Go for Image Processing: Manipulating and Analyzing Images
Images are an integral part of our digital world, from the photos we share on social media to the medical images used for diagnosis. Image processing, the art of manipulating and analyzing images, plays a crucial role in various fields, including computer vision, medical imaging, and graphic design. In this blog, we will delve into the world of image processing using the Go programming language, demonstrating how Go’s powerful libraries and techniques can be harnessed for image manipulation and analysis.
1. Why Choose Go for Image Processing?
Go, also known as Golang, is a statically typed, compiled language that has gained popularity in recent years due to its simplicity, efficiency, and robust standard library. It may not be the first language that comes to mind for image processing, but it offers several compelling reasons to consider:
1.1. Performance
Go is known for its exceptional performance. It compiles to machine code, which means it can efficiently handle CPU-intensive tasks, making it ideal for image processing tasks that require speed and responsiveness.
1.2. Concurrency
Go’s concurrency primitives, such as goroutines and channels, make it well-suited for parallel processing tasks. When dealing with large batches of images or complex algorithms, Go’s concurrency capabilities can significantly speed up the processing.
1.3. Strong Standard Library
Go’s standard library includes packages for handling images, making it easy to work with various image formats, perform basic manipulations, and analyze image data.
1.4. Cross-Platform Compatibility
Go is a cross-platform language, meaning your image processing code can run on different operating systems without major modifications.
2. Setting Up Your Development Environment
Before we dive into image processing with Go, you’ll need to set up your development environment. Ensure you have Go installed, and consider using a Go-specific IDE or text editor, such as Visual Studio Code with the Go extension, to enhance your productivity.
2.1. Image Loading and Display
Let’s start with a fundamental image processing task: loading and displaying an image. Go provides a convenient package called “image” that allows us to work with images in various formats.
go package main import ( "fmt" "image" "image/jpeg" "os" ) func main() { // Open the image file file, err := os.Open("sample.jpg") if err != nil { fmt.Println("Error opening image:", err) return } defer file.Close() // Decode the image img, _, err := image.Decode(file) if err != nil { fmt.Println("Error decoding image:", err) return } // Display image details bounds := img.Bounds() fmt.Println("Image format:", bounds.Dx(), "x", bounds.Dy()) }
In this example, we open an image file (“sample.jpg”), decode it, and then print the image’s dimensions. This is a simple way to load and inspect an image in Go.
2.2. Image Manipulation
Go’s “image” package provides the basic building blocks for image manipulation. You can perform operations like resizing, cropping, and rotating images. Let’s resize an image as an example:
go package main import ( "fmt" "image" "image/jpeg" "os" ) func main() { // Open the input image file inputFile, err := os.Open("input.jpg") if err != nil { fmt.Println("Error opening input image:", err) return } defer inputFile.Close() // Decode the input image inputImg, _, err := image.Decode(inputFile) if err != nil { fmt.Println("Error decoding input image:", err) return } // Resize the image width := 300 height := 200 outputImg := image.NewRGBA(image.Rect(0, 0, width, height)) g := resize.New(outputImg, outputImg.Bounds(), inputImg, inputImg.Bounds(), resize.Lanczos3) g.Draw(outputImg, outputImg.Bounds(), inputImg, inputImg.Bounds()) // Save the resized image outputFile, err := os.Create("output.jpg") if err != nil { fmt.Println("Error creating output image:", err) return } defer outputFile.Close() jpeg.Encode(outputFile, outputImg, nil) fmt.Println("Image resized and saved as output.jpg") }
In this example, we open an input image, decode it, and then create a new image with the desired dimensions. We use the resize package to perform the resizing operation and save the resulting image as “output.jpg.”
2.3. Image Analysis
Image analysis involves extracting useful information from images, such as identifying objects, detecting patterns, or measuring properties. Go offers libraries and tools to perform various image analysis tasks. Let’s explore a simple image analysis example: edge detection using the “gocv” package.
2.3.1. Edge Detection with gocv
gocv is a popular Go package for computer vision and image processing. It provides bindings to the OpenCV library, which is a powerful tool for image analysis. To get started, you’ll need to install gocv and set up OpenCV on your system.
Here’s a basic example of edge detection using gocv:
go package main import ( "fmt" "gocv.io/x/gocv" ) func main() { // Open the image img := gocv.IMRead("input.jpg", gocv.IMReadGray) if img.Empty() { fmt.Println("Error opening image") return } defer img.Close() // Apply Canny edge detection edges := gocv.NewMat() gocv.Canny(img, &edges, 100, 200) // Save the resulting edges image gocv.IMWrite("edges.jpg", edges) fmt.Println("Edges detected and saved as edges.jpg") }
In this code, we open an input image in grayscale mode and then apply the Canny edge detection algorithm. The resulting edges are saved as “edges.jpg.”
Conclusion
Go is a versatile programming language that can be a powerful tool for image processing. Its performance, concurrency support, and strong standard library make it well-suited for a wide range of image manipulation and analysis tasks. Whether you’re working on computer vision projects, enhancing your photos, or analyzing medical images, Go provides the tools and capabilities you need to get the job done efficiently and effectively.
In this blog, we’ve only scratched the surface of what you can achieve with Go in image processing. As you explore further, you’ll discover a wealth of libraries and resources that can help you tackle more advanced image analysis tasks, from object detection to facial recognition. So, dive in, experiment, and unlock the potential of Go in the fascinating world of image processing.
Image processing is a captivating field that continues to evolve with technological advancements. By leveraging the power of Go, you can be at the forefront of this exciting domain, creating innovative solutions and pushing the boundaries of what’s possible in image manipulation and analysis. Happy coding!
Table of Contents
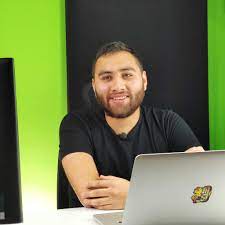
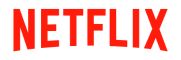