Revolutionize Your Web Content: Mastering Go’s HTML Template Package
Table of Contents
1. Introduction to Go’s HTML Template Package: Dynamic Web Content
If you’re a tech-savvy early-stage startup founder, VC investor, or tech leader, you know the importance of having a dynamic web presence. In this fast-paced digital era, websites need to be more than just static pages; they need to adapt and change according to user input or data. This is where Go’s HTML Template Package comes into play, allowing you to create dynamic web content efficiently.
2. What is Go’s HTML Template Package?
Go, also known as Golang, is a popular programming language known for its simplicity, efficiency, and strong support for concurrency. The HTML Template Package is a part of the standard Go library and provides a powerful way to generate dynamic HTML content. It is particularly useful when you need to render HTML templates with data from your application.
3. Getting Started
To start using Go’s HTML Template Package, you first need to import it into your Go program:
```go import ( "html/template" "os" ) ```
Next, you’ll need to parse your HTML template file using the `template.ParseFiles` function:
```go t, err := template.ParseFiles("your_template.html") if err != nil { panic(err) } ```
4. Creating Dynamic Content
One of the key features of the HTML Template Package is its ability to insert dynamic data into your HTML templates. Let’s say you want to display the name of a user on your webpage. You can achieve this by defining a struct and passing an instance of that struct to your template:
```go type User struct { Name string } user := User{Name: "John Doe"} err = t.Execute(os.Stdout, user) if err != nil { panic(err) } ```
In your HTML template, you can use the `{{.Name}}` placeholder to display the user’s name:
```html <!DOCTYPE html> <html> <head> <title>Hello, {{.Name}}!</title> </head> <body> <h1>Hello, {{.Name}}!</h1> </body> </html> ```
When you execute this template, it will dynamically generate HTML with the user’s name, making your webpage personalized and engaging.
5. Examples of Dynamic Content
- Iterating Over a Slice: You can use the `range` action to iterate over a slice of data and display it dynamically in your HTML template. This is incredibly useful when you want to show lists of items, such as blog posts or products.
```html <ul> {{range .Posts}} <li>{{.Title}}</li> {{end}} </ul> ```
- Conditional Rendering: With Go’s HTML Template Package, you can include conditional logic in your templates to display content based on certain conditions. For example, you can show different messages to logged-in and logged-out users.
```html {{if .IsLoggedIn}} <p>Welcome back, {{.Username}}!</p> {{else}} <p>Please log in to access this content.</p> {{end}} ```
- External Data Sources: Go’s HTML Template Package also allows you to fetch and display data from external sources, such as APIs. You can make HTTP requests, retrieve data, and seamlessly integrate it into your templates.
6. External Resources
To dive deeper into Go’s HTML Template Package and its capabilities, here are three external resources that you might find helpful:
- Official Go Documentation on HTML Templates: Explore the official documentation to gain a comprehensive understanding of the package and its features.
- A Tour of Go – HTML Template: Take a guided tour of Go’s HTML Template Package with interactive examples.
- Building Web Applications with Go: This blog post provides a practical guide on using Go for web development, including a section on HTML templates.
Conclusion
Go’s HTML Template Package is a valuable tool for creating dynamic web content, and it aligns perfectly with the needs of early-stage startup founders, VC investors, and tech leaders. With the ability to generate personalized and data-driven webpages, you can enhance your online presence and engage your audience effectively.
So, roll up your sleeves, explore the resources, and start building dynamic web applications with Go today!
Table of Contents
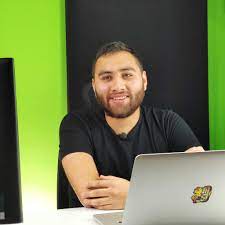
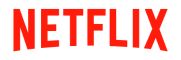