Understanding Go’s Interface and Polymorphism: Writing Flexible Code
Go, also known as Golang, is a statically typed, compiled programming language designed to be efficient, expressive, and concurrent. One of the language’s key features that contributes to its simplicity and flexibility is its implementation of interfaces and polymorphism. In this blog, we will explore Go’s interfaces, understand the concept of polymorphism, and see how they enable us to write more flexible and extensible code.
1. What are Interfaces?
In Go, an interface is a collection of method signatures. It defines a contract, specifying what methods a type must have to be considered as implementing the interface. Any type that satisfies all the method signatures of an interface implicitly implements that interface.
Interfaces in Go are defined using the interface keyword. Let’s see a simple example of an interface:
go package main import "fmt" type Shape interface { Area() float64 Perimeter() float64 }
In this example, we’ve defined a Shape interface with two method signatures: Area() and Perimeter(), both returning float64 values. Any type that implements these methods will automatically satisfy the Shape interface.
2. Declaring Interfaces
2.1. Empty Interfaces
In Go, an empty interface interface{} represents a type that has zero methods. This means any value can be assigned to an empty interface, making it a powerful construct for handling unknown types or creating generic functions.
go func printValue(val interface{}) { fmt.Println("Value:", val) }
In the above example, the function printValue can take any argument of any type due to the empty interface.
2.2. Interface Embedding
Go allows interfaces to be embedded within one another, creating a new interface that combines the method sets of its components. This feature allows us to compose interfaces and build more specific contracts on top of existing ones.
go type ReadWrite interface { Read() error Write() error } type ReadWriteClose interface { ReadWrite Close() error }
Here, ReadWriteClose is an interface that embeds the ReadWrite interface, adding an extra method Close(). Any type that satisfies ReadWriteClose must also implement the methods from the embedded ReadWrite interface.
3. Implementing Interfaces
In Go, a type automatically satisfies an interface if it implements all the methods declared in the interface. There is no explicit declaration of intent to implement an interface, making the implementation implicit.
Let’s implement the Shape interface on two types: Rectangle and Circle.
go type Rectangle struct { Width, Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func (r Rectangle) Perimeter() float64 { return 2 * (r.Width + r.Height) } type Circle struct { Radius float64 } func (c Circle) Area() float64 { return math.Pi * c.Radius * c.Radius } func (c Circle) Perimeter() float64 { return 2 * math.Pi * c.Radius }
Both Rectangle and Circle now implicitly implement the Shape interface because they provide implementations for all the required methods.
4. Polymorphism in Go
Polymorphism allows objects of different types to be treated as objects of a common superclass, providing a unified interface for multiple concrete implementations. Go achieves polymorphism using interfaces.
4.1. Dynamic Polymorphism
Dynamic polymorphism allows us to call different methods on objects at runtime, depending on their actual type. Since Go is statically typed, it doesn’t support dynamic polymorphism in the traditional sense, but it has an analogous concept using interfaces and type assertions.
go func PrintShapeInfo(s Shape) { fmt.Println("Area:", s.Area()) fmt.Println("Perimeter:", s.Perimeter()) }
In this example, the PrintShapeInfo function can accept any type that implements the Shape interface. It doesn’t need to know the concrete type at compile time.
4.2. Static Polymorphism
Static polymorphism, also known as compile-time polymorphism, is a concept where the appropriate method to call is determined at compile time based on the type information. Since Go is statically typed, it naturally supports static polymorphism through interfaces.
Consider the following example:
go type Printer interface { Print() } type StringPrinter struct { Text string } func (sp StringPrinter) Print() { fmt.Println(sp.Text) } type IntPrinter struct { Number int } func (ip IntPrinter) Print() { fmt.Println(ip.Number) }
Here, we have defined two types, StringPrinter and IntPrinter, both implementing the Printer interface. By defining a common method signature Print() in the interface, we achieve static polymorphism, enabling us to call the Print() method on both types without knowing their specific implementations.
go func PrintInfo(p Printer) { p.Print() }
The PrintInfo function can now accept any type that implements the Printer interface. At compile time, the correct Print() method for the given type will be called.
5. Benefits of Using Interfaces
Using interfaces in Go offers several benefits that enhance code quality, reusability, and maintainability.
5.1. Code Reusability
By defining behavior through interfaces, we can write functions that work with multiple types, promoting code reuse. For instance, we can create a generic CalculateArea function that operates on any type implementing the Shape interface, without needing separate functions for each shape.
go func CalculateArea(s Shape) float64 { return s.Area() }
5.2. Easy Extensibility
Interfaces allow us to extend existing code without modifying it. If we have a function that works with a specific interface, we can create new types that implement the same interface and seamlessly use them with the existing function.
This concept is particularly useful when dealing with third-party libraries. If a library defines an interface, you can create your own implementation and pass it to the library without altering its core functionality.
5.3. Mocking and Testing
Interfaces play a crucial role in writing unit tests. By defining interfaces for dependencies, we can create mock implementations to simulate behavior during testing. This isolates the code under test from its dependencies, making it easier to test each component in isolation.
6. Type Assertions and Type Switches
Since Go’s interfaces allow any concrete type to be used, it may sometimes be necessary to retrieve the underlying type to access its specific methods or fields. This is where type assertions and type switches come into play.
6.1. Type Assertions
A type assertion allows us to extract the concrete value from an interface. It has two forms: one returning a value and the other returning a value and a boolean indicating whether the assertion was successful.
go func PrintValue(val interface{}) { str, ok := val.(string) if ok { fmt.Println("Value is a string:", str) } else { fmt.Println("Value is not a string") } }
6.2. Type Switches
Type switches offer a concise way to check the underlying type of an interface against multiple possibilities. It helps to handle different cases based on the type.
go func ProcessValue(val interface{}) { switch v := val.(type) { case int: fmt.Println("Value is an integer:", v) case string: fmt.Println("Value is a string:", v) default: fmt.Println("Unknown type") } }
7. Interface Best Practices
To make the most of interfaces in your Go code, consider the following best practices:
- Keep Interfaces Small: Define interfaces with a few methods. Large interfaces can lead to unnecessary coupling and make it challenging to implement.
- Name Interfaces Descriptively: Choose descriptive and precise names for interfaces to clearly communicate their purpose and role.
- Provide Meaningful Method Names: Use method names that convey their purpose and behavior, making the code more self-explanatory.
- Favor Multiple Interfaces Over Large Ones: Rather than creating a single large interface, prefer composing smaller interfaces to achieve the required functionality.
- Document Interfaces: Write clear documentation for your interfaces, detailing their purpose and expected behavior. Well-documented interfaces are essential for their effective use.
Conclusion
Go’s interface and polymorphism capabilities offer a unique and powerful way to write flexible and extensible code. By defining behavior through interfaces, you can achieve code reusability, easy extensibility, and improve testability. Understanding how interfaces work, along with type assertions and switches, empowers you to write more dynamic and flexible programs in Go.
So, start embracing the power of Go’s interfaces and polymorphism to build robust and adaptable software systems!
Remember, practice is key to mastering this concept. Play around with interfaces, implement them in your projects, and observe the benefits they bring to your codebase. Happy coding!
Table of Contents
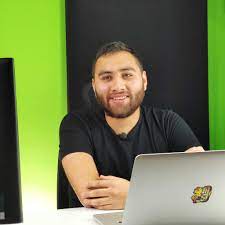
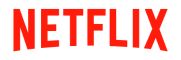