Go Q & A
How do you handle long-running tasks in Go?
Handling long-running tasks in Go requires careful consideration of concurrency, resource management, and error handling to ensure efficient and reliable execution. Go provides several mechanisms for managing long-running tasks, including Goroutines, channels, context, and timeouts.
- Goroutines: Goroutines are lightweight threads of execution in Go that enable concurrent processing of tasks without the overhead of traditional operating system threads. You can launch Goroutines using the go keyword, allowing multiple tasks to execute concurrently within the same Go program.
- Context and Timeouts: Use the context package to manage the lifecycle of long-running tasks and propagate cancellation signals across Goroutines. With the context.Context type and the context.WithTimeout function, you can set deadlines and timeouts for long-running operations, ensuring that tasks are canceled or terminated if they exceed a specified duration.
- Error Handling: Implement robust error handling mechanisms to handle errors and failures gracefully during long-running tasks. Use defer statements, error return values, and error channels to propagate errors, log diagnostic information, and perform cleanup operations when errors occur.
- Resource Management: Pay attention to resource management to prevent resource leaks and ensure efficient utilization of system resources. Close file handles, database connections, and network sockets when they are no longer needed to release system resources and avoid resource exhaustion.
- Concurrency Patterns: Apply concurrency patterns such as worker pools, fan-out/fan-in, and parallel processing to distribute and parallelize long-running tasks across multiple Goroutines. These patterns help maximize CPU utilization, reduce latency, and improve throughput for computationally intensive tasks.
- Graceful Shutdown: Implement graceful shutdown mechanisms to terminate long-running tasks safely and gracefully when the application is shut down or restarted. Use signals, context cancellation, and synchronization primitives to coordinate the shutdown process and ensure that active tasks complete their execution before exiting.
- Monitoring and Profiling: Monitor the performance and resource usage of long-running tasks using built-in profiling tools and monitoring solutions. Collect metrics, monitor memory consumption, and profile CPU usage to identify performance bottlenecks and optimize the execution of long-running tasks.
By following these best practices and leveraging Go’s concurrency model, you can effectively handle long-running tasks in Go applications, ensuring scalability, reliability, and responsiveness in high-concurrency environments.
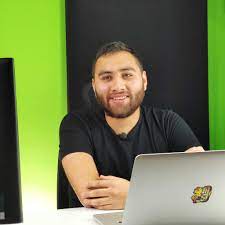
Previously at
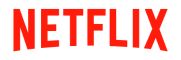
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.