Using Go for Geospatial Data: Working with Maps and GIS
In today’s data-driven world, geospatial information is integral to numerous applications, from location-based services to urban planning and environmental monitoring. If you’re a developer or data enthusiast looking to dive into the world of geospatial data, you’ve come to the right place. In this comprehensive guide, we’ll explore how to use the Go programming language to work with geospatial data, create maps, and perform Geographic Information System (GIS) analysis.
1. Why Go for Geospatial Data?
Before we delve into the practical aspects, let’s briefly discuss why Go is a fantastic choice for geospatial data tasks.
1.1. Speed and Efficiency
Go is known for its blazing-fast performance and efficiency. When dealing with large geospatial datasets, speed is crucial. Whether you’re parsing shapefiles, conducting spatial analysis, or rendering maps, Go’s performance can significantly reduce processing time.
1.2. Strong Ecosystem
The Go ecosystem boasts libraries and packages that make geospatial data manipulation and analysis relatively straightforward. Libraries like “gonum” for numerical computations and “go-geojson” for working with GeoJSON files simplify complex tasks.
1.3. Cross-Platform Compatibility
Go is a cross-platform language, which means your geospatial applications can run on various operating systems without major modifications. This versatility is invaluable when developing GIS tools for different environments.
Now that we understand why Go is a great choice let’s dive into the practical aspects of working with geospatial data in Go.
2. Setting Up Your Environment
Before we start coding, you’ll need to set up your development environment. We’ll assume you already have Go installed on your system. If not, visit the official Go website (golang.org) and follow the installation instructions for your platform.
2.1. Installing Dependencies
To work with geospatial data in Go, you’ll need to install a few essential libraries and tools. The most commonly used packages for geospatial tasks are:
- github.com/paulmach/orb: This library provides geometric primitives and operations, making it easier to work with points, lines, and polygons.
- github.com/paulmach/orb/geojson: If you’re dealing with GeoJSON data, this package allows you to parse, create, and manipulate GeoJSON documents effortlessly.
- github.com/fogleman/gg: For map rendering and visualization, the “gg” package is an excellent choice. It provides a simple and flexible API for creating maps and graphics.
You can install these packages using the go get command:
go go get -u github.com/paulmach/orb go get -u github.com/paulmach/orb/geojson go get -u github.com/fogleman/gg
With your environment set up and dependencies installed, we can move on to working with geospatial data.
3. Loading and Visualizing Geospatial Data
The first step in any geospatial project is to load and visualize your data. For this example, we’ll use a GeoJSON file containing geographic features. Let’s load this data and create a simple map using the “orb” and “gg” libraries.
3.1. Loading GeoJSON Data
Assuming you have a GeoJSON file named data.geojson, you can read it into your Go program as follows:
go package main import ( "fmt" "os" "github.com/paulmach/orb/geojson" ) func main() { // Open the GeoJSON file file, err := os.Open("data.geojson") if err != nil { fmt.Println("Error opening GeoJSON file:", err) return } defer file.Close() // Parse the GeoJSON data fc, err := geojson.DecodeFeatureCollection(file) if err != nil { fmt.Println("Error decoding GeoJSON:", err) return } // Now you can work with the GeoJSON feature collection fmt.Println("Number of features:", len(fc.Features)) }
This code snippet opens the GeoJSON file, decodes it into a feature collection, and prints the number of features in the collection.
3.2. Visualizing the Data
To visualize the data, we’ll create a simple map using the “gg” library and add the features from our GeoJSON file.
go package main import ( "fmt" "os" "github.com/paulmach/orb/geojson" "github.com/fogleman/gg" ) func main() { // Open the GeoJSON file file, err := os.Open("data.geojson") if err != nil { fmt.Println("Error opening GeoJSON file:", err) return } defer file.Close() // Parse the GeoJSON data fc, err := geojson.DecodeFeatureCollection(file) if err != nil { fmt.Println("Error decoding GeoJSON:", err) return } // Create a new image context const width, height = 800, 600 dc := gg.NewContext(width, height) // Set a background color dc.SetRGB(1, 1, 1) dc.Clear() // Define the map's bounding box bbox := fc.Bound() // Loop through features and draw them on the map for _, feature := range fc.Features { // Your drawing code here } // Save or display the map dc.SavePNG("map.png") }
This code sets up a canvas using the “gg” library, defines a bounding box for your map, and iterates through the GeoJSON features to draw them on the map. You can customize the drawing code based on your specific dataset and requirements.
4. Performing Spatial Analysis
Now that we’ve loaded and visualized our geospatial data, let’s explore some basic spatial analysis tasks you can perform with Go.
4.1. Point-in-Polygon Test
One common spatial analysis task is determining whether a point is inside a polygon. You might use this to check if a GPS coordinate falls within a specific region. Here’s how you can do it using the “orb” library:
go package main import ( "fmt" "github.com/paulmach/orb" "github.com/paulmach/orb/geojson" ) func main() { // Load a GeoJSON polygon polygonJSON := `{ "type": "Polygon", "coordinates": [[[0, 0], [0, 10], [10, 10], [10, 0], [0, 0]]] }` polygon, _ := geojson.UnmarshalPolygon([]byte(polygonJSON)) // Define a point point := orb.Point{5, 5} // Check if the point is inside the polygon inside := orb.InRing(point, polygon[0]) if inside { fmt.Println("Point is inside the polygon.") } else { fmt.Println("Point is outside the polygon.") } }
In this example, we define a polygon and a point, then use the orb.InRing() function to determine if the point is inside the polygon.
4.2. Distance Calculation
Calculating the distance between two points on the Earth’s surface is a fundamental spatial analysis task. Go provides libraries to make this calculation straightforward. Here’s an example using the “orb” library:
go package main import ( "fmt" "github.com/paulmach/orb" "github.com/paulmach/orb/planar" ) func main() { // Define two points (latitude, longitude) point1 := orb.Point{40.7128, -74.0060} // New York City point2 := orb.Point{34.0522, -118.2437} // Los Angeles // Calculate the distance between the points in meters distance := planar.Distance(point1, point2) fmt.Printf("Distance between New York City and Los Angeles: %.2f km\n", distance/1000) }
This code snippet calculates the distance between New York City and Los Angeles using the Haversine formula.
5. Building a Geospatial Application
Now that we’ve covered the basics, let’s work on a practical example of building a geospatial application. We’ll create a simple CLI tool that calculates the distance between two GPS coordinates.
5.1. Creating a Distance Calculator
go package main import ( "fmt" "os" "strconv" "github.com/paulmach/orb" "github.com/paulmach/orb/planar" ) func main() { if len(os.Args) != 5 { fmt.Println("Usage: distance-calculator lat1 lon1 lat2 lon2") os.Exit(1) } lat1, _ := strconv.ParseFloat(os.Args[1], 64) lon1, _ := strconv.ParseFloat(os.Args[2], 64) lat2, _ := strconv.ParseFloat(os.Args[3], 64) lon2, _ := strconv.ParseFloat(os.Args[4], 64) point1 := orb.Point{lat1, lon1} point2 := orb.Point{lat2, lon2} distance := planar.Distance(point1, point2) fmt.Printf("Distance between (%.4f, %.4f) and (%.4f, %.4f): %.2f km\n", lat1, lon1, lat2, lon2, distance/1000) }
You can compile and run this program by providing four arguments: the latitude and longitude of two points. It will then calculate and display the distance between those points.
Conclusion
In this guide, we’ve explored the world of geospatial data using the Go programming language. We’ve covered setting up your environment, loading and visualizing geospatial data, performing spatial analysis, and even building a simple geospatial application. Armed with these tools and knowledge, you can now embark on your own geospatial projects, from mapping applications to advanced GIS analysis.
Go’s speed, efficiency, and robust libraries make it an excellent choice for geospatial tasks. So, whether you’re tracking your fitness route, developing a location-based app, or tackling complex geographic problems, Go has you covered in the world of geospatial data. Happy coding!
Table of Contents
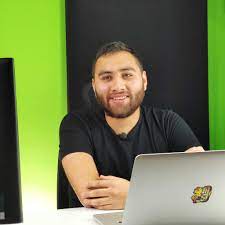
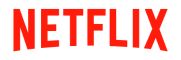