Building Scalable Web Applications: A Beginner’s Guide to RESTful APIs with Go
Building a RESTful API (Representational State Transfer Application Programming Interface) can be a daunting task, but with Go, it’s simplified, efficient, and enjoyable. Go is a statically typed, compiled language developed by Google that prioritizes simplicity, efficiency, and reliability. This makes it a top choice for businesses looking to hire Golang developers. It has become increasingly popular among developers due to its high performance and ease of use in building scalable web applications. This blog post will introduce you to web development with Go, focusing on building RESTful APIs, with examples to guide you along the way. If you’re planning to hire Golang developers, understanding these concepts can aid in communicating your requirements effectively.
Understanding RESTful APIs
In simple terms, a RESTful API is an application interface that uses HTTP methods to get, create, update, or delete data. The idea is to use standard HTTP methods in a consistent manner to manipulate resources represented in various formats like JSON, XML, or HTML.
Setting Up Go Environment
Before we get started, you will need to install Go on your local machine. Detailed instructions for doing so on various operating systems can be found on the [official Go website](https://golang.org/doc/install). Once the installation is complete, you can verify it by running the following command in your terminal:
``` $ go version ```
Let’s Start Coding!
Now, let’s create a basic Go application to illustrate how a RESTful API works. We’ll build an API for a simple blogging platform where users can read, add, and delete blog posts.
Step 1: Structuring Our Application
Create a new directory for your application and navigate into it using your terminal. Inside this directory, create a main.go file.
First, let’s define the structure of a blog post:
```go package main type Post struct { ID string `json:"id"` Title string `json:"title"` Content string `json:"content"` Author string `json:"author"` } ```
Here, `Post` is a struct that defines the properties of a blog post. The `json` tags are used to define the JSON key that maps to each struct field.
Step 2: Mock Data
In a real application, you would retrieve data from a database, but for the sake of simplicity, we’ll use some mock data:
```go var posts []Post = []Post{ { ID: "1", Title: "Intro to Go", Content: "Go is a statically typed, compiled language developed by Google...", Author: "John Doe", }, { ID: "2", Title: "Advanced Go", Content: "Go offers a range of advanced features...", Author: "Jane Doe", }, } ```
Step 3: Handling HTTP Requests
Go’s `net/http` package provides functionalities for building HTTP servers and clients. Let’s start by creating a simple server:
```go package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, you've requested: %s\n", r.URL.Path) }) http.ListenAndServe(":8080", nil) } ```
When you navigate to http://localhost:8080 on your web browser, you should see “Hello, you’ve requested: /” displayed.
Step 4: Defining Our API Endpoints
Now, we’ll define our API endpoints to interact with our blog posts.
First, let’s create a handler function to get all posts:
```go package main import ( "encoding/json" "net/http" ) func getPosts(w http.ResponseWriter, r *http.Request) { w .Header().Set("Content-Type", "application/json") json.NewEncoder(w).Encode(posts) } func main() { http.HandleFunc("/posts", getPosts) http.ListenAndServe(":8080", nil) } ```
In the getPosts function, we set the HTTP response’s content type to “application/json” and encode our posts into JSON.
Step 5: Handling CRUD Operations
Next, we’ll handle CRUD (Create, Read, Update, Delete) operations.
Get a Single Post
```go func getPost(w http.ResponseWriter, r *http.Request) { id := r.URL.Query().Get("id") for _, post := range posts { if post.ID == id { json.NewEncoder(w).Encode(post) return } } json.NewEncoder(w).Encode(&Post{}) } ```
In the getPost function, we fetch the “id” parameter from the URL query, and iterate over our posts slice to find a post with the matching id.
Create a New Post
To create new posts, we’ll need to parse JSON from the HTTP request body. Go makes this simple with the `json.NewDecoder` function:
```go func createPost(w http.ResponseWriter, r *http.Request) { var newPost Post json.NewDecoder(r.Body).Decode(&newPost) posts = append(posts, newPost) json.NewEncoder(w).Encode(newPost) } ```
Step 6: Bringing it All Together
Finally, let’s use the `gorilla/mux` package to create more flexible routes:
```go package main import ( "encoding/json" "net/http" "github.com/gorilla/mux" ) func main() { router := mux.NewRouter() router.HandleFunc("/posts", getPosts).Methods("GET") router.HandleFunc("/posts/{id}", getPost).Methods("GET") router.HandleFunc("/posts", createPost).Methods("POST") http.ListenAndServe(":8080", router) } ```
This completes a simple demonstration of building a RESTful API in Go, showcasing the expertise you would expect when you hire Golang developers. Of course, a real-world application would involve more complexities like handling CORS, validating input, authenticating requests, and connecting to a database, among other things. By comprehending these fundamentals, you can better articulate your project needs when you decide to hire Golang developers. But this should give you a good starting point to explore web development with Go.
Conclusion
Go offers powerful features for building high-performance web applications, a compelling reason to hire Golang developers for your next project. With its simplicity, strong standard library, and efficient performance, Go is an excellent choice for building RESTful APIs. Understanding these capabilities will ensure you make the most out of your decision to hire Golang developers. Happy coding!
Table of Contents
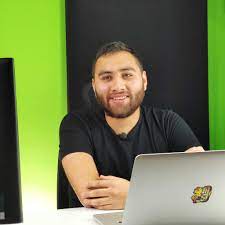
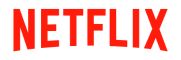