Using Go for Machine Learning: Exploring Popular Libraries
Machine learning has become an integral part of various industries, enabling businesses to leverage data-driven insights for better decision-making. While Python has been the go-to language for many machine learning practitioners, other languages are also gaining popularity in this domain. One such language is Go, renowned for its simplicity, performance, and concurrency features. In this blog, we will delve into the world of machine learning with Go and explore some of the popular libraries that make it possible.
1. Why Go for Machine Learning?
When it comes to machine learning, Python has been the dominant language due to its extensive ecosystem and mature libraries. However, Go offers several advantages that make it an appealing choice for certain machine learning applications. Go’s simplicity and ease of use contribute to faster development cycles, and its static typing helps catch errors at compile-time rather than runtime.
Additionally, Go’s performance is noteworthy, making it suitable for computationally intensive tasks. Its built-in support for concurrency and parallelism allows for efficient utilization of modern hardware, enabling high-performance machine learning models. As a result, Go is particularly well-suited for real-time and low-latency applications.
2. Go Libraries for Machine Learning
While Go might not have as many machine learning libraries as Python, it does offer some excellent options that cater to various machine learning tasks. Let’s explore three popular libraries that can be leveraged for machine learning in Go.
2.1 Gonum:
Gonum is a powerful numerical library for Go, inspired by NumPy and MATLAB. It provides a wide range of mathematical functions and structures for linear algebra, optimization, and statistics. Gonum’s linear algebra package offers support for vectors, matrices, and operations like matrix multiplication and decomposition. This library serves as a solid foundation for implementing machine learning algorithms in Go.
2.2 Gorgonia:
Gorgonia is a deep learning library for Go, heavily inspired by TensorFlow. It allows developers to define, train, and deploy complex neural networks efficiently. Gorgonia’s API is designed to be familiar to users of other deep learning frameworks, making it easier to transition from Python to Go. With Gorgonia, you can build and train neural networks for various tasks, such as image classification and natural language processing.
2.3 Fathom:
Fathom is a library specifically designed for anomaly detection in time series data. It provides algorithms and functions for identifying abnormal patterns and outliers in datasets. Fathom supports both supervised and unsupervised anomaly detection techniques and can be used to detect anomalies in areas like cybersecurity, finance, and network monitoring. Its simplicity and ease of use make it an excellent choice for anomaly detection tasks in Go.
3. Getting Started with Gonum:
To demonstrate the capabilities of Gonum, let’s dive into a simple linear regression example. Follow the steps below to get started:
3.1 Installation:
Install Gonum using the following command:
shell go get -u gonum.org/v1/gonum/…
3.2 Linear Regression with Gonum:
Here’s an example code snippet that performs linear regression using Gonum:
go package main import ( "fmt" "gonum.org/v1/gonum/floats" "gonum.org/v1/gonum/mat" ) func main() { // Create the input matrix X and output vector Y X := mat.NewDense(4, 2, []float64{ 0, 0, 1, 0, 0, 1, 1, 1, }) Y := mat.NewVecDense(4, []float64{0, 1, 1, 1}) // Compute the least squares solution var solution mat.VecDense err := solution.SolveVec(X, Y) if err != nil { fmt.Println("Error:", err) return } // Print the coefficients coefficients := solution.RawVector().Data fmt.Println("Coefficients:", coefficients) }
This code creates an input matrix X and an output vector Y for a simple linear regression problem. It then uses the SolveVec function from Gonum to compute the least squares solution. Finally, the coefficients are printed.
4. Exploring Gorgonia for Neural Networks:
Gorgonia provides a flexible and intuitive API for building and training neural networks in Go. Let’s see how to get started with Gorgonia using a simple example.
4.1 Installation:
Install Gorgonia using the following command:
shell go get -u gorgonia.org/gorgonia
4.2 Building a Simple Neural Network:
Here’s an example code snippet that demonstrates building a simple neural network using Gorgonia:
go package main import ( "fmt" "gorgonia.org/gorgonia" "gorgonia.org/tensor" ) func main() { // Define the neural network architecture g := gorgonia.NewGraph() x := gorgonia.NewTensor(g, tensor.Float32, 2, gorgonia.WithShape(2, 1), gorgonia.WithName("x")) w := gorgonia.NewMatrix(g, tensor.Float32, gorgonia.WithShape(1, 2), gorgonia.WithName("w")) b := gorgonia.NewMatrix(g, tensor.Float32, gorgonia.WithShape(1, 1), gorgonia.WithName("b")) dot := gorgonia.Must(gorgonia.Mul(w, x)) output := gorgonia.Must(gorgonia.Add(dot, b)) // Compile and run the computation graph machine := gorgonia.NewTapeMachine(g, gorgonia.BindDualValues(w, b)) if err := machine.RunAll(); err != nil { fmt.Println("Error:", err) return } // Fetch and print the output result := output.Value().Data().([]float32) fmt.Println("Output:", result) }
In this code, we define a simple neural network with one input layer, one weight matrix (w), and one bias matrix (b). We then perform matrix multiplication and addition operations using Gorgonia’s tensor operations. Finally, we compile and run the computation graph using a TapeMachine and fetch the output.
5. Anomaly Detection with Fathom:
Fathom simplifies the process of detecting anomalies in time series data. Let’s see how to use Fathom for unsupervised anomaly detection.
5.1 Installation:
Install Fathom using the following command:
shell go get -u github.com/analytics-go/fathom
5.2 Unsupervised Anomaly Detection:
Here’s an example code snippet that demonstrates unsupervised anomaly detection using Fathom:
go package main import ( "fmt" "github.com/analytics-go/fathom" ) func main() { // Create a time series dataset dataset := []float64{1.0, 2.0, 1.5, 1.2, 1.3, 10.0, 1.1, 1.2, 1.0, 1.4, 1.5} // Create a Fathom model and fit the data model := fathom.NewModel() model.Fit(dataset) // Detect anomalies in the data anomalies := model.Detect(dataset) // Print the detected anomalies fmt.Println("Anomalies:", anomalies) }
This code creates a time series dataset and uses Fathom to detect anomalies within it. The Fit function is used to train the model on the dataset, and the Detect function is used to identify anomalies. The detected anomalies are then printed.
Conclusion:
In this blog, we explored the possibilities of using Go for machine learning tasks. We discussed the advantages of Go, such as its simplicity, performance, and concurrency features. We also explored three popular libraries for machine learning in Go: Gonum for numerical computations, Gorgonia for deep learning, and Fathom for anomaly detection. With these libraries, Go developers can now leverage the power and efficiency of the Go language for various machine learning applications.
Table of Contents
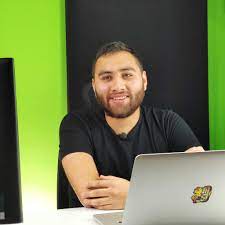
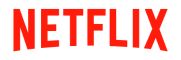