Behind the Scenes of Go: A Deep Dive into Garbage Collection and Efficient Memory Management
Understanding how memory management works in Go, particularly garbage collection, is crucial for writing efficient and optimized Go programs. This knowledge is a key factor when looking to hire Golang developers, as it demonstrates their capacity to write performant, resource-friendly applications. In this blog post, we’ll delve into the specifics of Go’s garbage collection and provide examples to better illustrate how it works. With these insights, you’ll be better equipped to evaluate the expertise of potential Golang developers you aim to hire.
Introduction to Memory Management in Go
In a computing environment, memory management is the task of managing the primary memory – a process that involves tracking each byte in the computer’s memory and deciding which processes are allocated to different memory blocks. This is important because efficient memory management enhances the performance of applications by ensuring that memory is properly allocated and deallocated.
In many traditional languages like C and C++, developers are responsible for memory management. But in modern languages such as Go (Golang), memory management is automatic, and the garbage collector plays a key role in this.
What is Garbage Collection?
Garbage collection (GC) is a form of automatic memory management. It’s a process through which programs try to reclaim the memory occupied by objects that are no longer in use by the application.
In Go, garbage collection happens concurrently with the program execution and it doesn’t require the programmer to explicitly allocate and deallocate memory blocks. This is one of the main reasons Go is admired for its simplicity and efficiency.
How Garbage Collection Works in Go
Go uses a tricolor mark-and-sweep garbage collector algorithm. This is a concurrent, parallel, and generational algorithm, which provides low pause times and does not compromise on performance.
- Mark Phase: During the marking phase, Go GC traces through each object in the memory. It colors all objects – white, gray or black. All objects are initially white. When an object is reached, it’s turned gray. When all reachable objects from a gray object are marked, it’s then turned black. Unreachable objects (still white after the marking phase) are considered as “garbage”.
- Sweep Phase: During the sweep phase, the memory occupied by the white objects (garbage) is reclaimed.
Let’s illustrate this with an example:
```go package main import ( "fmt" "runtime/debug" ) func main() { debug.SetGCPercent(-1) // disable automatic GC a := new(int) *a = 1 b := new(int) *b = 2 c := new(int) *c = 3 a = nil b = nil // At this point, the memory referenced by a and b is unreachable, // and they are considered as "garbage". fmt.Println("Before GC: ", *c) runtime.GC() // call GC manually fmt.Println("After GC: ", *c) } ```
In the example above, we first disable automatic garbage collection with `debug.SetGCPercent(-1)`. Then we create three pointers a, b, and c, referencing different memory locations. After setting a and b to nil, we manually call the GC with `runtime.GC()`. After the garbage collection, the memory referenced by a and b is freed, and only the memory referenced by c is kept.
Go’s Efficient Memory Management
The tricolor mark-and-sweep garbage collector algorithm, as used in Go, provides several benefits that result in efficient memory management.
- Parallelism: Go’s GC uses as many threads as there are available CPU cores for the mark phase. This means it can mark multiple objects concurrently, resulting in reduced GC latency.
- Generational Collection: Go’s GC performs generational garbage collection, meaning that it optimizes the process by primarily focusing on newer objects as they are more likely to be garbage (according to the generational hypothesis).
- Minimal Stop the World (STW) Time: “Stop the World” is a period during which the program’s execution is paused so that the GC can do its work. Go’s GC aims to minimize STW time, which in turn improves the application’s performance.
Here is an example demonstrating how Go handles a large number of objects efficiently.
```go package main import ( "fmt" "runtime" "time" ) func main() { start := time.Now() for i := 0; i < 100000000; i++ { s := &struct{}{} // create a new object _ = s } duration := time.Since(start) fmt.Println("Execution time: ", duration) var m runtime.MemStats runtime.ReadMemStats(&m) fmt.Printf("Memory Acquired: %v MiB\n", m.Sys/1024/1024) fmt.Printf("Memory Used: %v MiB\n", m.Alloc/1024/1024) fmt.Printf("GC Times: %v\n", m.NumGC) } ```
The program creates a large number of objects in a loop. Despite the significant memory demand, Go manages this efficiently, minimizing memory usage and the number of GC invocations. This efficient memory management is one of the key aspects to look for when you hire Golang developers, as it shows their ability to write scalable and optimized code, even when dealing with a large amount of data.
Conclusion
Go’s garbage collector is a remarkable tool for developers. It simplifies memory management by automatically allocating and deallocating memory blocks, allowing developers to focus more on the logic of their applications.
While GC in Go might appear magical, understanding how it works can empower developers to write more efficient and optimized Go programs. This level of understanding is a crucial attribute when you plan to hire Golang developers, as it enables them to make informed decisions about memory usage, and where necessary, adjust their coding practices to better fit the garbage collector’s operations.
It’s also important to note that while GC offers many advantages, it’s not a silver bullet for all memory problems. Good programming practices and a sound understanding of how memory works in Go are essential for building effective and optimized Go applications. Hence, when you hire Golang developers, ensure they possess these critical skills for managing memory-related challenges effectively.
Table of Contents
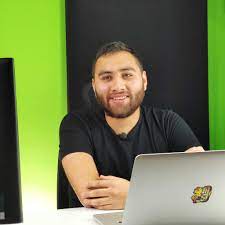
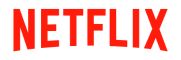