Go for IoT: Building Connected Systems with GoLang
The Internet of Things (IoT) has transformed the way we interact with our environment, allowing everyday objects to communicate and exchange data seamlessly. From smart homes and wearable devices to industrial automation and smart cities, IoT is revolutionizing various industries. As the number of connected devices continues to grow, the need for robust and efficient programming languages also rises. This is where GoLang (also known as Golang) steps in as an ideal choice for building connected systems in the IoT domain.
In this blog, we will explore how GoLang can be a game-changer for IoT development, its key features, and how it simplifies the process of creating reliable and scalable connected systems. We will also delve into some practical examples and code snippets to demonstrate the power of Go in the IoT landscape.
1. Why GoLang for IoT?
Before diving into the specifics, let’s take a moment to understand why GoLang is gaining popularity for IoT development.
- Concurrent and Parallel Execution: One of the most significant advantages of GoLang is its built-in support for concurrency. IoT systems often involve handling multiple tasks simultaneously, such as reading sensor data, processing it, and controlling actuators. Go’s Goroutines and Channels enable concurrent execution, making it easy to manage various tasks concurrently, improving overall system performance.
- Efficiency and Performance: GoLang is a compiled language, which means it directly compiles into machine code, resulting in faster execution times and better performance. Its small memory footprint and efficient garbage collection make it well-suited for resource-constrained IoT devices.
- Simplicity and Readability: GoLang’s simple and elegant syntax promotes readability and ease of maintenance, which is crucial in IoT projects that might involve several developers working on the same codebase.
- Cross-Platform Support: GoLang is designed to be cross-platform, allowing developers to write code on one platform and easily deploy it on various devices and operating systems, a common requirement in the diverse IoT ecosystem.
- Rich Standard Library: GoLang comes with a comprehensive standard library, including packages for networking, encryption, and HTTP, which reduces the need for external dependencies, making the development process more streamlined.
2. Key Features of GoLang for IoT
- Goroutines and Channels: Goroutines are lightweight, independently executing functions that enable concurrent programming in Go. They provide an elegant way to handle multiple tasks concurrently. Channels, on the other hand, facilitate communication and data sharing between Goroutines, ensuring synchronized and safe operations.
- Concurrency with Goroutines Example:
go package main import ( "fmt" "time" ) func worker(id int, jobs <-chan string, results chan<- string) { for job := range jobs { // Simulate some work time.Sleep(time.Second) results <- fmt.Sprintf("Worker %d processed job: %s", id, job) } } func main() { numWorkers := 3 jobs := make(chan string, 5) results := make(chan string, 5) for i := 1; i <= numWorkers; i++ { go worker(i, jobs, results) } for i := 1; i <= 5; i++ { jobs <- fmt.Sprintf("Job %d", i) } close(jobs) for i := 1; i <= 5; i++ { fmt.Println(<-results) } }
In this example, we create three Goroutines to process jobs concurrently using channels for communication between the main program and the Goroutines.
- Lightweight Threading Model: GoLang utilizes a unique lightweight threading model, where Goroutines are managed by the Go runtime rather than the operating system. This allows for the creation of thousands of Goroutines without overwhelming system resources.
- Error Handling with Multiple Return Values: GoLang’s support for multiple return values simplifies error handling in IoT applications. It’s a common practice to return both the actual result and an error value from a function, making it easy to identify and handle errors effectively.
Error Handling Example:
go package main import ( "errors" "fmt" ) func divide(x, y float64) (float64, error) { if y == 0 { return 0, errors.New("cannot divide by zero") } return x / y, nil } func main() { result, err := divide(10, 2) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Result:", result) }
In this example, the divide function returns both the result of the division and an error if the divisor is zero.
- Memory Safety and Garbage Collection: GoLang’s memory safety features, such as array bounds checking, automatic garbage collection, and the lack of pointer arithmetic, prevent many common programming errors that could lead to memory corruption and security vulnerabilities.
- Rich Networking Libraries: GoLang provides a robust set of networking libraries, including TCP/IP, HTTP, and WebSocket, allowing developers to create IoT systems that can communicate efficiently with other devices and cloud services.
- JSON Support: IoT systems often rely on JSON as a data interchange format. GoLang provides excellent support for JSON encoding and decoding through its encoding/json package, making it easy to work with JSON data in IoT applications.
3. Go for IoT: Practical Examples
- Creating an MQTT Publisher: MQTT (Message Queue Telemetry Transport) is a widely used lightweight messaging protocol in IoT. Let’s see how easy it is to create an MQTT publisher using the “paho.mqtt.golang” library.
go package main import ( "fmt" "time" "github.com/eclipse/paho.mqtt.golang" ) func main() { opts := mqtt.NewClientOptions() opts.AddBroker("tcp://broker.example.com:1883") client := mqtt.NewClient(opts) if token := client.Connect(); token.Wait() && token.Error() != nil { fmt.Println("Error connecting to the broker:", token.Error()) return } topic := "iot/sensor/temperature" for i := 1; i <= 5; i++ { payload := fmt.Sprintf("Temperature reading %d", i) token := client.Publish(topic, 1, false, payload) token.Wait() time.Sleep(time.Second) } client.Disconnect(250) }
In this example, we publish simulated temperature readings to an MQTT broker using the “paho.mqtt.golang” library.
- Building a RESTful API for IoT Devices: RESTful APIs are commonly used to interact with IoT devices. Here’s how we can create a simple RESTful API using the “gin” web framework.
go package main import ( "fmt" "net/http" "github.com/gin-gonic/gin" ) type SensorData struct { ID int `json:"id"` Temperature string `json:"temperature"` Humidity string `json:"humidity"` } var sensors []SensorData func main() { router := gin.Default() router.GET("/sensors", getSensorData) router.POST("/sensors", addSensorData) router.Run(":8080") } func getSensorData(c *gin.Context) { c.JSON(http.StatusOK, sensors) } func addSensorData(c *gin.Context) { var newSensor SensorData if err := c.ShouldBindJSON(&newSensor); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } sensors = append(sensors, newSensor) c.Status(http.StatusCreated) }
In this example, we create a RESTful API using the “gin” framework to fetch and store sensor data.
Conclusion
GoLang’s inherent support for concurrency, efficiency, simplicity, and cross-platform capabilities makes it an excellent choice for building connected systems in the IoT landscape. Its robust standard library and growing ecosystem of third-party packages further enhance its capabilities. With practical examples and code snippets, we’ve seen how GoLang simplifies IoT development, from handling concurrent tasks to building RESTful APIs.
Whether you’re a seasoned developer or just starting in the IoT domain, Go for IoT provides a powerful and scalable foundation for your connected systems. As IoT continues to revolutionize the way we live and work, GoLang stands tall as a reliable and efficient language to build the next generation of IoT applications. So, embrace GoLang’s capabilities, and unlock the potential of your IoT projects like never before!
Table of Contents
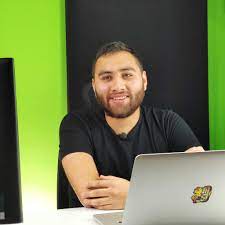
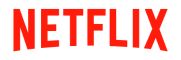