleY) screen.DrawImage(image, op)
4.2. Sprites and Animation
To create sprite-based animations, you can split your sprite sheet into individual frames and cycle through them in your game loop.
go // Load sprite sheet spriteSheet, _, err := ebitenutil.NewImageFromFile("spritesheet.png") if err != nil { log.Fatal(err) } // Define sprite dimensions and positions spriteWidth := 32 spriteHeight := 32 spriteX := 0 spriteY := 0 // Create an animation by changing sprite position func update(screen *ebiten.Image) error { // ... spriteX += spriteWidth if spriteX >= spriteSheet.Bounds().Max.X { spriteX = 0 } op := &ebiten.DrawImageOptions{} op.GeoM.Translate(x, y) srcRect := image.Rect(spriteX, spriteY, spriteX+spriteWidth, spriteY+spriteHeight) screen.DrawImage(spriteSheet.SubImage(srcRect).(*ebiten.Image), op) // ... return nil }
4.3. Text and UI Elements
Ebiten supports rendering text and UI elements using the ebiten.Text type. You can display scores, health bars, and other in-game information using text.
go text := &ebiten.Text{ Text: "Score: 100", Color: color.White, } func update(screen *ebiten.Image) error { // ... text.Draw(screen, "Score: 100", fontFace, 20, 20) // ... return nil }
5. Advanced Features
As you become more comfortable with Ebiten, you can explore its advanced features to create richer and more complex games.
5.1. Audio
Ebiten supports audio playback, allowing you to add sound effects and background music to your games. You can load and play audio files with ease.
go audioContext, err := audio.NewContext(44100) if err != nil { log.Fatal(err) } sound, err := ebitenutil.NewSoundFromFile("sound.wav") if err != nil { log.Fatal(err) } func update(screen *ebiten.Image) error { // ... if ebiten.IsKeyPressed(ebiten.KeySpace) { audioContext.Play(sound) } // ... return nil }
5.2. Game States and Screens
For more complex games, you can implement different game states or screens, such as menus, levels, and game over screens. Each screen can have its own logic and rendering.
go type GameState int const ( Menu GameState = iota Playing GameOver ) func update(screen *ebiten.Image) error { switch gameState { case Menu: // Handle menu logic and rendering case Playing: // Handle gameplay logic and rendering case GameOver: // Handle game over logic and rendering } return nil }
5.3. Physics and Collision Libraries
For physics-based games or advanced collision detection, you can integrate external libraries with Ebiten. Popular physics engines like Matter.js or Chipmunk can be used in conjunction with Ebiten to enhance your game’s realism.
Conclusion
Ebiten is a powerful and versatile game development library for the Go programming language. With its simplicity, cross-platform compatibility, and performance optimization, it’s an excellent choice for both beginners and experienced developers. Start your game development journey with Ebiten today, and bring your game ideas to life!
In this blog, we’ve covered the basics of setting up your development environment, handling input, implementing game mechanics, and rendering graphics. As you delve deeper into Ebiten’s capabilities, you’ll be able to create more complex and captivating games. Don’t hesitate to explore the official Ebiten documentation and join the community to unlock the full potential of this remarkable game library. Happy coding and game development!
Table of Contents
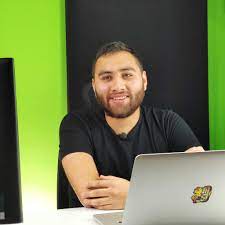
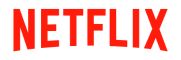